Generate Java classes from .XSD files...?
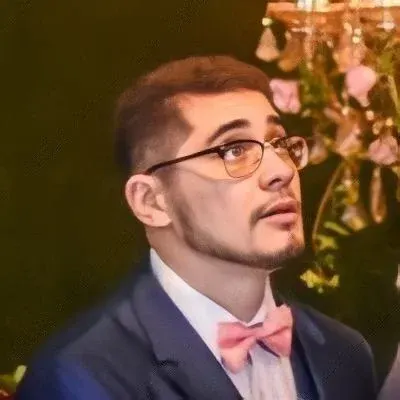
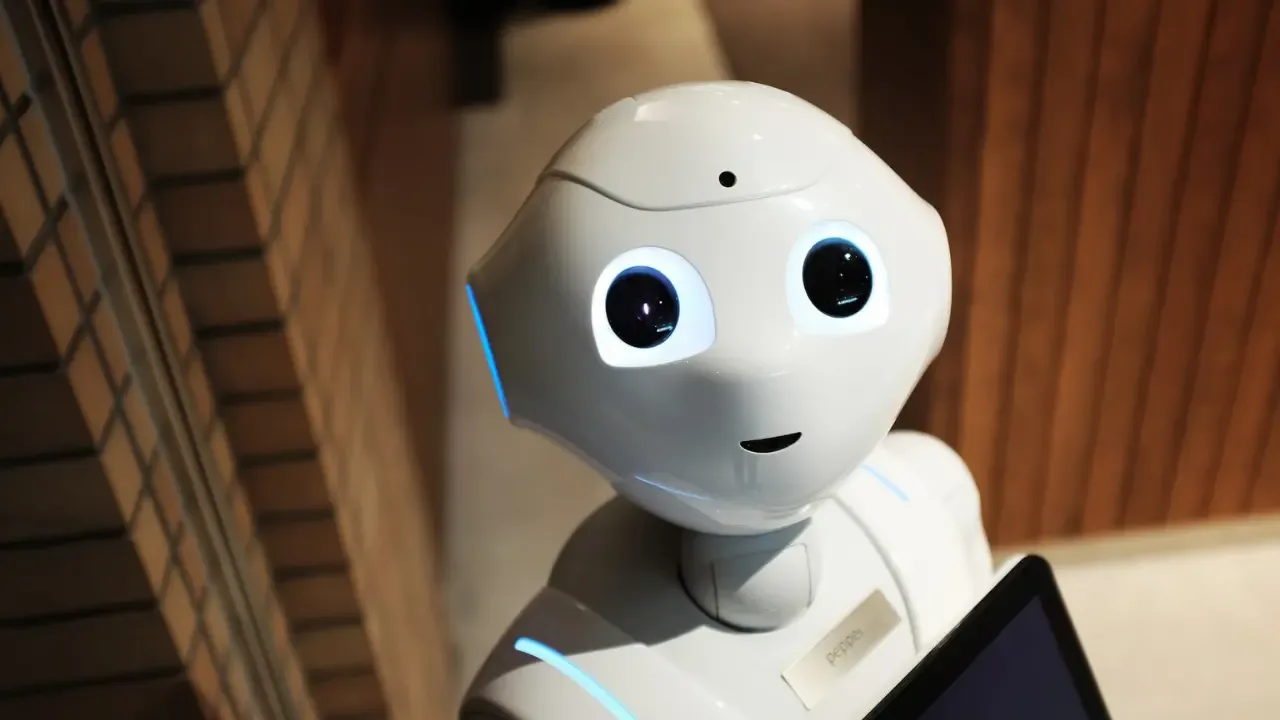
How to Generate Java Classes from .XSD Files
So, you've got a gigantic QuickBooks SDK .XSD schema file and you want to generate Java classes from it? Don't worry, we've got you covered! 🙌
The Problem
Before we dive into the solution, let's understand the problem. The .XSD (XML Schema Definition) file defines the structure and data types of the XML requests and responses for the QuickBooks API. You want to be able to easily generate Java classes from these .XSD files to conveniently marshal XML to Java objects and vice versa.
The Solution
Solution 1: JAXB (Java Architecture for XML Binding)
JAXB is a powerful Java library that allows you to generate Java classes from XML schema files (.XSD). Here's how you can use it:
Make sure you have the Java Development Kit (JDK) installed on your machine.
Add the JAXB dependencies to your project. You can do this by including the following code snippet in your project's
pom.xml
file if you are using Maven:
<dependencies>
<dependency>
<groupId>javax.xml.bind</groupId>
<artifactId>jaxb-api</artifactId>
<version>3.0.1</version>
</dependency>
<dependency>
<groupId>com.sun.xml.bind</groupId>
<artifactId>jaxb-impl</artifactId>
<version>3.0.0</version>
</dependency>
</dependencies>
Create a JAXB binding file (e.g.,
bindings.xml
) where you define the mappings between XML elements and Java classes. Here's an example:
<jaxb:bindings version="1.0" xmlns:jaxb="http://java.sun.com/xml/ns/jaxb" xmlns:xsd="http://www.w3.org/2001/XMLSchema">
<jaxb:bindings schemaLocation="your-schema.xsd">
<jaxb:bindings node="//xsd:complexType[@name='YourComplexType']">
<jaxb:class name="YourClass"/>
</jaxb:bindings>
</jaxb:bindings>
</jaxb:bindings>
Run the JAXB binding compiler (
xjc
) tool to generate the Java classes. Open your terminal or command prompt and navigate to the directory where yourbindings.xml
file is located. Execute the following command:
xjc -b bindings.xml -d src/main/java your-schema.xsd
This command tells the xjc
tool to use the bindings.xml
file and generate the Java classes in the src/main/java
directory.
That's it! You now have the Java classes generated from the .XSD file.
Solution 2: XMLBeans
If you prefer an alternative to JAXB, you can use XMLBeans. XMLBeans is another Java library that provides tools for working with XML data using generated Java classes. Here's how you can use it:
Download the XMLBeans library from Apache XMLBeans and extract it.
Add the XMLBeans JAR file to your project's classpath. You can do this by including the following code snippet in your project's
pom.xml
file if you are using Maven:
<dependencies>
<dependency>
<groupId>org.apache.xmlbeans</groupId>
<artifactId>xmlbeans</artifactId>
<version>3.1.0</version>
</dependency>
</dependencies>
Run the
scomp
tool provided by XMLBeans to generate the Java classes. Open your terminal or command prompt and navigate to the directory where you extracted the XMLBeans library. Execute the following command:
scomp -out src/main/java -compiler "javac" your-schema.xsd
This command tells the scomp
tool to generate the Java classes in the src/main/java
directory using the javac
compiler.
Easy peasy! You now have the Java classes generated from the .XSD file using XMLBeans.
Conclusion
Generating Java classes from .XSD files is no longer a challenge with the help of JAXB or XMLBeans. Choose the solution that suits your project requirements and start marshaling XML to Java objects effortlessly.
Got any questions or alternative approaches? Share your thoughts in the comments below! Let's brainstorm together! 💡
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
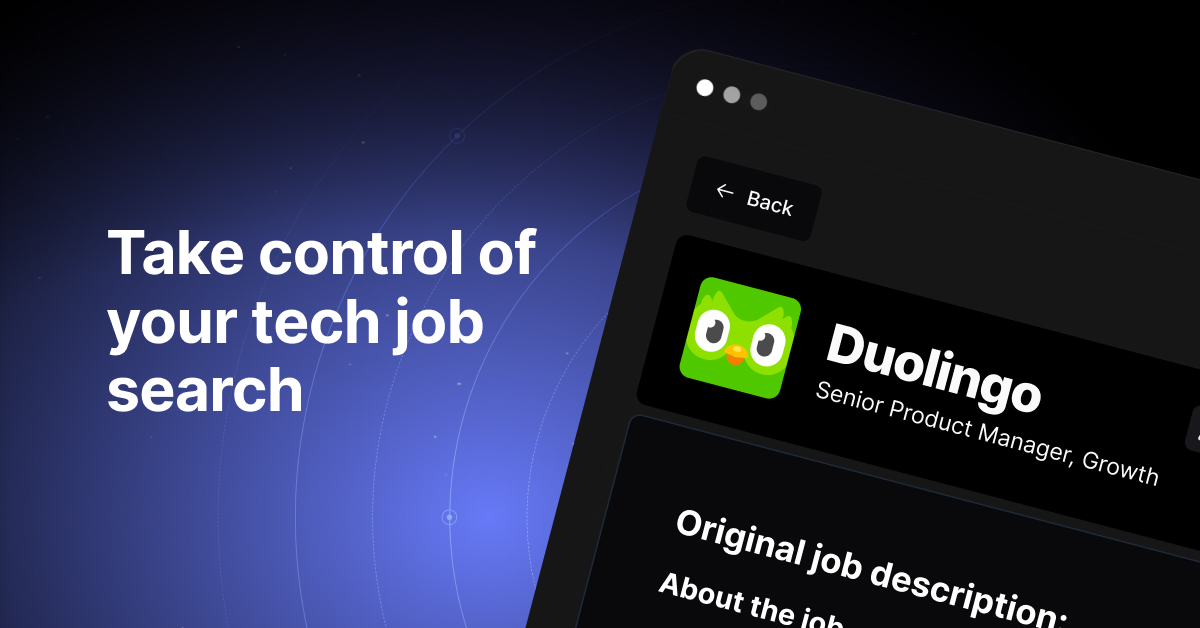