Get an OutputStream into a String
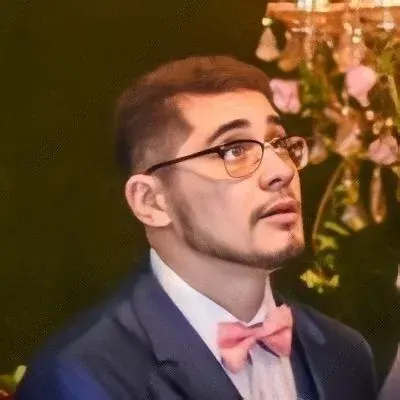
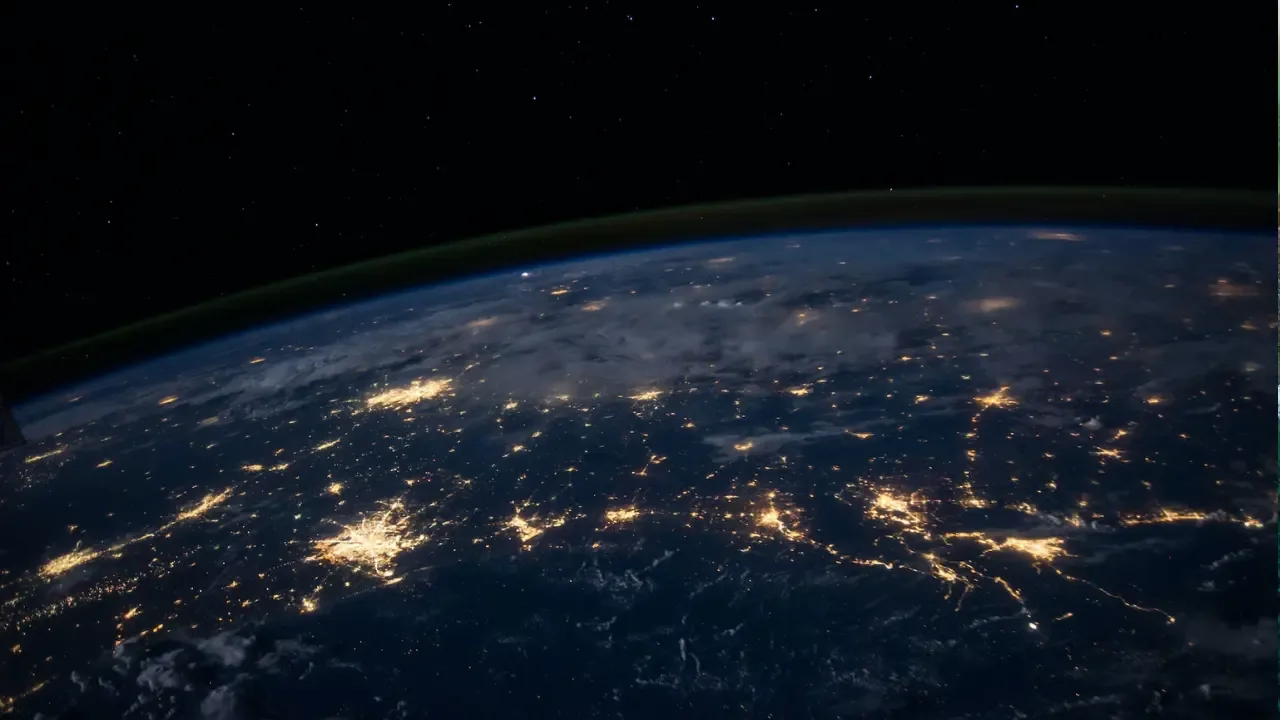
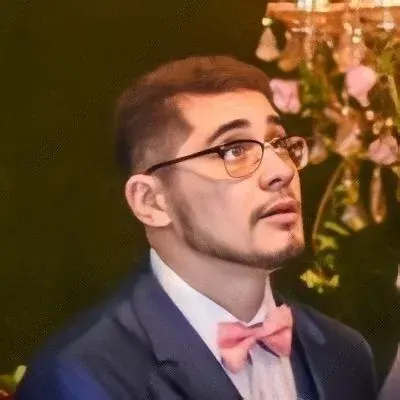
๐ Tech Blog: "๐ฅ Getting an OutputStream into a String in Java ๐ฅ"
Hey there, tech enthusiasts! ๐ Are you wondering how to pipe ๐บ the output from an java.io.OutputStream
to a String
in Java? Look no further! ๐ In this post, we will address this common issue and provide you with easy solutions.
So, imagine you have a method called writeToStream(Object o, OutputStream out)
that writes certain data from an object to the given stream. But what if you want to capture this output conveniently into a String
? Let's explore some solutions! ๐ก
One approach you might consider is creating a custom class called StringOutputStream
(like the one mentioned in the question). This class extends OutputStream
and contains a StringBuilder
for accumulating the output. Here's an example:
class StringOutputStream extends OutputStream {
StringBuilder mBuf;
public void write(int byte) throws IOException {
mBuf.append((char) byte);
}
public String getString() {
return mBuf.toString();
}
}
This implementation overrides the write
method to append each byte to the StringBuilder
and provides a getString
method to retrieve the accumulated output as a String
. Simple, right? ๐
Now, you might be wondering if there's a better way to achieve the same result without creating a custom class. Well, the good news is that Java provides a handy class called ByteArrayOutputStream
. ๐
By using ByteArrayOutputStream
, you can easily convert the output into a byte[]
, which can then be transformed into a String
. Here's an example:
ByteArrayOutputStream out = new ByteArrayOutputStream();
writeToStream(object, out);
String output = out.toString();
In this example, we create a ByteArrayOutputStream
out
, pass it to the writeToStream
method, and finally convert the accumulated bytes to a String
using the toString
method. Voila! ๐
Using ByteArrayOutputStream
is a more conventional and straightforward approach in Java. It saves you from reinventing the wheel and keeps your code clean and readable. ๐งน
Before we wrap up, let's emphasize that writing tests is always wise! ๐งช For situations like this one, where you only want to run a test, implementing a lightweight solution like the StringOutputStream
class mentioned earlier might be a good option. But in general, we recommend the ByteArrayOutputStream
approach for its simplicity and compatibility with existing Java codebases.
Now that you have a couple of solutions under your belt, go ahead and give them a try! Experiment, have fun, and let us know your thoughts. ๐ญ
Share your experience with us in the comments below! Have you ever encountered a different approach to this problem? We'd love to hear about it! Let's connect and learn from each other. ๐ฅโจ
And remember, keep exploring, keep learning! ๐ Happy coding! ๐๐ป