Getting Spring Application Context
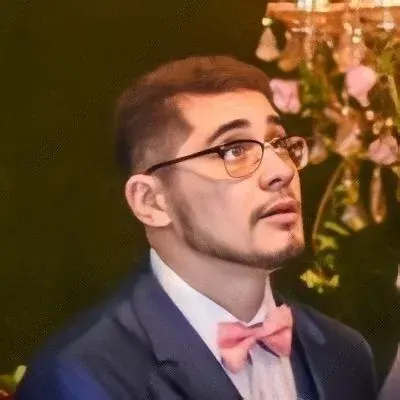
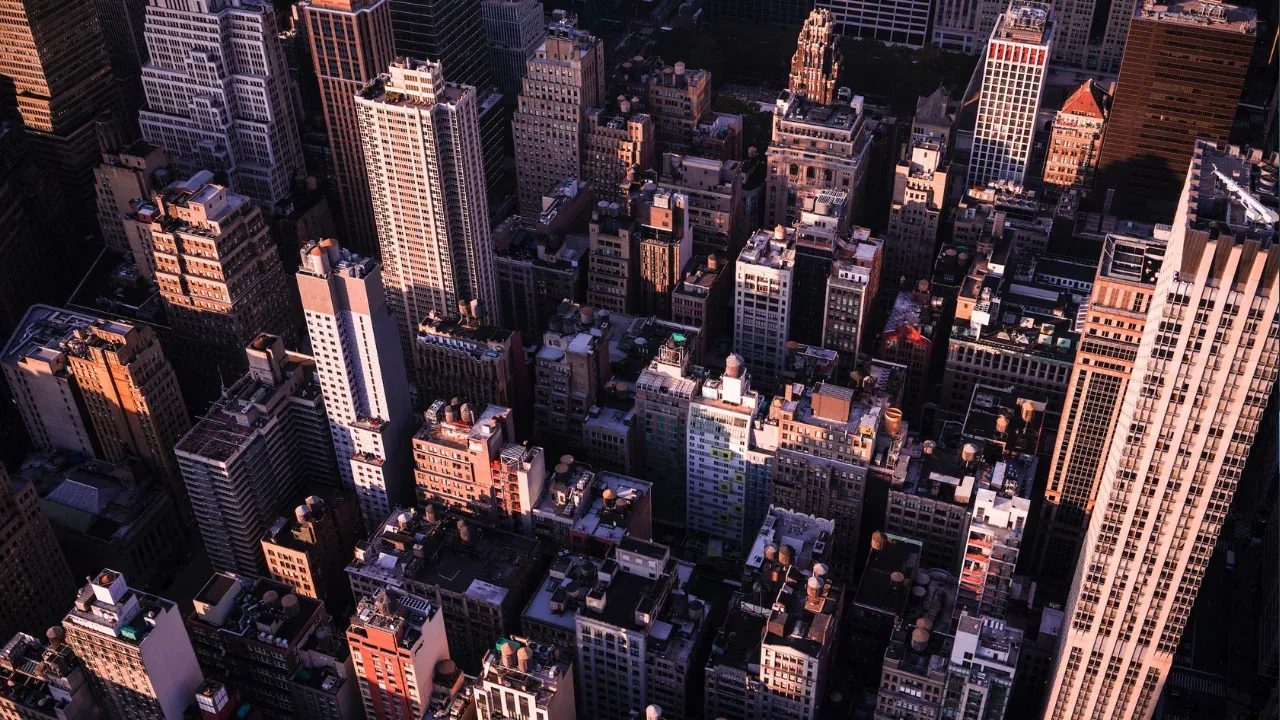
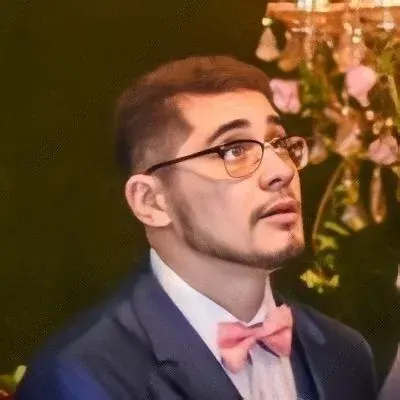
📣 Easy-Peasy Guide to Getting the Spring Application Context 🌱
So, you've stumbled upon the complicated world of Spring application context! 😰 Don't worry, my friend, I've got your back with this handy-dandy guide that will make your life so much easier. Let's dive in! 💦
Understanding the Problem 🧐
The problem at hand is accessing the ApplicationContext in a Spring application without explicitly passing it down through the call stack. 🔄 You're right to assume that the ApplicationContext is a singleton, as Spring only creates one instance of it throughout the lifetime of your application. 🌟
Common Issues and Solutions 💡
Issue 1️⃣: Passing ApplicationContext through the call stack
One common approach is to pass the ApplicationContext through the call stack by using constructor injection or method parameters. But let's be real, who wants all that extra hassle and clutter in their code? Not you, my friend! 😎
Solution 1️⃣: Implementing the ApplicationContextAware interface
Spring comes to the rescue with the ApplicationContextAware interface. By implementing this interface, your class can get a reference to the ApplicationContext without passing it around like a hot potato. 👐
Here's an example:
public class MyCoolClass implements ApplicationContextAware {
private static ApplicationContext applicationContext;
@Override
public void setApplicationContext(ApplicationContext context) throws BeansException {
applicationContext = context;
}
}
Now, you can access the ApplicationContext anywhere in your class like this:
MyCoolBean bean = MyCoolClass.applicationContext.getBean(MyCoolBean.class);
No need to pass the ApplicationContext all over the place anymore! 🙌
Issue 2️⃣: Accessing ApplicationContext in non-Spring managed classes
But what if you need the ApplicationContext in a class that Spring doesn't manage? 🤷♀️ Fear not, my friend, there's a solution for that too!
Solution 2️⃣: Using the ApplicationContextProvider
We can create a simple ApplicationContextProvider class to hold a reference to the ApplicationContext, making it accessible anywhere in your application. Let's see how it's done!
public class ApplicationContextProvider implements ApplicationContextAware {
private static ApplicationContext applicationContext;
public static ApplicationContext getApplicationContext() {
return applicationContext;
}
@Override
public void setApplicationContext(ApplicationContext context) throws BeansException {
applicationContext = context;
}
}
Now, you can access the ApplicationContext in any non-Spring managed class like this:
ApplicationContext context = ApplicationContextProvider.getApplicationContext();
MyCoolBean bean = context.getBean(MyCoolBean.class);
Voila! Now you can access the ApplicationContext from anywhere, even in classes that Spring doesn't manage. 🎉
The Call-to-Action! 📣
I hope this guide has saved you from the headaches of passing the ApplicationContext through your call stack. If you found this post helpful, leave a comment sharing your thoughts and experiences! 👇
And don't forget to share this post with your fellow Spring developers who could use a hand in taming the wild ApplicationContext beast. Sharing is caring, after all! 💖