How can I initialise a static Map?
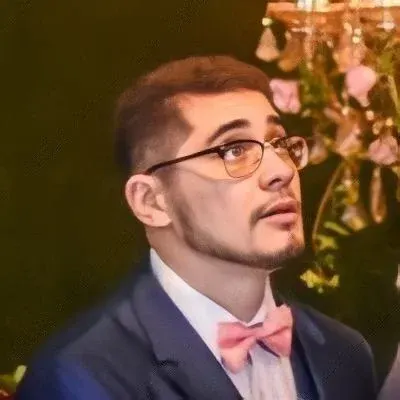
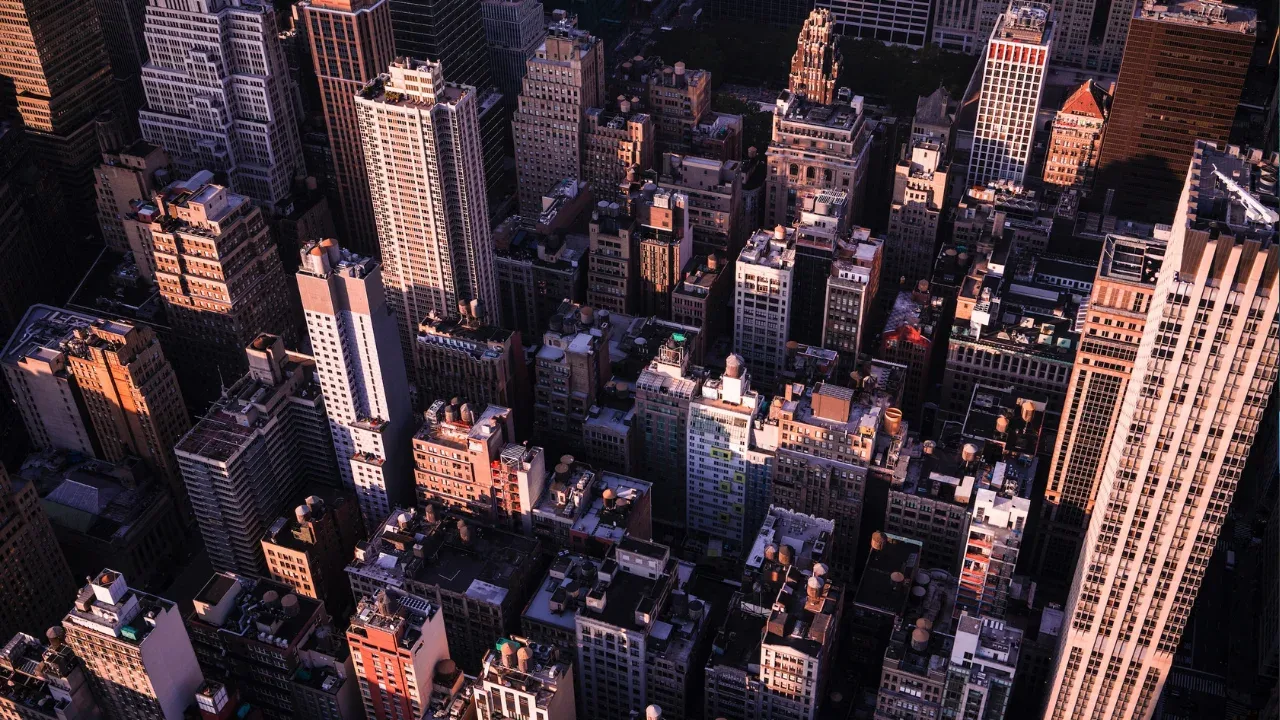
📝🔍📢 How to Initialise a Static Map in Java: Explained with Examples!
Are you struggling with how to initialise a static Map in Java? 🤔 Don't worry! In this blog post, we will explore different methods to solve this problem and provide you with easy solutions. So, let's dive right in and find out which approach suits your needs the best! 💪
📌 Method One: Static Initialiser One way to initialise a static Map is by using a static initialiser block. In this method, you declare your static Map and then add key-value pairs to it within the static block. This ensures that the Map is initialised when the class is loaded. Take a look at the example below:
import java.util.HashMap;
import java.util.Map;
public class Test {
private static final Map<Integer, String> myMap = new HashMap<>();
static {
myMap.put(1, "one");
myMap.put(2, "two");
}
}
✅ Pros of the Static Initialiser Method:
Simple and straightforward
Allows you to initialise the Map with custom values
Ensures the Map is always ready for use
🚫 Cons of the Static Initialiser Method:
Requires an additional static block in your code
The Map cannot be modified after initialisation
📌 Method Two: Instance Initialiser (Anonymous Subclass) Another approach is to use an instance initialiser, also known as an anonymous subclass. In this method, you create a new HashMap subclass and initialise it with the desired values. Here's how you can do it:
import java.util.HashMap;
import java.util.Map;
public class Test {
private static final Map<Integer, String> myMap2 = new HashMap<>(){
{
put(1, "one");
put(2, "two");
}
};
}
✅ Pros of the Instance Initialiser Method:
Allows you to initialise the Map with custom values
No need for an extra static block
🚫 Cons of the Instance Initialiser Method:
Slightly more complex syntax with anonymous subclass creation
Now that you know the two methods, it's time to choose the one that fits your requirements! 😎
🔗💬 But wait, before you go, we'd love to hear from you! Which method do you prefer for initialising a static Map in Java? Let us know in the comments below! Share your experiences and insights to help other developers make an informed decision. 🙌
Remember, there's no one-size-fits-all solution, so share your thoughts and let's start a discussion! Happy coding! 🚀👩💻👨💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
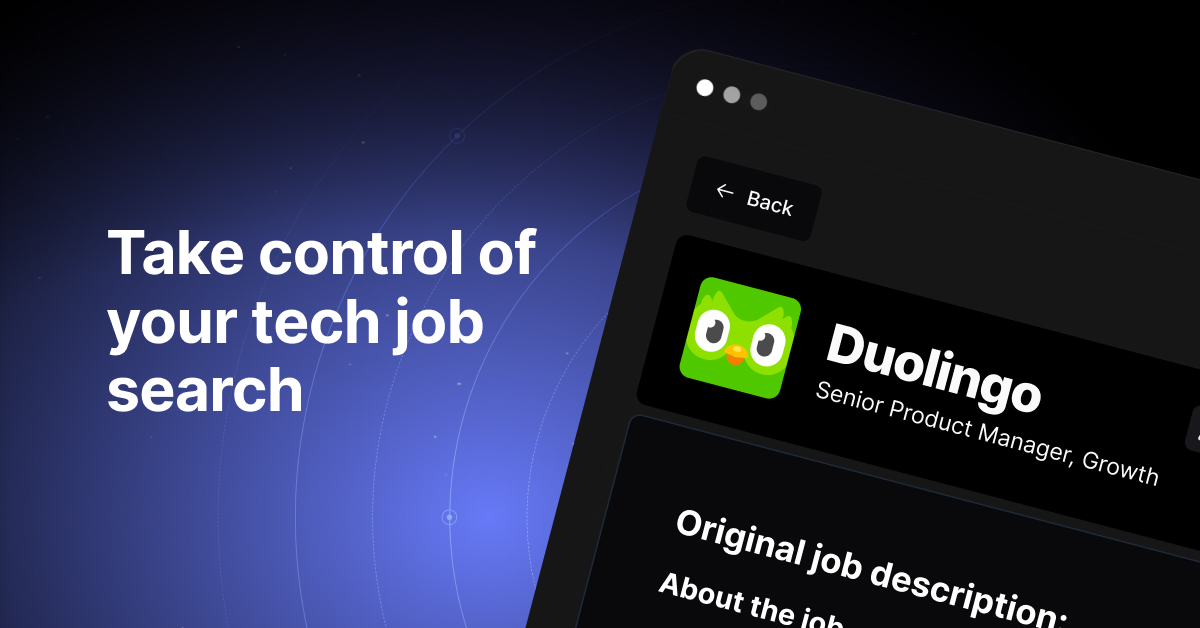