How can I concatenate two arrays in Java?
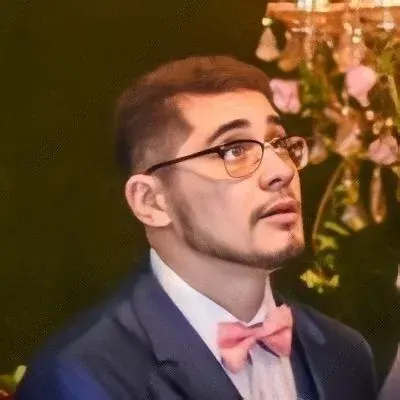
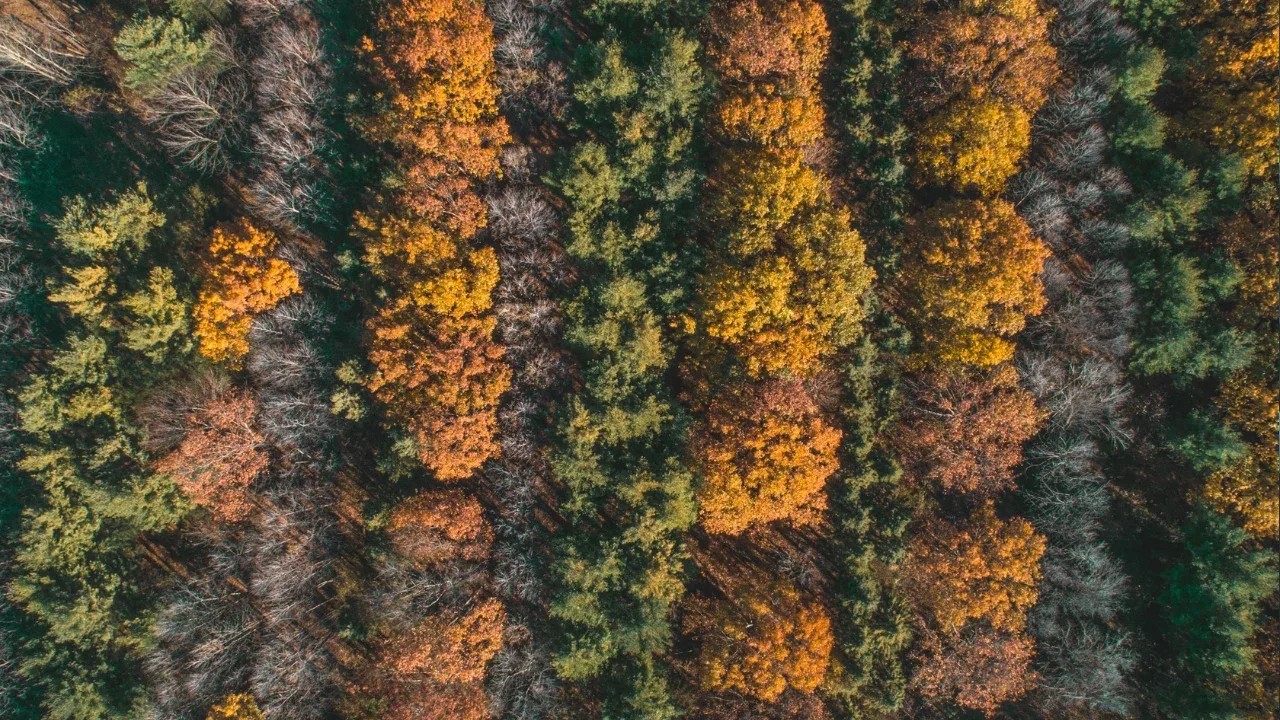
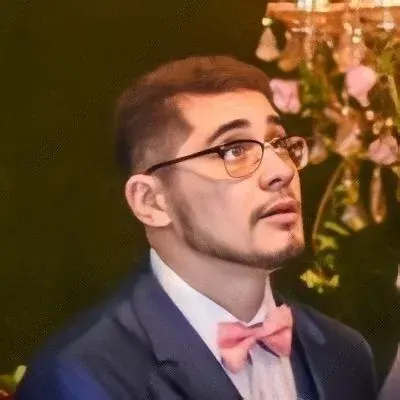
🌟 How to Concatenate Two Arrays in Java 🌟
So you want to concatenate two String
arrays in Java? I've got you covered! In this blog post, I will guide you through the process step by step and provide you with simple and effective solutions. Let's dive right in! 💪
The Problem: Concatenating Two Arrays
Here's the situation:
void f(String[] first, String[] second) {
String[] both = ???
}
You need to concatenate the first
and second
arrays to create a new array called both
. But how can you achieve this without tearing your hair out? Fear not, my friend, for I have some nifty solutions for you!
Solution #1: Using a Loop
One way to concatenate arrays in Java is by using a loop. Here's how you can do it:
void f(String[] first, String[] second) {
String[] both = new String[first.length + second.length];
int index = 0;
for (String str : first) {
both[index++] = str;
}
for (String str : second) {
both[index++] = str;
}
// Use the 'both' array here
}
In this solution, we create a new array both
with a size equal to the sum of the lengths of first
and second
arrays. Then, we use two separate loops to copy the elements from first
and second
to the both
array. The index
variable keeps track of the current position in the both
array.
Solution #2: Using System.arraycopy()
Java provides a handy method called System.arraycopy()
that allows you to efficiently copy elements from one array to another. Here's how you can use it to concatenate arrays:
void f(String[] first, String[] second) {
String[] both = new String[first.length + second.length];
System.arraycopy(first, 0, both, 0, first.length);
System.arraycopy(second, 0, both, first.length, second.length);
// Use the 'both' array here
}
In this solution, we create the both
array with the necessary length, and then we use System.arraycopy()
twice: once to copy the elements from first
to both
, and again to copy the elements from second
to both
. The second argument represents the starting index in the source array, the third argument represents the destination array, the fourth argument represents the starting index in the destination array, and the last argument represents the number of elements to be copied.
Call-to-Action: Share Your Thoughts!
Now that you know how to concatenate arrays in Java, why not take it for a spin? Implement one of the solutions I showed you or try out both to see which one you prefer. Then, come back and share your experience with me in the comments below! I'd love to hear from you! 🚀
Thanks for reading! If you found this blog post helpful, don't hesitate to share it with your fellow programmers. Happy coding! 😄✨