How can I convert a stack trace to a string?
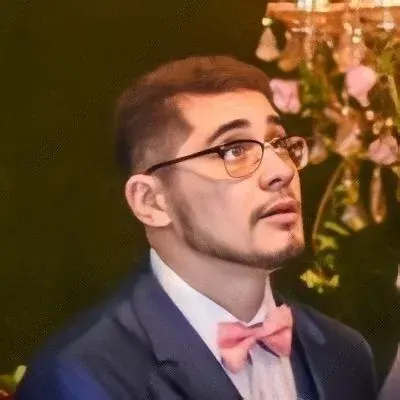
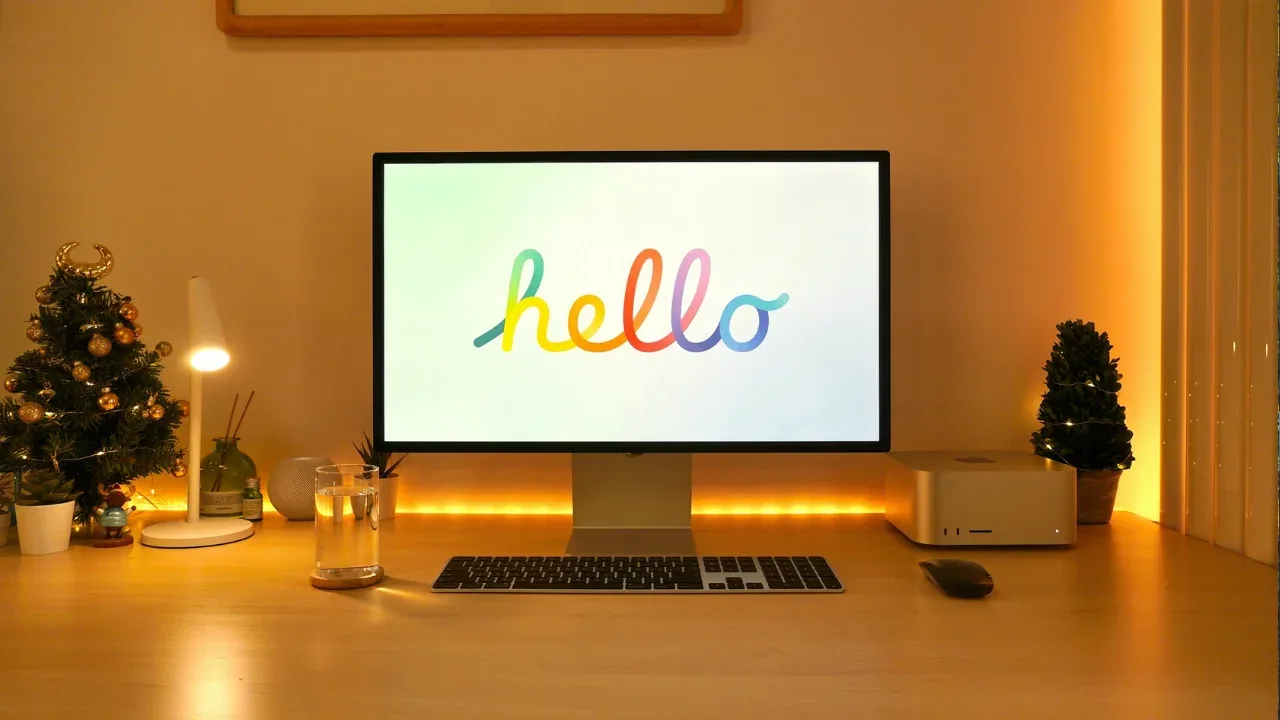
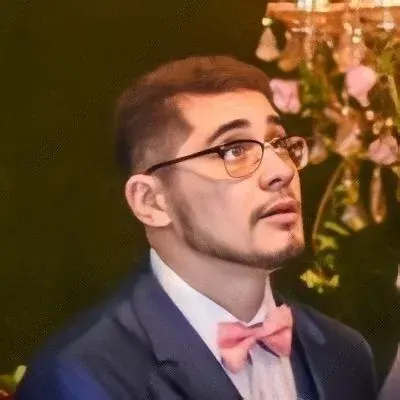
📝💻🕸️ Converting a Stack Trace to a String: A Guide for Tech Enthusiasts 🚀🔎🔍
Are you a developer trying to make sense of a perplexing stack trace? 😫 Do you ever find yourself scratching your head in confusion as you attempt to convert the uncooperative stack trace into a more readable format? 🧐 Well, worry no more! In this blog post, we'll tackle the common issue of converting a stack trace to a string, and we'll provide you with easy solutions that will save you time and frustration. Let's dive in! 💪🌊
Understanding the Problem 🤔❓
When an unexpected error occurs in your code, the JVM (Java Virtual Machine) captures a snapshot of the execution stack, commonly referred to as a "stack trace." This stack trace is an invaluable tool for troubleshooting, as it provides a detailed account of the method calls leading up to the error. But when you need to capture or display this stack trace in a more human-readable format, things can get tricky. 😵🔄💥
The Solution: Throwable.getStackTrace() ⚙️💡🛠️
If you're working with Java, you're in luck! Java provides a handy method called Throwable.getStackTrace()
that returns an array of StackTraceElement
objects, each representing a single method call in the stack trace. 🙌📜
To convert this array into a string that depicts the stack trace, you have a few options. Let's explore two straightforward approaches:
Approach 1: Using the Arrays Class 📚🧮
One way to convert the stack trace to a string is by using the Arrays
class in Java. Here's an example snippet to demonstrate how to accomplish this:
import java.util.Arrays;
// ...
public static String stackTraceToString(Throwable throwable) {
return Arrays.toString(throwable.getStackTrace());
}
In this code snippet, we leverage the Arrays.toString()
method to convert the array of StackTraceElement
objects to a string representation. Easy peasy! 😎
Approach 2: Custom String Formatting 🖌️🎨🔤
Alternatively, you might prefer a more custom string formatting approach. Here's an example implementation:
public static String stackTraceToString(Throwable throwable) {
StringBuilder sb = new StringBuilder();
for (StackTraceElement element : throwable.getStackTrace()) {
sb.append(element.toString()).append("\n");
}
return sb.toString();
}
In this implementation, we iterate over each StackTraceElement
and append it to a StringBuilder
instance, separated by newline characters for improved readability. This approach gives you more control over the format of the resulting stack trace string.
Call-to-Action: Share Your Insights! 📣📝🗨️
Voila! You now have two easy-to-use methods for converting a stack trace to a string in Java. Time to put your newfound knowledge to the test! Try out these approaches in your next project and save yourself from countless hours of stack trace deciphering. 😄🚀
We'd love to hear from you! Have you encountered any other solutions for this problem? Is there a technique or library that you find particularly useful? Share your insights and experiences in the comments section below! Together, let's conquer the stack trace challenge! 💪💬✨
Happy coding! 👩💻👨💻
🔥💡📚🎯
Did you find this article helpful? Give it a 👍, and don't forget to share it with your fellow developers!
If you want to stay updated with the latest tech insights and tutorials, make sure to subscribe to our newsletter. You won't want to miss out on our upcoming articles! 📬👀
Follow us on Twitter (@TechBlog) for daily coding tips and tricks! Let's stay connected and continue learning together! 🐦💻🌟