How can I avoid Java code in JSP files, using JSP 2?
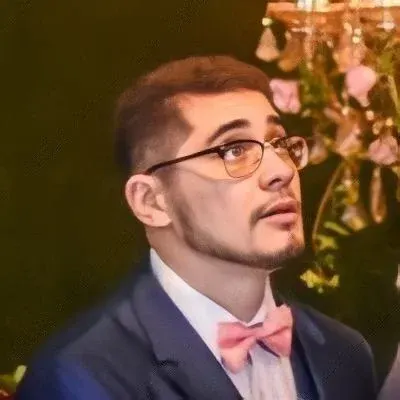
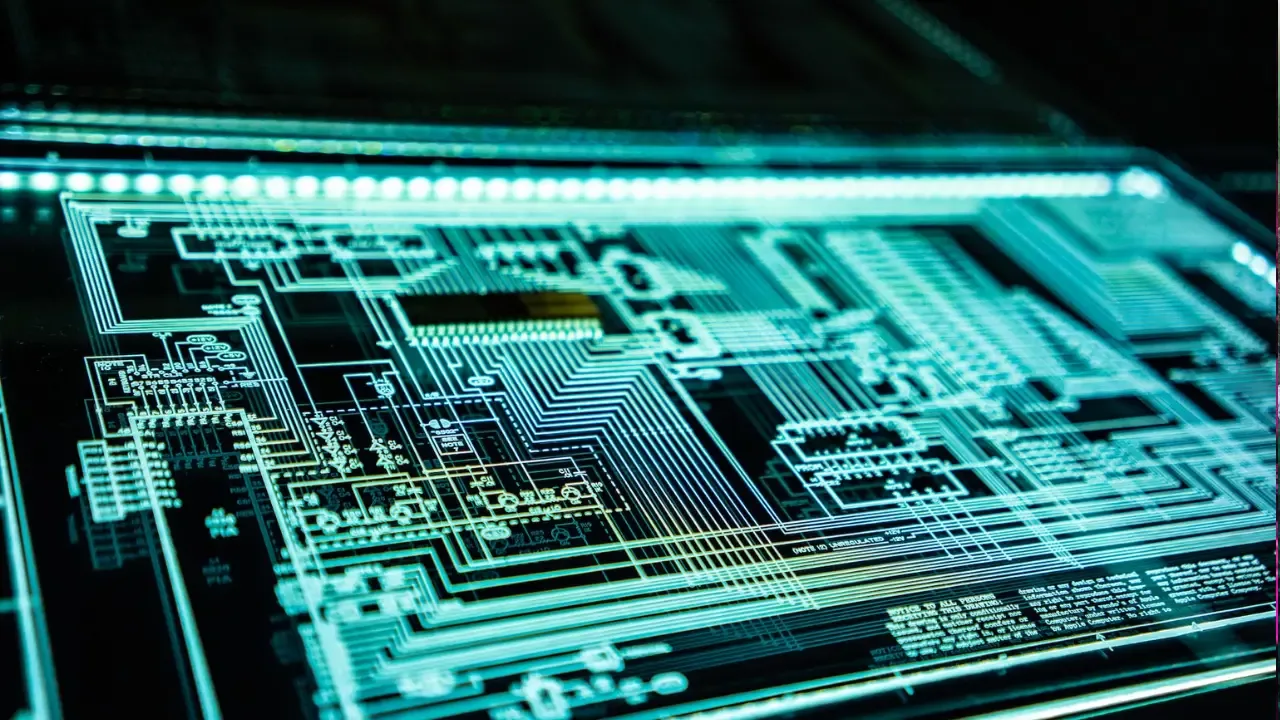
💻 Avoiding Java Code in JSP Files: A Complete Guide using JSP 2 🚀
Have you ever come across those old-school JSP files cluttered with lots of Java code? 🤔 It can be messy and difficult to maintain, right? But worry not, my friend! In JSP version 2, there's a cool technique that allows us to avoid Java code in JSP files entirely! 🙌
The Problem
Consider the following lines of code in a JSP file:
<%= x+1 %>
<%= request.getParameter("name") %>
<%! counter++; %>
This is called scriptlet code, and it's a direct way of embedding Java code into your JSP files. Sure, it gets the job done, but let's face it, it's not the most elegant or maintainable solution out there. 😅
The Solution: Expression Language (EL) 🎉
JSP version 2 introduced a concept called Expression Language (EL). EL is a simple, lightweight language that allows you to evaluate expressions directly within your JSP files, without the need for any Java code. Sounds amazing, right? 🌟
So how do we replace the old scriptlet code with EL expression? Let's take a look at the alternatives. 💪
Replace <%= x+1 %>
with ${x+1}
Instead of using scriptlet code, you can now use ${expression}
to evaluate and render the result directly. In our case, the replacement would look like this:
${x+1}
See how simple, clean, and readable it is now? It lets us focus more on the logic rather than getting buried in Java code. 🙌
Replace <%= request.getParameter("name") %>
with ${param.name}
EL also provides a handy shortcut to access request parameters without any Java code. We can replace the old scriptlet code with ${param.name}
. For example:
${param.name}
Isn't this so much better? EL simplifies our code and makes it more maintainable. 🎉
Replace <%! counter++; %>
with ${pageContext.setAttribute("counter", counter+1)}
Now, handling page-level variables using EL is as easy as pie! Instead of using scriptlet code to increment a counter, we can leverage ${pageContext.setAttribute("counter", counter+1)}
. Let's check it out:
${pageContext.setAttribute("counter", counter+1)}
EL empowers us with a concise syntax to manage page-level variables without any Java code clutter. Isn't that just awesome? 😍
Conclusion
By embracing JSP version 2's Expression Language (EL), we can bid farewell to messy and cluttered JSP files filled with Java code. EL enables us to write clean, readable, and easily maintainable code. 🌈
So go ahead, update your JSP files, refactor the old scriptlet code, and embrace the power of EL! Your future self will thank you. 💪
If you found this guide helpful and want to learn more about web development tips and tricks, make sure to subscribe to our newsletter for regular updates! 💌
Have you already made the switch from Java code in JSP files to using JSP 2? Share your thoughts and experiences in the comments section below! Let's learn and grow together! 🚀🌟
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
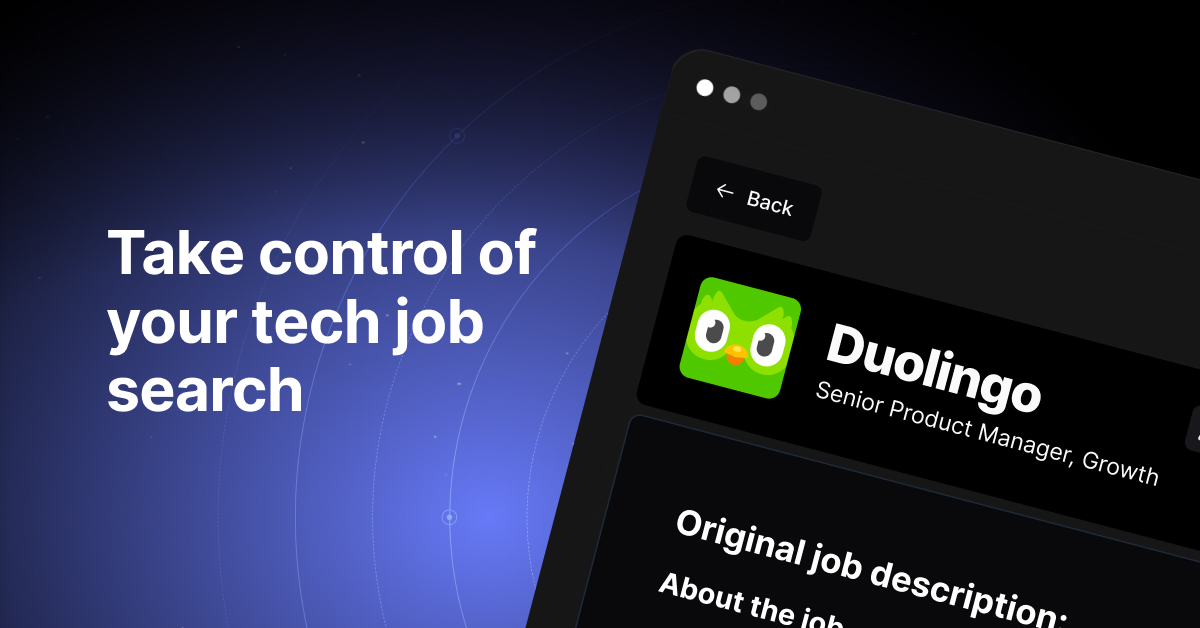