How can I create an executable/runnable JAR with dependencies using Maven?
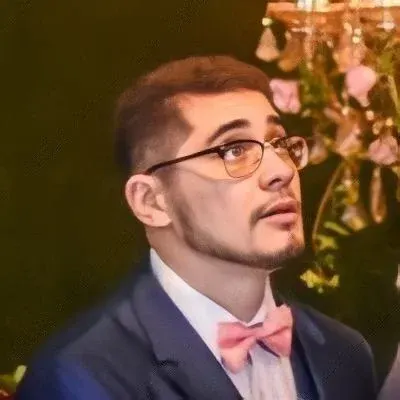
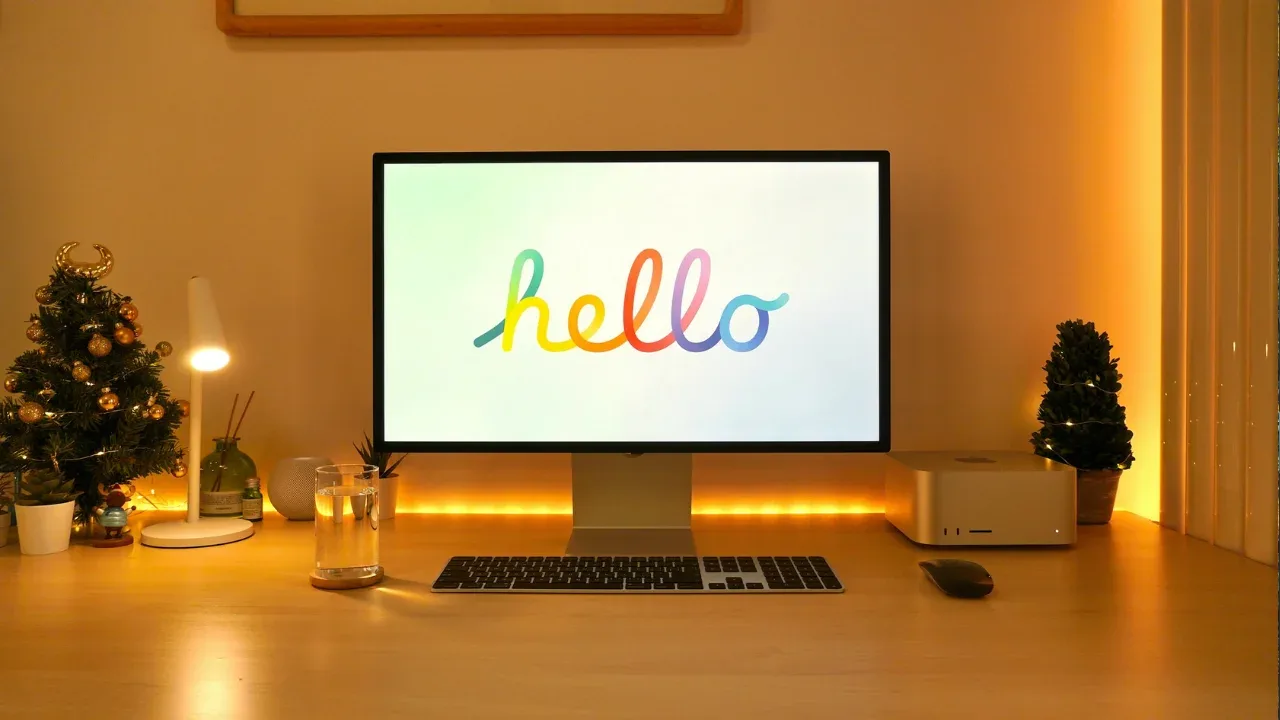
🚀 Creating an Executable JAR with Dependencies using Maven: A Complete Guide 📦
So, you've built your awesome Java project, and now you want to package it into a single, self-contained JAR for easy distribution. But there's one problem – your project has several dependencies! 😱
No need to panic! Maven, the powerful build tool, comes to the rescue. In this guide, we'll show you how to create an executable JAR with all its dependencies using Maven, so your users can run your project without any hassle. Let's get started! 💪
1. Start with a Maven project 🏗️
Before we dive into the juicy details, make sure you have a Maven project set up. If you don't, fear not! Simply head over to the Maven website, download and install Maven, and use it to create a new project.
2. Configure Maven's assembly plugin 🛠️
To create a fully executable JAR, we need to configure Maven's assembly plugin. Open your project's pom.xml
file and ensure that the following plugin is included within the <build>
section:
<plugins>
...
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-assembly-plugin</artifactId>
<version>3.3.0</version>
<configuration>
<descriptorRefs>
<descriptorRef>jar-with-dependencies</descriptorRef>
</descriptorRefs>
<archive>
<manifest>
<mainClass>com.yourproject.YourMainClass</mainClass>
</manifest>
</archive>
</configuration>
<executions>
<execution>
<id>make-assembly</id>
<phase>package</phase>
<goals>
<goal>single</goal>
</goals>
</execution>
</executions>
</plugin>
...
</plugins>
Make sure to replace com.yourproject.YourMainClass
with the actual fully qualified name of your project's main class.
3. Build your executable JAR 🏭
Now that you have Maven set up and the assembly plugin configured, it's time to build your executable JAR. Open a terminal or command prompt, navigate to your project's root directory, and run the following command:
mvn clean package
Maven will start building your project and packaging all its dependencies into the JAR. Once the process is complete, you will find your shiny new executable JAR in the target
directory of your project.
4. Test your JAR 🔍
Testing is vital before sharing your JAR with the world. To ensure everything is working as expected, run your executable JAR using the following command:
java -jar target/your-jar-filename.jar
Replace your-jar-filename.jar
with the actual filename of your JAR. If your project has a graphical user interface (GUI), check that it launches correctly and all its functionalities work seamlessly.
5. Share and distribute your JAR 🌍
Congratulations, you've successfully created an executable JAR with all its dependencies! Now it's time to share your masterpiece with the world. There are various ways to distribute your JAR:
Upload it to a file-sharing platform like Dropbox or Google Drive and share the download link.
Create a GitHub repository and upload your project, making the JAR available for download.
Publish your project on a package registry like Maven Central, so others can easily include it as a dependency in their projects.
📣 Engage with the Community!
Did you find this guide helpful? Have you encountered any issues or discovered additional tips along the way? We'd love to hear from you!
Head over to our blog and leave a comment, share your experience, or ask any questions you may have. Together, let's continue learning and growing as a community of tech enthusiasts! 🌐💬🚀
That concludes our guide on creating an executable JAR with dependencies using Maven. We hope you found it informative and easy to follow. Now go ahead and package your project into a self-contained JAR, and let it shine for the world to see! 🌟✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
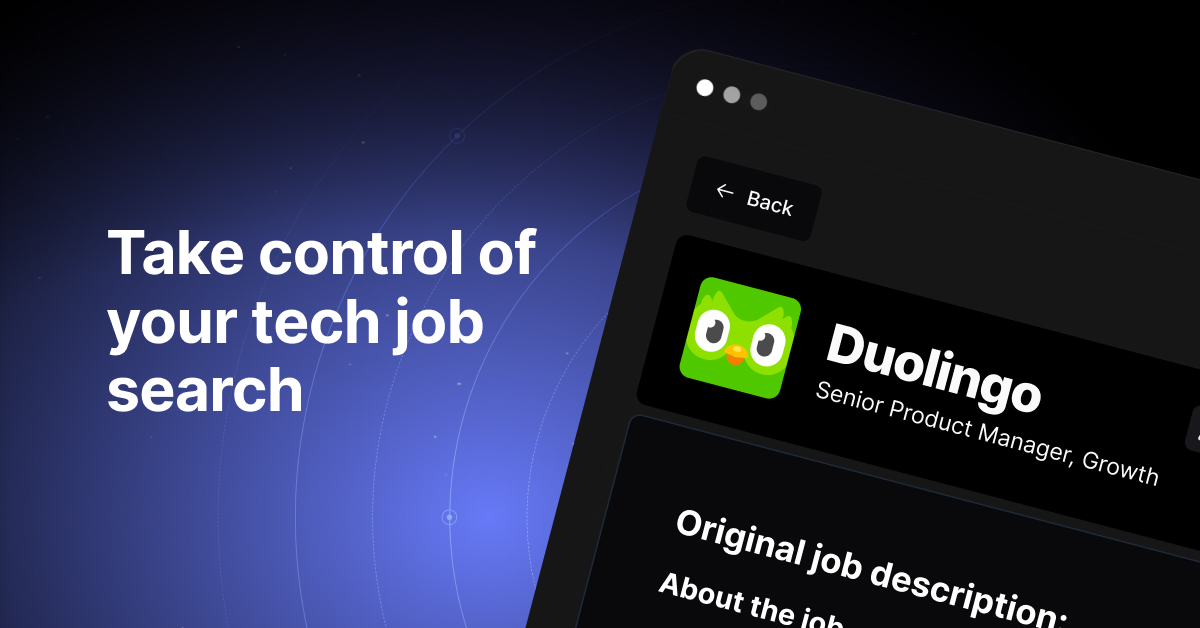