How can I clear or empty a StringBuilder?
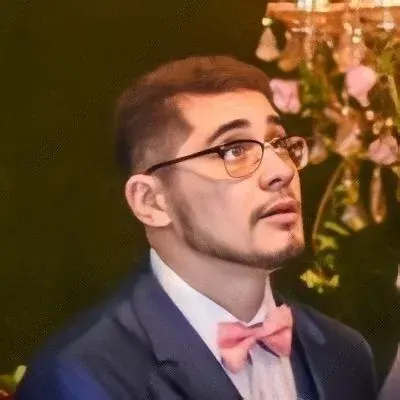
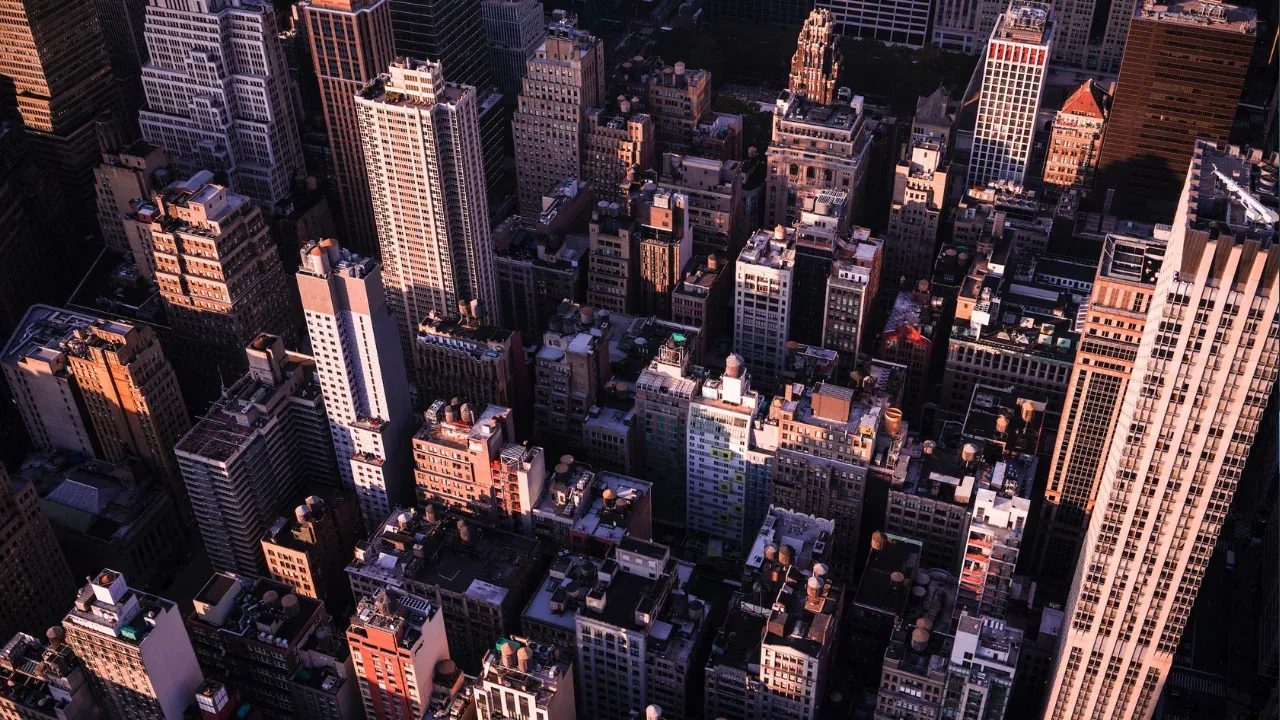
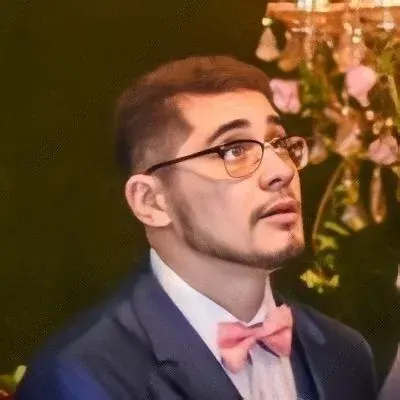
How to Clear a StringBuilder: A Complete Guide with Easy Solutions 👊💥🔄
So, you find yourself in a situation where you need to clear or empty a StringBuilder
in Java. You might have come across the StringBuilder.Clear
method in .NET and wondered why there isn't a similar method in Java's StringBuilder
documentation. Fret not, we have got you covered! In this guide, we will address this common issue and provide you with easy solutions. Let's dive in! 🏊♀️📚
The Dilemma 😕
You want to use a StringBuilder
in a loop, and every few iterations, you need to clear it and start fresh. After scouring the StringBuilder
documentation, you might have noticed that there isn't a direct StringBuilder.Clear
method available. Instead, you come across the seemingly complicated delete
method. But don't be discouraged! We have simpler alternatives to achieve the same goal. 🔄🚀
Solution 1: Creating a New StringBuilder 🆕
One simple solution is to create a brand new StringBuilder
object whenever you need to clear your existing StringBuilder
. This might seem counterintuitive at first, but it's actually an efficient approach. Here's an example:
StringBuilder myStringBuilder = new StringBuilder();
// ... your code here ...
myStringBuilder = new StringBuilder(); // Start fresh!
By initializing a new StringBuilder
, you effectively clear out the previous content and get a clean slate to work with. This solution is straightforward and guarantees a fresh StringBuilder
with minimal overhead. 💡🆓
Solution 2: Resetting Length to Zero 📏0️⃣
Another solution is to reset the length of your existing StringBuilder
to zero. This approach allows you to reuse the same StringBuilder
object without creating new instances. Here's how you can do it:
StringBuilder myStringBuilder = new StringBuilder();
// ... your code here ...
myStringBuilder.setLength(0); // Reset the length to zero!
By setting the length to zero, you essentially truncate the StringBuilder
content, effectively clearing it. This method is efficient since it doesn't involve object creation and saves memory. 🛡🔄
Solution 3: Reusing Existing StringBuilder by Deleting Content ✂️🔄
If you prefer to work with a single StringBuilder
object, you can use the delete
method to remove the content selectively. While it might seem more complex initially, it can be useful when you want to keep some portions and clear the rest. Here's how you can accomplish this:
StringBuilder myStringBuilder = new StringBuilder();
// ... your code here ...
myStringBuilder.delete(0, myStringBuilder.length()); // Delete everything!
The delete
method takes two arguments - start
and end
- to determine the portion of the content you want to remove. By starting from index zero and specifying the length of the existing StringBuilder
, you can effectively delete everything. Although this method may seem less intuitive, it's a powerful way to selectively clear content. ✂️❌🆓
Conclusion and Your Turn to Act 🎉🔧
There you have it! Three easy solutions to clear or empty a StringBuilder
in Java. Whether you choose to create a new StringBuilder
, reset the length to zero, or selectively delete content, you now have the tools to tackle this common issue with confidence. 💪🎯
Now it's your turn to put these solutions into action! Which method will you choose to clear your StringBuilder
? Let us know in the comments below! And don't forget to share this guide with your fellow Java developers who might be struggling with the same issue. Together, we can simplify even the trickiest problems! 🙌🚀
Happy coding! 💻😊