Gson: Directly convert String to JsonObject (no POJO)
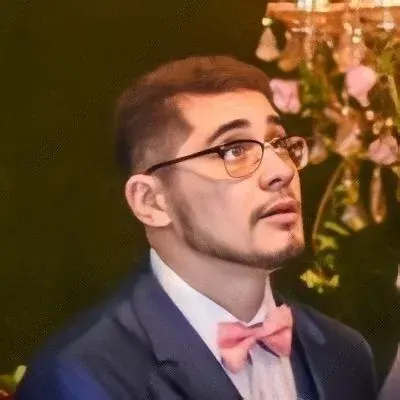
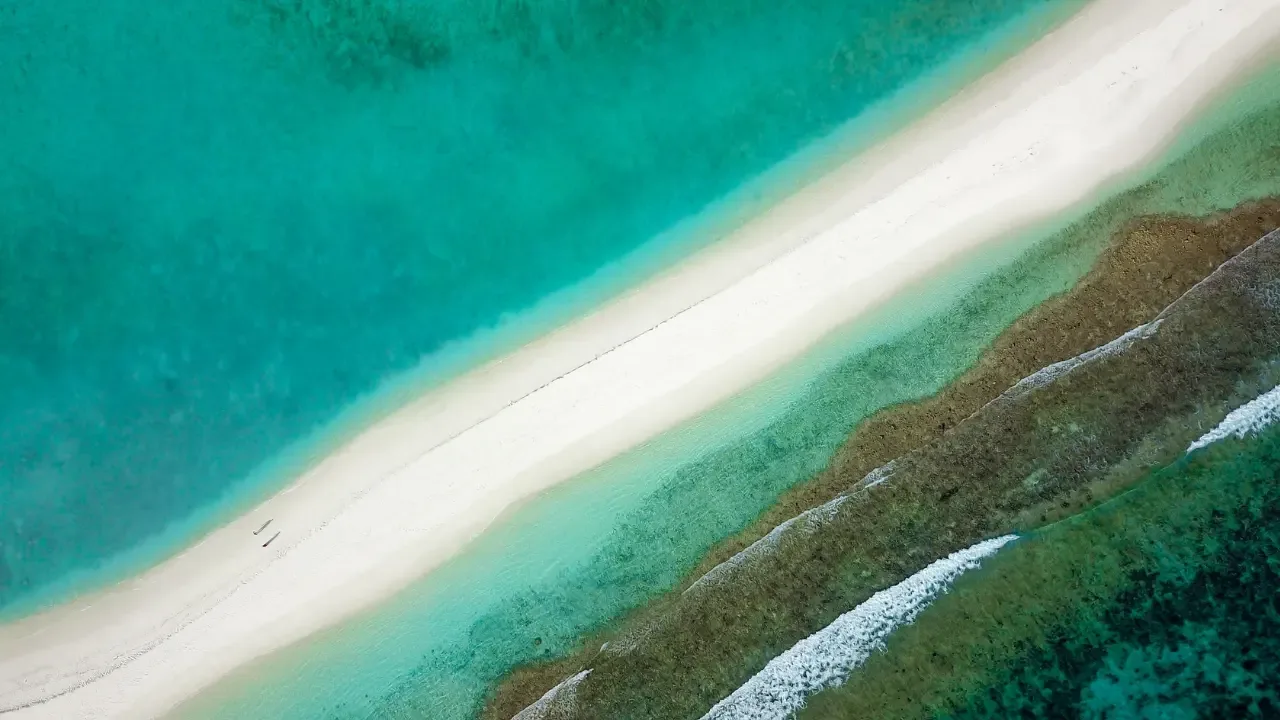
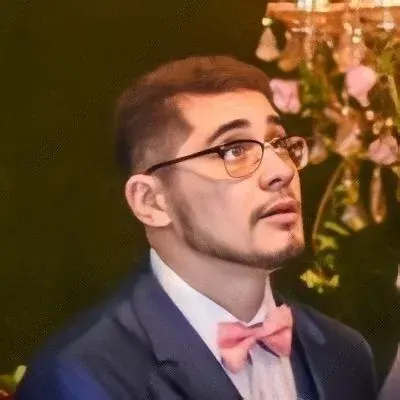
📝 Gson: Directly convert String to JsonObject (no POJO) 🌐
Are you trying to manipulate a JSON tree using GSON but facing the issue of not having a Plain Old Java Object (POJO) to convert a string into before converting it to JsonObject? Fear not! We have got you covered with an easy solution.
🤔 The Problem
The problem arises when you have a string representation of JSON and want to convert it directly into a JsonObject without going through the process of creating a POJO. This can be frustrating, especially if you are not familiar with all the dependencies and intricacies of GSON.
🔍 The Solution
Fortunately, there is a straightforward solution to this problem. You can use GSON's JsonParser
class to parse the string into a JsonElement
and then convert it into a JsonObject
. Here's how you can do it:
import com.google.gson.*;
public class GsonStringToJsonObjectExample {
public static void main(String[] args) {
String jsonString = "{ \"a\": \"A\", \"b\": true }";
JsonParser parser = new JsonParser();
JsonElement jsonElement = parser.parse(jsonString);
if (jsonElement.isJsonObject()) {
JsonObject jsonObject = jsonElement.getAsJsonObject();
// You now have your JsonObject
System.out.println(jsonObject);
} else {
// Handle the case when the parsed JSON is not a valid object
System.out.println("Invalid JSON object");
}
}
}
In this example, we first create an instance of JsonParser
. Then, we use the parse()
method to parse the JSON string into a JsonElement
. We can then perform checks on the parsed element to ensure that it is a Json object using isJsonObject()
. Finally, we convert the JsonElement
into a JsonObject
using getAsJsonObject()
.
And there you have it! You can directly convert a string into a JsonObject
without the need for a POJO.
💡 Example Usage
Let's see this in action using the example provided in the context:
import com.google.gson.*;
public class GsonStringToJsonObjectExample {
public static void main(String[] args) {
String jsonString = "{ \"a\": \"A\", \"b\": true }";
JsonParser parser = new JsonParser();
JsonElement jsonElement = parser.parse(jsonString);
if (jsonElement.isJsonObject()) {
JsonObject jsonObject = jsonElement.getAsJsonObject();
System.out.println(jsonObject);
} else {
System.out.println("Invalid JSON object");
}
}
}
Output:
{"a":"A","b":true}
💪 Take It Further
Now that you know how to directly convert a string into a JsonObject
, you can efficiently perform JSON tree manipulation using GSON without the need for additional POJOs.
Experiment with different JSON strings and explore the wide range of functionalities offered by GSON for JSON manipulation.
✨ Conclusion
In this guide, we learned how to convert a string directly into a JsonObject
without the need for a POJO using GSON. We also explored an example and encouraged you to dive deeper into JSON tree manipulation with GSON.
So go ahead, try it out, and start manipulating JSON trees like a pro!
If you have any questions or thoughts to share, don't hesitate to leave them in the comments below. Let's keep the conversation flowing! 💬👇
Happy coding! 🚀💻