Get list of JSON objects with Spring RestTemplate
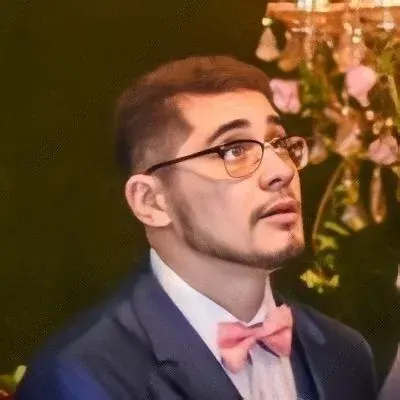
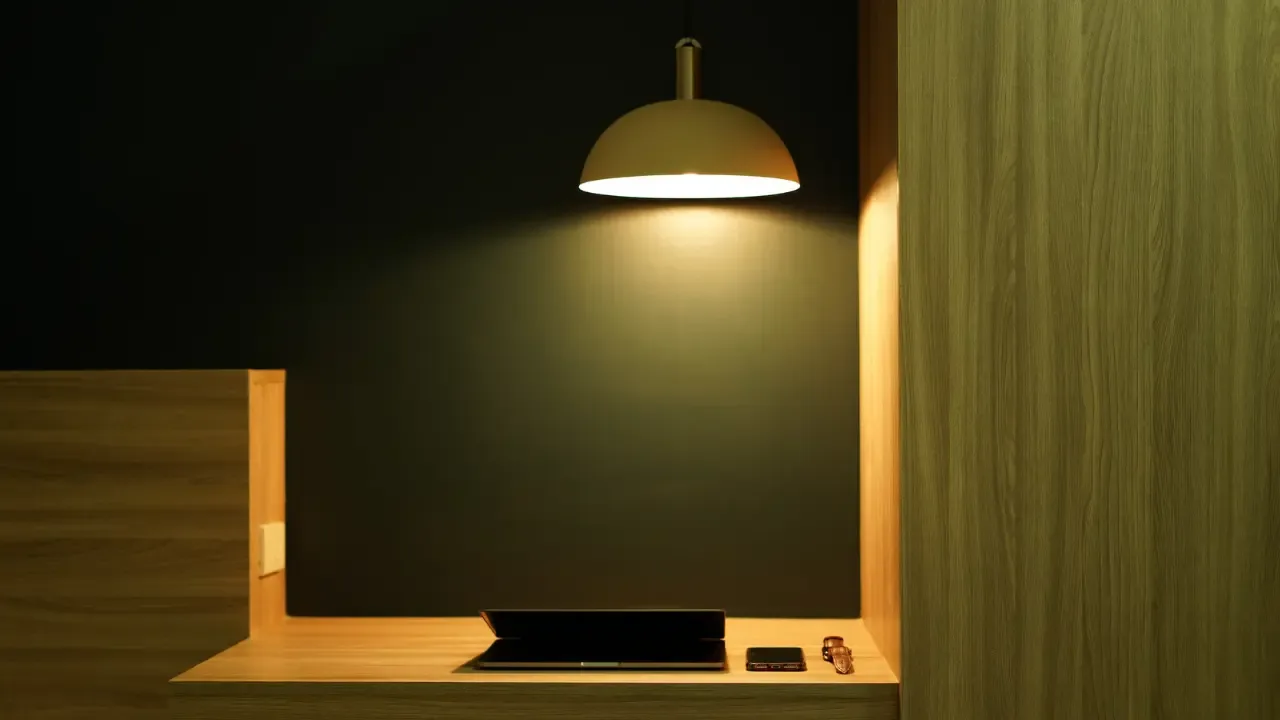
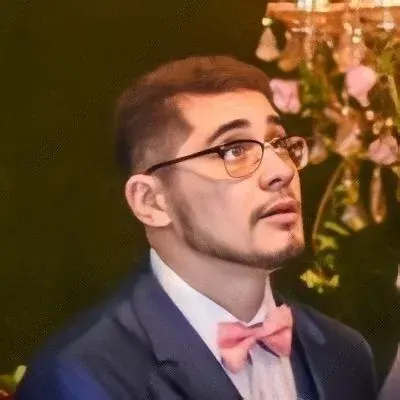
š Title: How to Easily Get a List of JSON Objects with Spring RestTemplate
š” Introduction: Are you struggling with mapping a list of JSON objects using Spring RestTemplate? Do you want to learn how to handle nested JSON objects effortlessly? Look no further! In this guide, we will address common issues surrounding this topic and provide easy solutions to help you get the desired results. Whether you're a beginner or an experienced developer, this blog post will provide valuable insights. So let's dive in!
š Problem 1: Mapping a List of JSON Objects The first question we'll tackle is how to map a list of JSON objects using Spring RestTemplate. Let's take an example scenario where we want to consume the JSON data from the BitPay API.
š Step 1: Set Up the RestTemplate To begin with, we need to set up the RestTemplate in our Spring application. Here's how you can do it:
RestTemplate restTemplate = new RestTemplate();
š Step 2: Define the Object Model Next, we need to define an object model that matches the structure of the JSON data we want to map. For example, let's say the JSON data has fields like "currency" and "rate". We can create a corresponding Java class:
public class Rate {
private String currency;
private double rate;
// Generate getters and setters
}
š Step 3: Make the API Request To retrieve the JSON data and map it to our object model, we can use the following code:
List<Rate> rates = restTemplate.exchange(
"https://bitpay.com/api/rates",
HttpMethod.GET,
null,
new ParameterizedTypeReference<List<Rate>>() {}
).getBody();
š Solution: You've Successfully Mapped a List of JSON Objects! By following these three simple steps, you can now easily get a list of JSON objects using Spring RestTemplate. Feel free to customize the object model according to your JSON structure, and explore the retrieved data in your application.
š” Tips:
Ensure that the JSON structure matches your object model to avoid any mapping errors.
Use appropriate error handling techniques when making API requests to handle exceptions gracefully.
š Problem 2: Mapping Nested JSON Objects Now, let's address the second question of mapping nested JSON objects. This is particularly useful when dealing with complex JSON structures. We will continue using the BitPay API example.
š Step 1: Update the Object Model In order to map nested JSON objects, we need to update our object model. Let's modify the previous "Rate" class to include a nested object called "data":
public class Rate {
private String currency;
private double rate;
private Data data;
// Generate getters and setters
}
public class Data {
private String code;
private int status;
// Generate getters and setters
}
š Step 2: Retrieve the Nested JSON To retrieve the nested JSON data, we can modify our API request code as follows:
List<Rate> rates = restTemplate.exchange(
"https://bitpay.com/api/rates",
HttpMethod.GET,
null,
new ParameterizedTypeReference<List<Rate>>() {}
).getBody();
š Solution: You've Successfully Mapped Nested JSON Objects! By updating our object model and following similar steps as before, you can now effortlessly map nested JSON objects using Spring RestTemplate. Retrieve the desired nested data from the mapped objects and utilize it in your application.
š” Call-to-Action: Share Your Experience and Engage! We hope this guide helped you overcome the challenges of mapping JSON objects with Spring RestTemplate. Now, it's your turn! Share your experiences, tips, and any additional insights in the comments section below. Let's engage and learn from each other's experiences!
⨠Conclusion: In this blog post, we covered how to map a list of JSON objects and handle nested JSON objects using Spring RestTemplate. We provided step-by-step solutions, along with useful tips, to simplify the process for you. Remember, practice makes perfect, so don't hesitate to experiment with different JSON structures and explore more advanced features of RestTemplate. Happy mapping!