Gson: How to exclude specific fields from Serialization without annotations
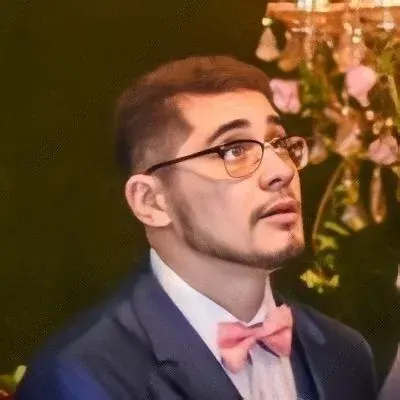
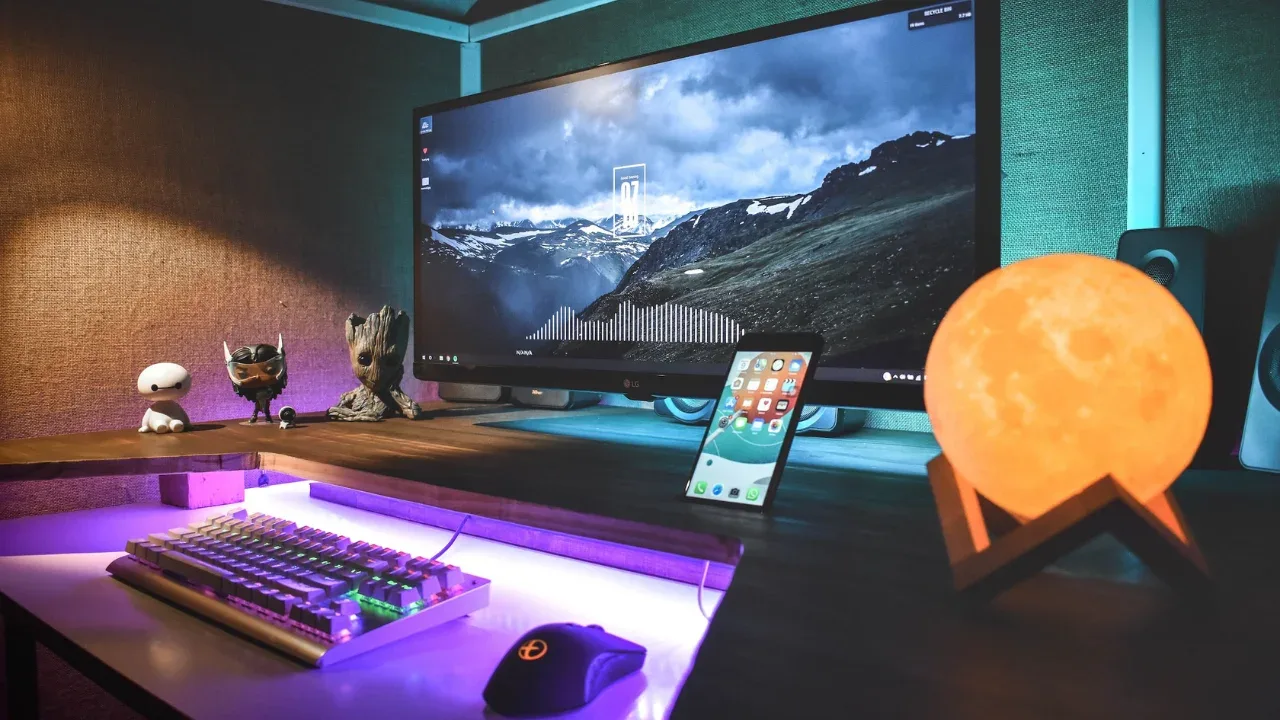
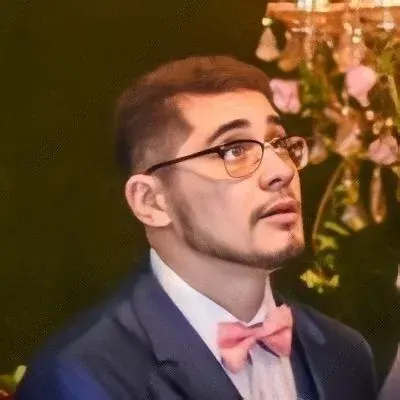
😎 How to Exclude Specific Fields from Serialization using Gson 😎
If you're struggling with field exclusion when working with Gson, you're not alone. Gson is a powerful library for converting Java objects to JSON and vice versa, but sometimes excluding specific fields from serialization can be a bit tricky, especially when you want to avoid using annotations. But fear not! In this guide, we'll walk you through the common issues and provide easy solutions to help you exclude those fields without breaking a sweat.
The Scenario
Let's say we have two classes, Student
and Country
, with the following structure:
public class Student {
private Long id;
private String firstName = "Philip";
private String middleName = "J.";
private String initials = "P.F";
private String lastName = "Fry";
private Country country;
private Country countryOfBirth;
}
public class Country {
private Long id;
private String name;
private Object other;
}
In this case, we want to exclude the name
field of the country
object from serialization. We also want to exclude it only for the country
object, but not for the countryOfBirth
object.
The Challenge
By default, Gson serializes all the fields in an object. To exclude specific fields, you can use a custom ExclusionStrategy
and the GsonBuilder
. However, the challenge arises when you need to exclude a property of a field, like country.name
.
The shouldSkipField(FieldAttributes fa)
method of the ExclusionStrategy
interface provides information about the field being serialized, but it doesn't contain enough information about the specific property we want to filter out.
The Solution
To overcome this challenge, we can slightly modify the structure of our classes and use a regular expression (RegEx) to filter out the desired fields.
public class Student {
private Long id;
private String firstName = "Philip";
private String middleName = "J.";
private String initials = "P.F";
private String lastName = "Fry";
private Country country;
private Country countryOfBirth;
// Getter and Setter for country
public Country getCountry() {
return country;
}
public void setCountry(Country country) {
this.country = country;
}
// Use a custom getter to exclude specific fields
public Country getCountryWithNameExcluded() {
country.setName(null);
return country;
}
}
public class Country {
private Long id;
private String name;
private Object other;
// Getters and Setters
// ...
}
In the Student
class, we added a custom getter getCountryWithNameExcluded()
that sets the name
field of the country
object to null
before returning it. This effectively excludes the name
field from serialization without any annotations.
Now, let's take a look at how we can use Gson to serialize the Student
object, excluding the country.name
field.
Gson gson = new GsonBuilder()
.setExclusionStrategies(new ExclusionStrategy() {
@Override
public boolean shouldSkipField(FieldAttributes fa) {
// Use RegEx to match the desired fields
String fieldName = fa.getDeclaringClass().getSimpleName() + "." + fa.getName();
return fieldName.matches("Country\\.name");
}
@Override
public boolean shouldSkipClass(Class<?> clazz) {
return false;
}
})
.create();
String json = gson.toJson(student.getCountryWithNameExcluded());
In this example, we create a custom ExclusionStrategy
and override the shouldSkipField()
method. We use a RegEx pattern (Country\\.name
) to match the desired field name (country.name
), and if there's a match, the field will be excluded from serialization.
🌟 Wrap Up
By leveraging a custom getter and using a RegEx pattern with Gson's ExclusionStrategy
, we can exclude specific fields from serialization without using annotations. This technique allows us to achieve fine-grained control over the exclusion of fields and can be easily extended to include more complex scenarios.
So, the next time you find yourself struggling with field exclusion in Gson, remember this guide and exclude those fields like a pro!
If you have any other questions or need further assistance, drop a comment below and let's discuss!
👉 Happy coding! 👈