How do I avoid checking for nulls in Java?
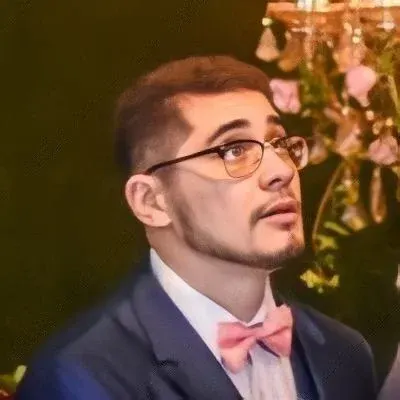
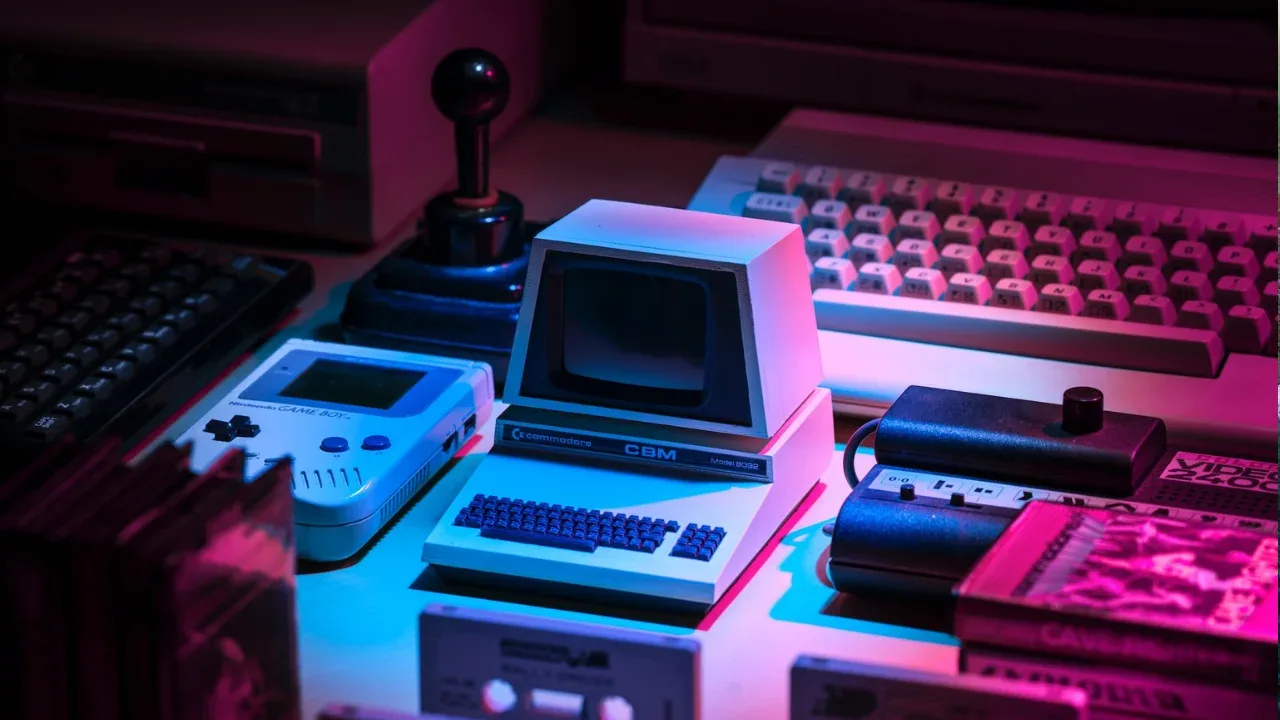
How to ๐ซ Avoid Checking for Nulls in Java?
Are you tired of constantly checking for null values in your Java code? Do you find it tedious and error-prone? Well, fret no more! In this blog post, we will explore some alternative approaches to avoid those pesky NullPointerExceptions.
โ ๏ธ Danger Zone: NullPointerException โ ๏ธ
Before we dive into the solutions, let's understand why NullPointerExceptions occur. In Java, when you attempt to perform an operation on an object that is not initialized (i.e., null), the JVM will throw a NullPointerException. This can happen when you forget to assign a value to a variable or when a method returns null.
๐ซ The Traditional Way: Checking for Nulls
The most common approach to avoiding NullPointerExceptions is by checking if a variable is not null before accessing its properties or invoking its methods. For example:
if (x != null) {
x.doSomething(); // Safely accessing x only if it's not null
}
๐ The Optional Class
Java 8 introduced the Optional
class, which provides a more elegant and concise way to handle null values. With Optional
, you can gracefully handle the absence of a value without the need for explicit null checks.
Optional<X> optionalX = Optional.ofNullable(x);
if (optionalX.isPresent()) {
optionalX.get().doSomething(); // Safely accessing x only if it's not null
}
๐ก The Elvis Operator: Avoiding the If-Else Dance
In Java, you might have encountered the infamous if-else dance when assigning a default value to a variable if it's null. The Elvis operator (?:
) can help you simplify this code and make it more readable.
X fallbackX = new X(); // A default value to fall back to if x is null
X actualX = x != null ? x : fallbackX;
๐ ๏ธ The Null Object Pattern
If checking for nulls is a recurring theme in your codebase, you might consider applying the Null Object Pattern. This pattern allows you to create a special null object that implements the same interface as the intended object but provides no-op or default behavior.
public interface X {
void doSomething();
}
public class NullX implements X {
@Override
public void doSomething() {
// No-op behavior for the null object
}
}
// Usage:
X myX = x != null ? x : new NullX();
myX.doSomething(); // Safely invoking doSomething() without explicit null check
โจ The Optional Chaining (Java 8+)
Java 8 introduced a handy syntax called Optional chaining, which allows you to eliminate explicit null checks when accessing properties or invoking methods on nested objects.
Optional.ofNullable(x)
.ifPresent(value -> value.doSomething()); // Safely invoking doSomething() if x is not null
๐งช Unit Testing - Friend or Foe?
Unit testing your code is a critical part of ensuring its correctness and identifying potential issues. Writing robust unit tests that cover both null and non-null scenarios can help reveal any null-related bugs before they reach production.
๐ The Call-to-Action: Share Your Experiences!
Have you encountered NullPointerExceptions in your Java code, and how did you handle them? Share your experiences, tips, and tricks in the comments section below! Let's learn from each other and write null-safe code together! ๐
๐ Remember to subscribe to our newsletter and follow us on Twitter for more Java-related content. Happy coding! ๐ฅ๏ธโจ
Note: We acknowledge that avoiding nulls is not always possible or practical in every scenario. However, by leveraging some of the techniques mentioned above, you can reduce NullPointerException occurrences and improve the overall readability and safety of your code.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
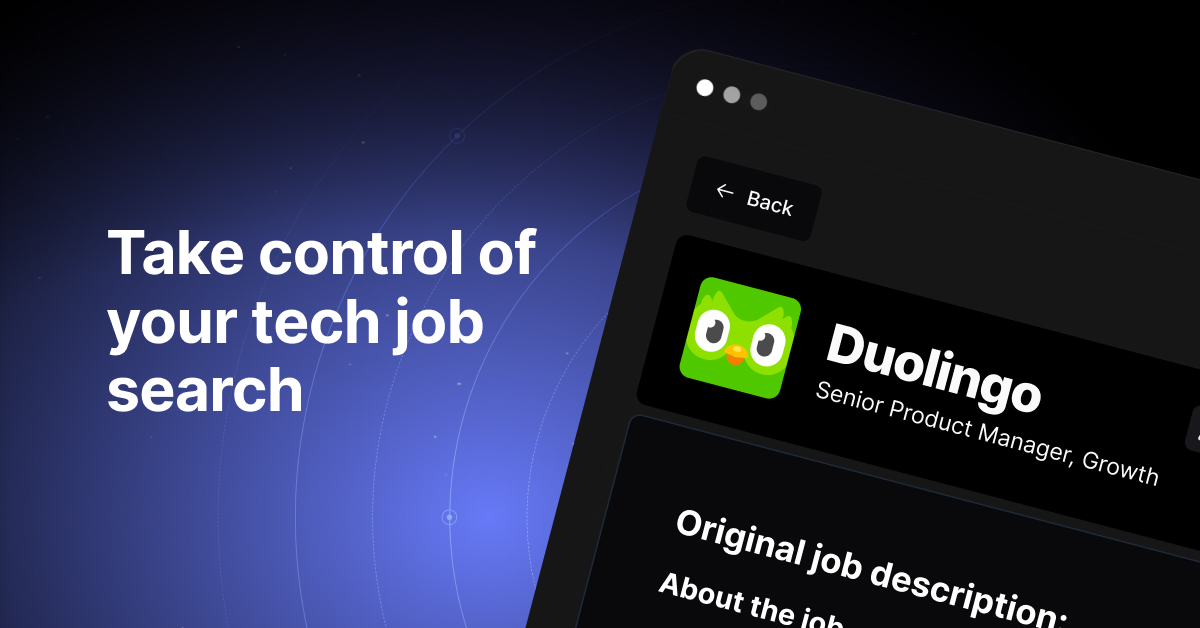