How can I read a large text file line by line using Java?
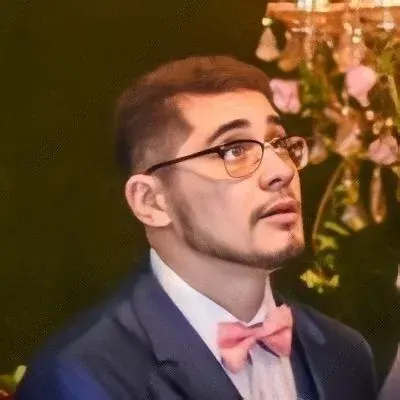
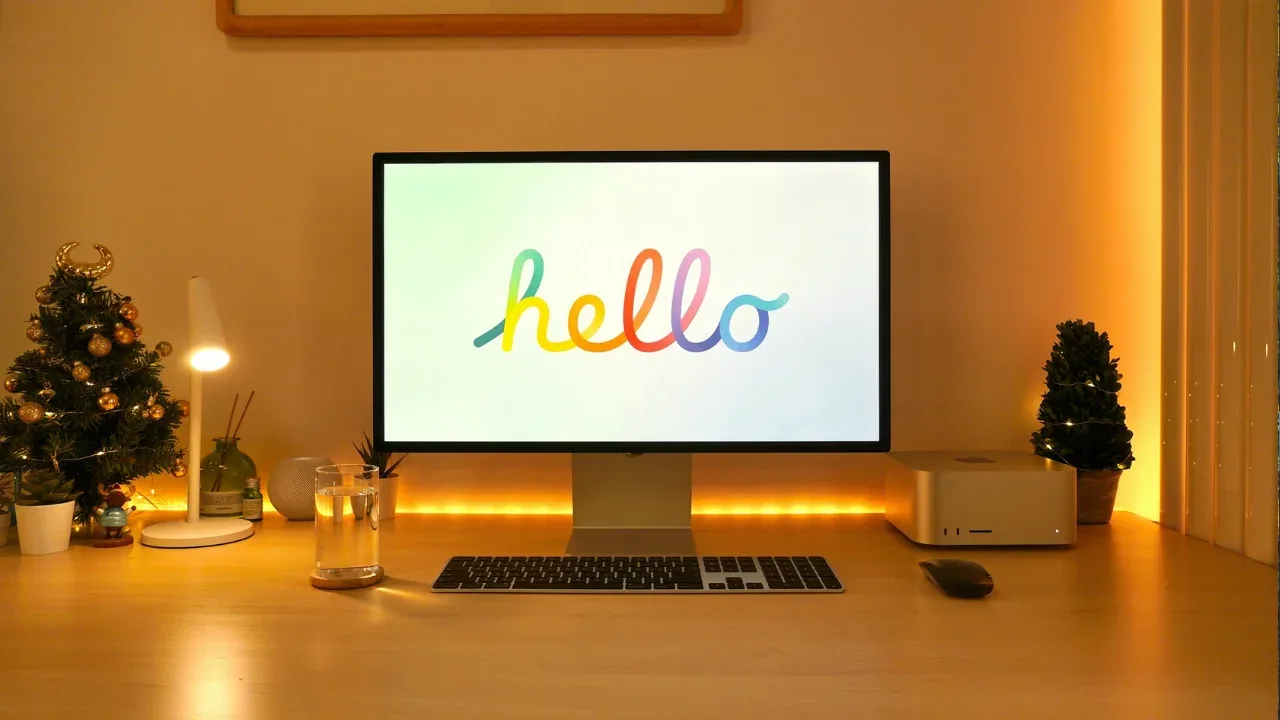
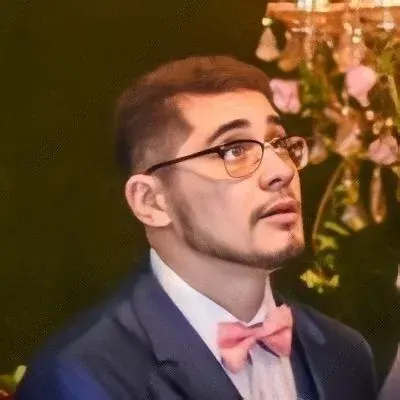
📝 Hey there tech enthusiasts! Have you ever found yourself in a situation where you needed to read a massive text file line by line using Java? 📃 Guess what, you're not alone! Many developers face this dilemma, especially when dealing with large datasets or log files. But fear not, because I'm here to guide you through this challenge and provide you with easy and efficient solutions. Let's dive right in! 💪
🔍 The Problem: So, you have a gargantuan text file, say around 5-6 GB in size, and you're wondering how to read it line by line without breaking a sweat. Reading the entire file into memory can be time-consuming and resource-intensive. We need a faster and more efficient way to accomplish this task.
💡 Solution Approach:
To overcome this challenge, we can leverage Java's BufferedReader
class and its readLine()
method. This class allows us to read data from a file in chunks, reducing memory usage and improving performance. Here's how you can do it:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class ReadFileLineByLine {
public static void main(String[] args) {
String filePath = "path/to/your/file.txt";
try (BufferedReader br = new BufferedReader(new FileReader(filePath))) {
String line;
while ((line = br.readLine()) != null) {
// Process each line here
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
🚀 Explanation:
Let's break down the code. First, we create a BufferedReader
object by passing a FileReader
object that reads from the specified file path. The BufferedReader
class reads the file in chunks or buffers, rather than reading character by character or byte by byte, making it faster.
Next, we enter a while
loop, which continues until the readLine()
method returns null
, indicating the end of the file. Within the loop, we process each line as required. In this example, we simply print each line using System.out.println()
. You can replace this with your desired logic to handle each line.
📢 Call-to-Action: Now that you know how to read large text files line by line using Java, go ahead and give it a try! If you have any questions or encountered any issues, feel free to share them in the comments below. Don't forget to smash that like button and share this post with your fellow devs who might find it helpful. Happy coding! 😄👍