How can I inject a property value into a Spring Bean which was configured using annotations?
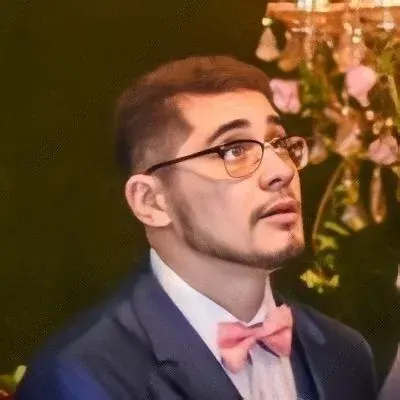
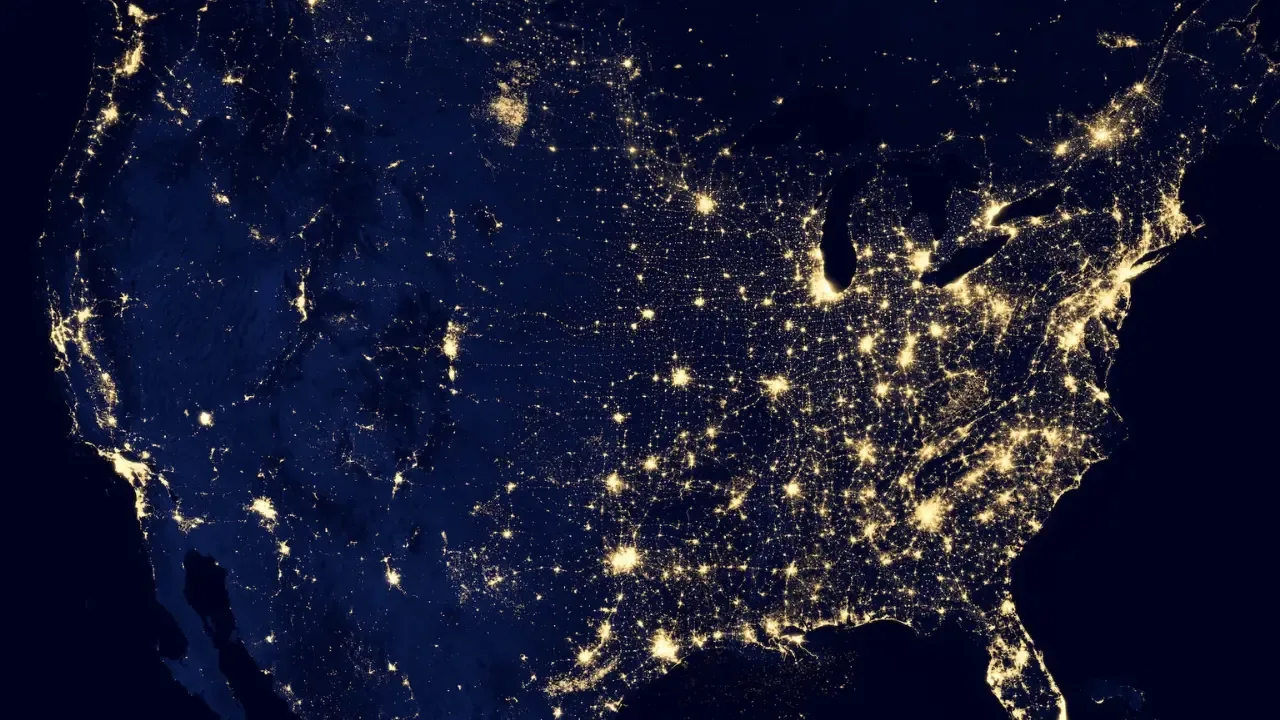
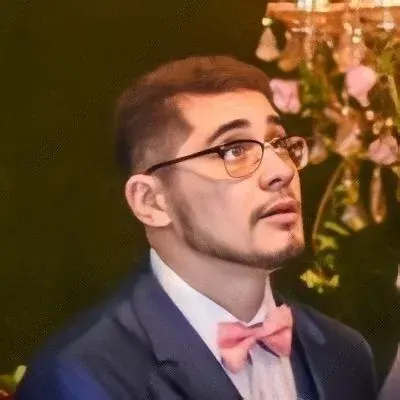
How to Inject a Property Value into a Spring Bean Configured with Annotations
š± So, you have a bunch of Spring beans that are picked up from the classpath using annotations, but you want to inject a property value into one of them. š±
š First, let's understand the problem. The bean you want to inject the property into is not mentioned in the Spring XML file, as it is configured via annotations. This means that you can't simply define the property value in the XML file as you would normally do. š
The Solution: @Value Annotation
Enter the š @Value
annotation. By using this annotation, you can inject property values directly into your Spring beans, even if they are configured with annotations.
Here's how you can do it:
First, make sure you have a
PropertyPlaceholderConfigurer
defined in your Spring XML file. This configurer will resolve the property values from a properties file. For example:
<bean id="propertyConfigurer" class="org.springframework.beans.factory.config.PropertyPlaceholderConfigurer">
<property name="location" value="/WEB-INF/app.properties" />
</bean>
In your Spring bean class (in this case,
PersonDaoImpl
), use the@Value
annotation to inject the property value wherever you need it. For example:
@Repository("personDao")
public class PersonDaoImpl extends AbstractDaoImpl implements PersonDao {
@Value("${results.max}")
private int maxResults;
// Rest of the class implementation
}
In the above code, the value of ${results.max}
will be resolved by the PropertyPlaceholderConfigurer
and injected into the maxResults
variable.
And that's it! š You have successfully injected a property value into a Spring bean configured with annotations!
Accessing the Property Value Programmatically
But what if you need to access the property value programmatically, instead of injecting it into a field?
You can still achieve that by injecting the Environment
object provided by Spring and using its getProperty
method. Here's an example:
@Repository("personDao")
public class PersonDaoImpl extends AbstractDaoImpl implements PersonDao {
@Autowired
private Environment environment;
// Method to access the property value
public void someMethod() {
String maxResults = environment.getProperty("results.max");
// Use the property value
}
// Rest of the class implementation
}
In the above code, we injected the Environment
object using the @Autowired
annotation and then used its getProperty
method to fetch the value of the desired property.
Time to Inject Properties Like a Pro! šŖ
Now that you know how to inject property values into Spring beans configured with annotations, go ahead and give it a try! Get those properties flowing into your beans like magic. āØ
And hey, we would love to hear about your experience! Share your thoughts, questions, or any cool things you have done with property injection in the comments below. Let's geek out together! š¤š¬
That's it for now! Stay tuned, subscribe to our newsletter, and keep up with the latest tech tips, guides, and cool stuff. Until next time! šš