How can I fix "android.os.NetworkOnMainThreadException"?
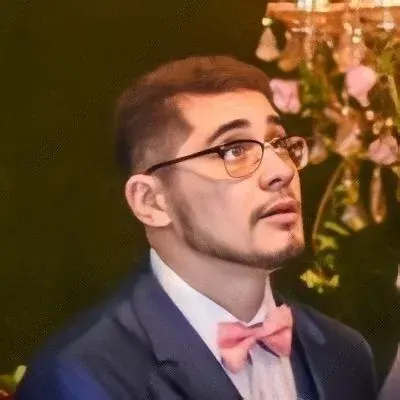
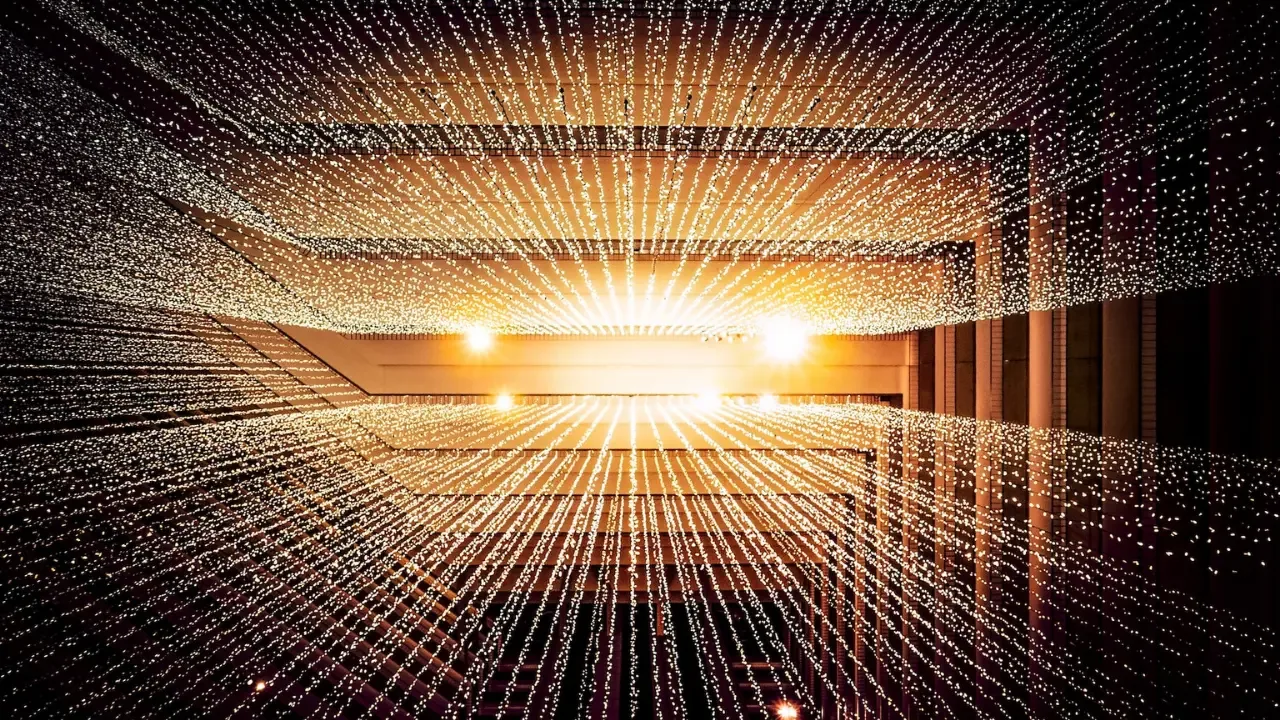
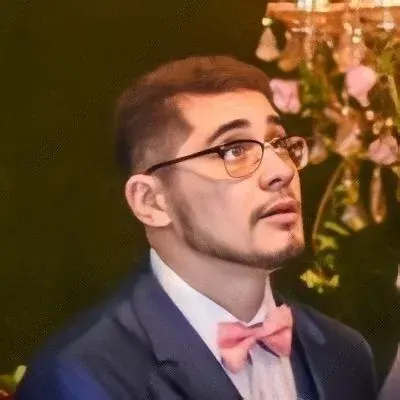
How to Fix 'android.os.NetworkOnMainThreadException' 📱💥
Are you an Android developer encountering the dreaded android.os.NetworkOnMainThreadException
error? 😱 Don't worry, you're not alone! This error occurs when you attempt to perform network operations on the main thread of your Android application. In this blog post, we'll explain why this error occurs, provide easy-to-follow solutions, and give you a compelling call-to-action at the end. Let's get started! 🚀
Understanding the Error 🧐
To understand the android.os.NetworkOnMainThreadException
error, you need to know that Android applications are single-threaded by default. The main thread, also known as the UI thread, is responsible for handling user interactions and updating the user interface. Performing long-running operations, such as network requests, on the main thread can lead to unresponsive and sluggish apps. To prevent this, Android enforces the StrictMode policy, which throws a NetworkOnMainThreadException
when network operations are detected on the main thread. 🕷️🚫
Common Issues 🚩
The error often occurs when developers make HTTP requests or perform other network-related tasks directly on the main thread. In your code snippet, it's evident that you are attempting to open a network connection on the main thread, triggering the exception. 😬
URL url = new URL(urlToRssFeed);
SAXParserFactory factory = SAXParserFactory.newInstance();
SAXParser parser = factory.newSAXParser();
XMLReader xmlreader = parser.getXMLReader();
RssHandler theRSSHandler = new RssHandler();
xmlreader.setContentHandler(theRSSHandler);
InputSource is = new InputSource(url.openStream());
xmlreader.parse(is);
return theRSSHandler.getFeed();
Easy Solutions ✅
To fix the android.os.NetworkOnMainThreadException
error, you need to move your network operations off the main thread. Here are a couple of simple solutions:
Solution 1: Use Asynchronous Tasks (AsyncTask) 🔄
One popular approach is to utilize the AsyncTask
class provided by Android. This class allows you to perform background tasks and update the UI thread when the task is complete. To rewrite your code using AsyncTask
, follow these steps:
Create a new class that extends
AsyncTask<Void, Void, YourResultType>
, whereVoid
represents input parameters,Void
indicates the progress value, andYourResultType
represents the result of your network operation.Override the
doInBackground()
method and move your network code inside it.Update the UI using the
onPostExecute()
method with the result obtained from the background task.
Here's an example of how you can modify your code snippet using AsyncTask
:
private class NetworkTask extends AsyncTask<Void, Void, YourResultType> {
@Override
protected YourResultType doInBackground(Void... params) {
URL url = new URL(urlToRssFeed);
SAXParserFactory factory = SAXParserFactory.newInstance();
SAXParser parser = factory.newSAXParser();
XMLReader xmlreader = parser.getXMLReader();
RssHandler theRSSHandler = new RssHandler();
xmlreader.setContentHandler(theRSSHandler);
InputSource is = new InputSource(url.openStream());
xmlreader.parse(is);
return theRSSHandler.getFeed();
}
@Override
protected void onPostExecute(YourResultType result) {
// Update UI with the result
}
}
// Execute the network task
new NetworkTask().execute();
Solution 2: Use Kotlin Coroutines 🌪️
If you're using Kotlin, another modern approach is to utilize Kotlin Coroutines. Coroutines provide a simple and elegant way to write asynchronous code that is both concise and efficient. To perform network operations using coroutines, follow these steps:
Declare your network operation as a
suspend
function.Use the
lifecycleScope.launch(Dispatchers.IO)
coroutine builder to execute the network operation on a background thread.Update the UI on the main thread using the
lifecycleScope.launch(Dispatchers.Main)
coroutine builder.
Here's an example of how you can modify your code snippet using Kotlin coroutines:
lifecycleScope.launch(Dispatchers.IO) {
val url = URL(urlToRssFeed)
val factory = SAXParserFactory.newInstance()
val parser = factory.newSAXParser()
val xmlreader = parser.xmlReader
val theRSSHandler = RssHandler()
xmlreader.contentHandler = theRSSHandler
val is = InputSource(url.openStream())
xmlreader.parse(is)
val result = theRSSHandler.feed
lifecycleScope.launch(Dispatchers.Main) {
// Update UI with the result
}
}
There you have it! Two easy solutions to fix the android.os.NetworkOnMainThreadException
error. Choose the approach that suits your needs and bring your application's performance back to life! 💪
Wrap Up and Your Next Steps 🎉📲
Don't let the android.os.NetworkOnMainThreadException
error hinder your Android development journey. Follow the solutions provided and start building smooth and responsive apps today! 🚀
If you found this article helpful, share it with your fellow developers and spread the knowledge. Let's foster a community of Android developers who tackle challenges head-on! 🤝💡
Now, go forth and conquer the android.os.NetworkOnMainThreadException
error! If you have any questions or want to share your success story, drop a comment below. Happy coding! 😄🔥