File to byte[] in Java
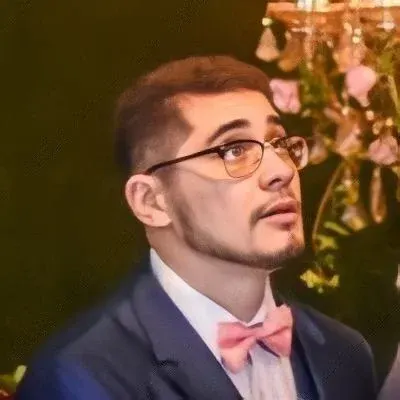
![Cover Image for File to byte[] in Java](https://images.ctfassets.net/4jrcdh2kutbq/376eUYzJAW7m4wV7PLqKoF/686c0d07d68f4a0bd05ecf1a1ec4bd01/Untitled_design__17_.webp?w=3840&q=75&fm=webp)
Converting a File to byte[] in Java: Unveiling the Mystery 📂➡️🔢
Do you ever find yourself in a situation where you need to convert a Java File object into a byte array? 🤔 Fear not, my friend! In this blog post, we'll dive into the depths of this common issue and provide you with some simple solutions. Let's unravel the mystery, shall we? 🧐
The Quest for Conversion 🏹
So, imagine you have a java.io.File
object representing a file on your system. You want to convert it into a byte[]
for further processing, manipulation, or transmission. But how can you achieve this transformation? 🤷♂️
The Traditional Approach 📜
One common approach to converting a File to byte[]
is by manually reading the file's contents and storing them in a byte array. Here's some code to demonstrate this:
import java.io.FileInputStream;
import java.io.IOException;
public class FileToByteArrayConverter {
public static byte[] convert(File file) throws IOException {
try (FileInputStream fis = new FileInputStream(file)) {
byte[] bytes = new byte[(int) file.length()];
fis.read(bytes);
return bytes;
}
}
// Other useful methods and functionalities
}
In the code above, we create a new FileInputStream
with the given File
object, read the file's contents into a byte array, and return it. We also take advantage of the try-with-resources statement to automatically close the file input stream.
However, this approach might not be suitable for large files, as it requires reading the entire file into memory at once. This can lead to memory issues if you're dealing with files that are excessively large. 😰
An Alternative Path 🌟
If you're concerned about memory usage or want a more efficient solution, fear not! Java provides an alternative way to convert a File to byte[]
using the java.nio.file
package. Let's take a look at how we can achieve this:
import java.nio.file.Files;
import java.nio.file.Path;
import java.io.IOException;
public class FileToByteArrayConverter {
public static byte[] convert(File file) throws IOException {
return Files.readAllBytes(file.toPath());
}
// Other useful methods and functionalities
}
In this alternative approach, we use the Files
class from the java.nio.file
package and its readAllBytes()
method. This method reads all the bytes from a file specified by its Path
and returns them as a byte array. It offers a more memory-efficient solution, as it avoids reading the entire file into memory at once.
Engage in the Conversion 🤗
Now that you have some solutions in your arsenal, you can easily convert a File to byte[]
with confidence! Whether you prefer the traditional method or the memory-efficient alternative, you can tackle this common challenge effortlessly. 💪
Just remember to handle any potential IOExceptions
that may arise when working with files, and perform any necessary error checking or validation to ensure your code behaves as expected.
Conclusion 🎉
Converting a File to byte[]
in Java doesn't have to be a daunting task. By using either the traditional method or the memory-efficient alternative, you can accomplish this conversion effortlessly and efficiently. So go ahead, empower your code to handle files with ease! 💥
If you found this guide helpful, or have any questions, feel free to leave a comment below. Let's engage in a delightful coding conversation! 🗣️💬
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
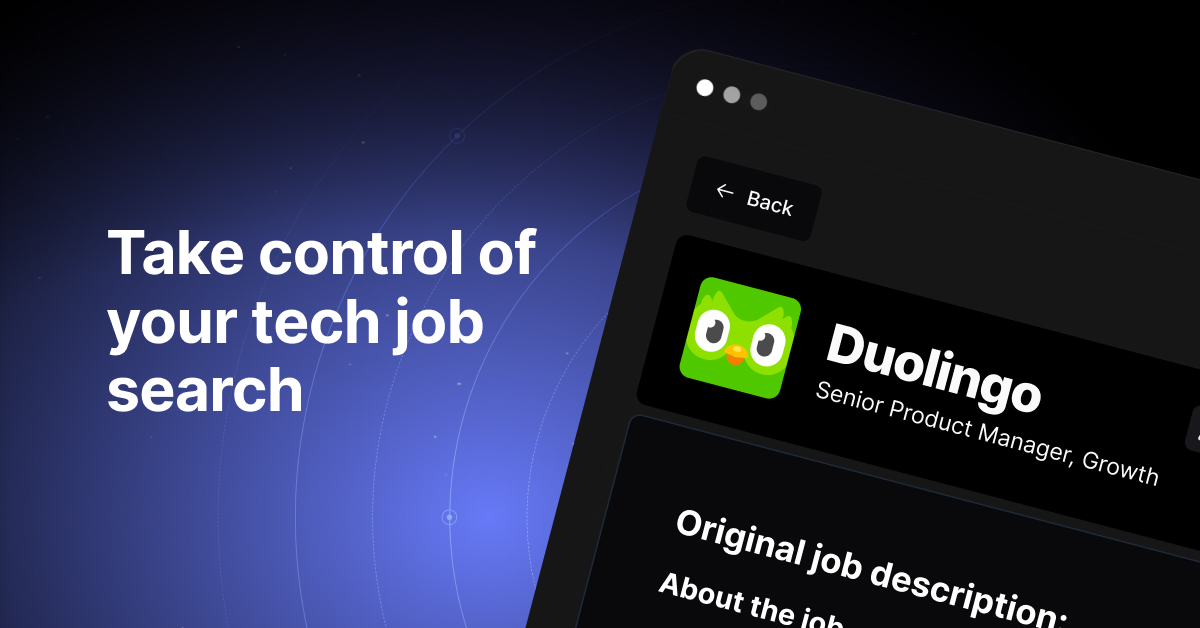