How do I break out of nested loops in Java?
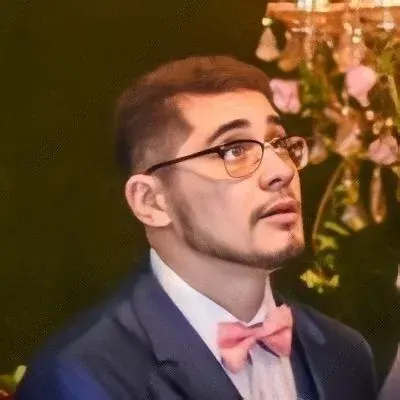
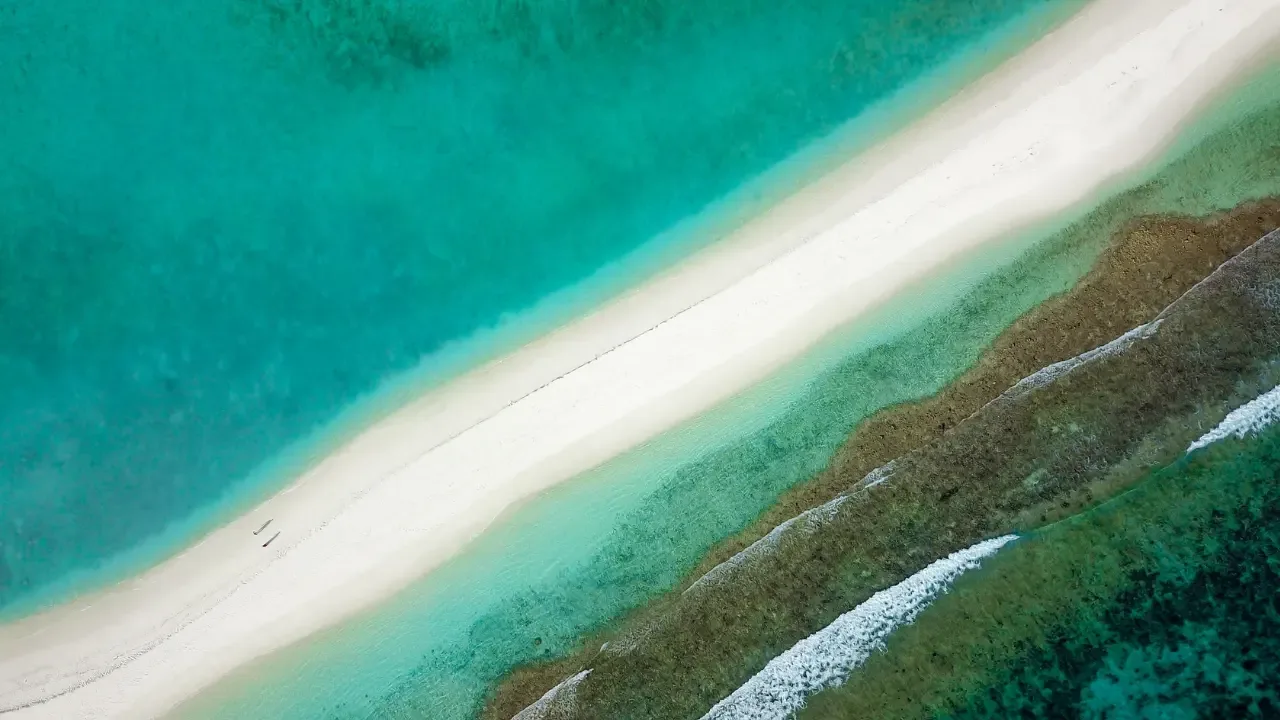
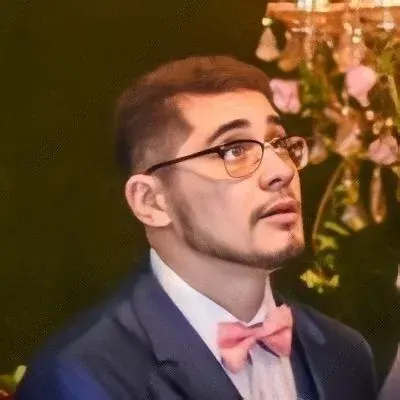
🔀 Breaking Out of Nested Loops in Java: An Easy Guide 🔀
Are you tired of being trapped in the unending maze of nested loops in Java? 😩 Don't worry, help is here! 🎉 In this blog post, we will dive into the depths of nested loops, explore common issues faced when trying to break out, and provide you with easy solutions to escape the looping madness. Let's get started! 💪
📌 **The Nested Loop Scenario ** 📌
Imagine you have a nested loop construct similar to the code snippet below:
for (Type type : types) {
for (Type t : types2) {
if (some condition) {
// Do something and break...
break; // Breaks out of the inner loop
}
}
}
In this example, we have two loops - an outer loop iterating through types
and an inner loop iterating through types2
. When a certain condition is met inside the inner loop, we want to break out of both loops and continue with the execution of the loop block.
👉 Issue 1: Breaking Only the Inner Loop
The code snippet provided already solves the problem of breaking out of the inner loop. By utilizing the break
statement within the inner loop, we achieve the desired outcome. This statement breaks out of the inner loop and continues with the next iteration of the outer loop.
✨ Solution ✨
To break out of both loops, we need to introduce a label and utilize it with the break
statement. Labels in Java act as markers to identify specific areas within nested constructs.
To break out of both loops, modify the code as shown below:
outerLoop:
for (Type type : types) {
for (Type t : types2) {
if (some condition) {
// Do something and break both loops...
break outerLoop; // Breaks out of the outer loop
}
}
}
In the example above, we added the outerLoop
label before the outer loop declaration. The break
statement is then modified to break outerLoop
, ensuring that both loops are broken and the execution continues after the outer loop.
👉 Issue 2: Avoiding the Use of Gotos
Perhaps you came across solutions suggesting the use of gotos
to break out of nested loops. However, you may have found them unappealing or even frowned upon by some programmers.
Don't worry, the solution we presented above completely avoids the use of gotos
. We introduced the concept of labels to provide a clean, readable, and efficient alternative.
👉 Issue 3: Not Wanting to Separate into a Different Method
Separating the inner loop into a different method might seem like a feasible solution. However, if you prefer to keep the loop construct intact and avoid additional method calls, the solution with labels suits your requirements perfectly.
🎯 Call-to-Action: Engage and Share Your Experience! 🎯
We hope this guide helped you break out of nested loops in Java with ease! 😃 Now it's your turn to put it into action and solve those nested loop dilemmas. Share your experience with us and let us know if you found this guide helpful. We love hearing from our readers! 🙌
Feel free to leave a comment below, share this post with your fellow Java enthusiasts ✨, and subscribe to our newsletter for more insightful guides and tutorials. Happy coding! 💻🔥