"Field required a bean of type that could not be found." error spring restful API using mongodb
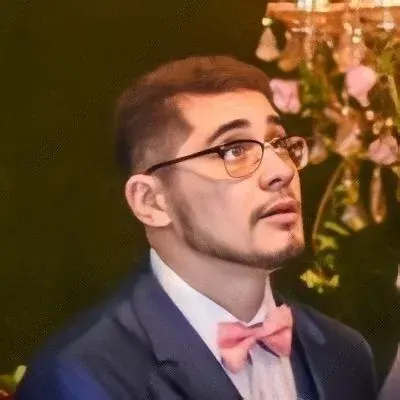
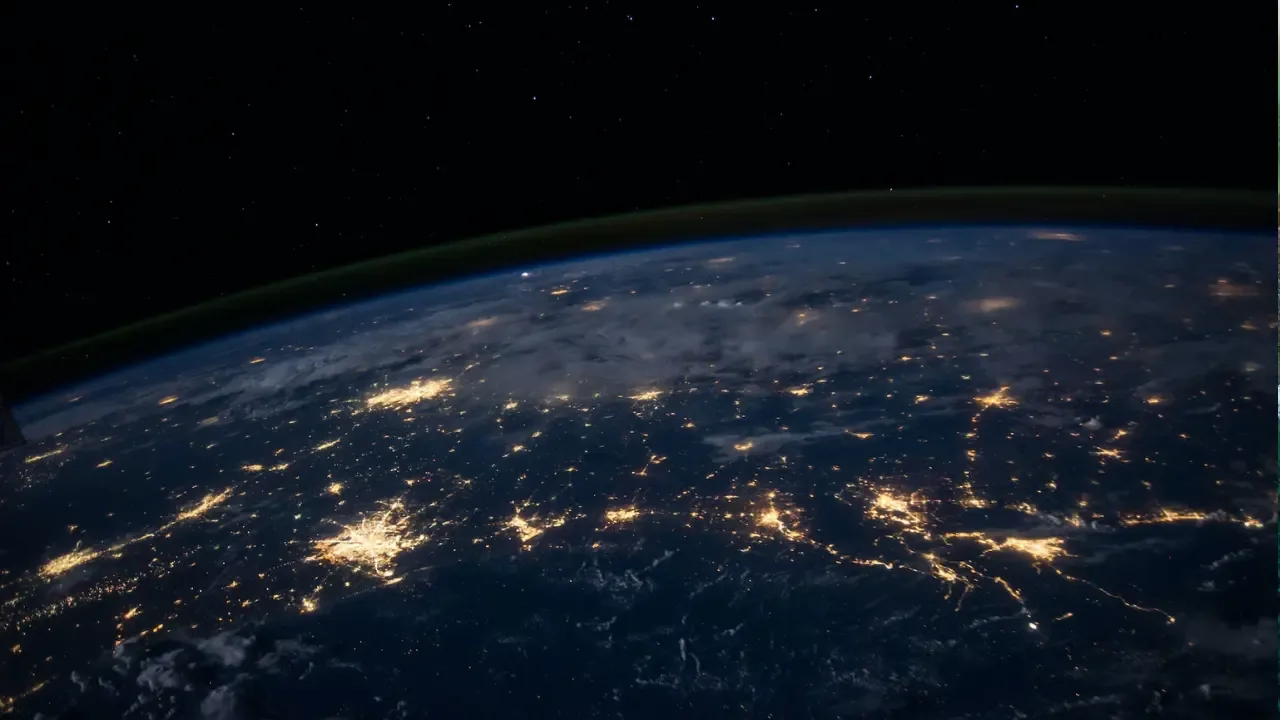
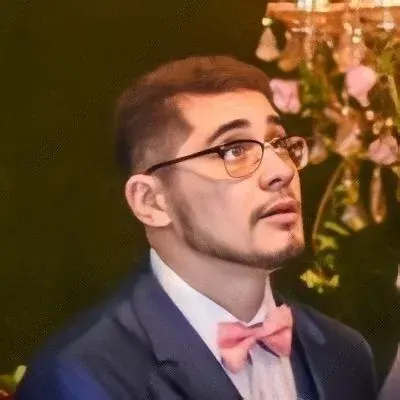
Field required a bean of type that could not be found error in Spring RESTful API with MongoDB
š Are you new to Spring and MongoDB, trying to build a RESTful API and faced with the "Field required a bean of type that could not be found" error? Don't worry, we've got you covered! š¤©
Understanding the error
When you encounter the "Field required a bean of type 'main.java.service.UserService' that could not be found" error, it means that Spring is unable to find the required bean for dependency injection. In simpler terms, Spring is saying that it cannot find an instance of UserService
to inject into the UsersController
.
Common causes of the error
Missing or incorrect bean configuration: This error often occurs when the required bean is not properly configured in the application context.
Missing annotation: If the
UserService
or related classes are missing the necessary annotations, Spring won't be able to identify them as beans.Incorrect package structure: Improper package structure could also lead to Spring's inability to locate the required bean.
Finding the solution
Now, let's dive into the details of how to solve this error.
1. Check bean configuration
First, ensure that your UserService
interface is correctly configured as a bean. In most cases, you don't need to explicitly define it as a bean because Spring provides automatic bean registration based on certain conditions.
To enable automatic bean registration, annotate the main.java.service
package (or any other package containing the UserService
implementation) with @ComponentScan
.
Here's how your Application
class should look after the modification:
package main.java.rest;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.ComponentScan;
@SpringBootApplication
@ComponentScan(basePackages = "main.java")
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
This change will make Spring scan the main.java
package and its sub-packages to find and register beans.
2. Ensure correct annotations are used
Make sure that you are using the correct annotations to annotate your classes. In the provided code snippets, the UserService
interface should be annotated with @Repository
.
@Repository
public interface UserService extends MongoRepository<User, String> {
List<User> findAll();
}
Additionally, don't forget to annotate the User
class with @Document
:
@Document(collection="user")
public class User {
// ... your code
}
3. Verify package structure
Double-check and ensure that your package structure is correct. Spring heavily relies on package scanning to locate and register beans. In the provided directory structure, it appears that your classes are in the correct location.
4. Verify dependency management
If you're using Gradle, make sure your build.gradle
file has the necessary dependencies for Spring Boot and MongoDB.
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-data-mongodb'
// ... other dependencies
}
5. Restart and rebuild application
After making the necessary changes, clean and rebuild your project. Then, restart your application to ensure all changes are applied.
Your next step
Give these solutions a try and see if the error persists. Remember, this error usually occurs due to misconfiguration, missing annotations, or incorrect package structure.
š If you found this article helpful, share it with others who might be facing a similar issue. You could be their hero! šŖ
š¤ Do you have any other questions or need further assistance? Leave a comment below, and we'll be happy to help you out! Let's conquer the world of Spring and MongoDB together! šš„