Fastest way to determine if an integer"s square root is an integer
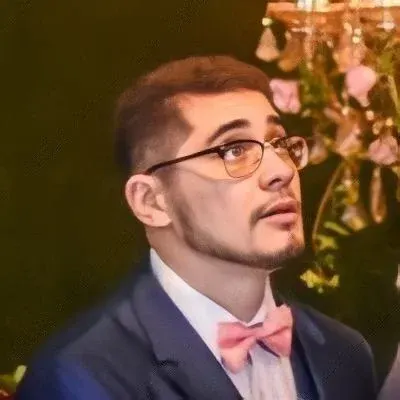
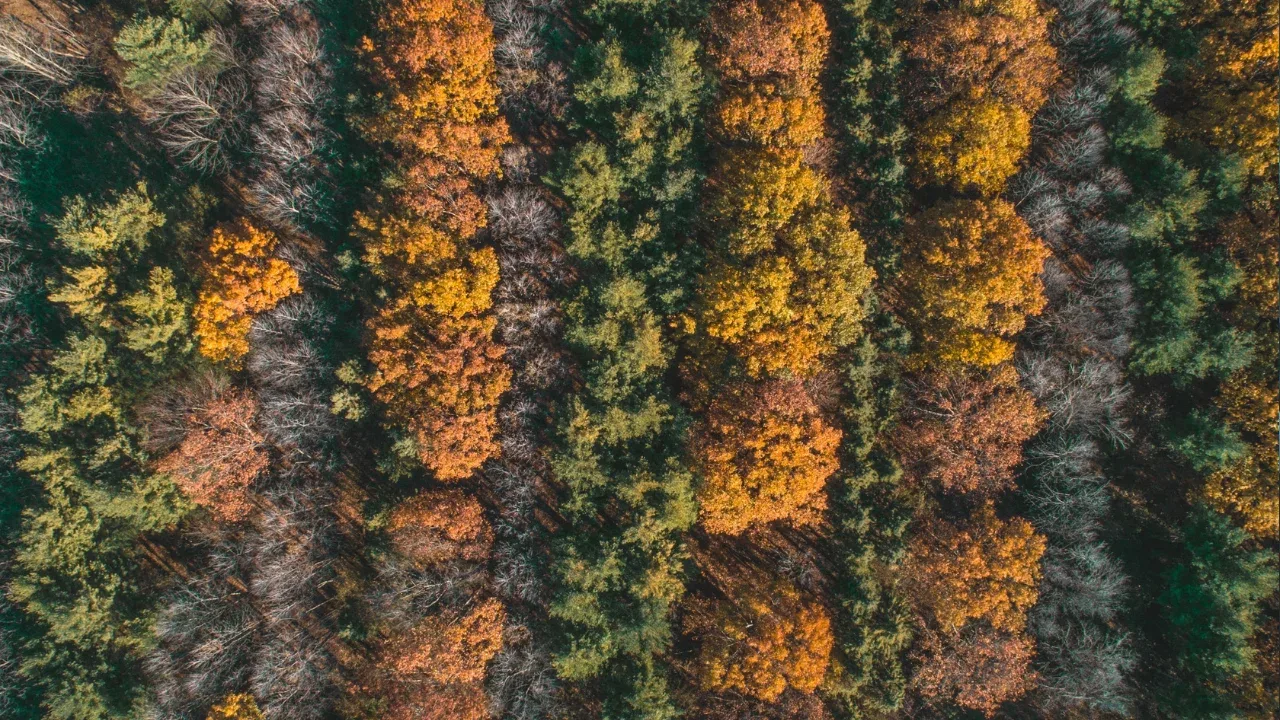
What's the Fastest Way to Determine if an Integer's Square Root is an Integer? 🚀
Are you tired of relying on the built-in Math.sqrt() function to determine if an integer is a perfect square? Looking for a faster solution that restricts itself to the integer domain? You're in luck! In this blog post, we'll explore different methods to solve this problem and find the fastest approach. Let's dive in! 💥
The Simple and Straightforward Way 💡
Here's the initial solution provided:
public final static boolean isPerfectSquare(long n) {
if (n < 0)
return false;
long tst = (long)(Math.sqrt(n) + 0.5);
return tst*tst == n;
}
This approach works fine, but can we make it faster? Let's find out! ⚡️
Evaluating Different Solutions ⚙️
After analyzing the problem, several alternative solutions were tested. Here's what we found:
Adding
0.5
to the result of Math.sqrt() is not necessary. It doesn't affect the accuracy of the solution, at least not on our machine. So you can simplify the function accordingly.The fast inverse square root method was faster, but it gave incorrect results for n >= 410,881. However, we can use the FISR hack for values below this threshold, as suggested by BobbyShaftoe.
Newton's method, a classic approach, was slower than Math.sqrt(). This is because Math.sqrt() likely uses a similar technique, but it's implemented in the hardware, making it much faster.
A modified version of Newton's method that only uses integer math was still slower than Math.sqrt(). Additionally, some hacks were needed to avoid overflow and ensure compatibility with all positive 64-bit signed integers.
Binary chop, while a valid method, was even slower. On average, it requires 16 passes to find the square root of a 64-bit number.
The performance difference between using
or
statements and aswitch
statement depends on the programming language. In C++,or
statements are faster, while in Java and C#, there's no noticeable difference.Creating a lookup table as a private static array of boolean values was slightly slower than the previous methods. This is because array bounds are checked in Java, impacting performance.
The Fastest Approach ⚡️⚡️⚡️
Based on our experiments, it seems that the initial straightforward solution involving Math.sqrt() is already quite efficient. Therefore, we recommend sticking with that approach. Attempts to optimize it further resulted in either incorrect results or slower execution times.
Your Turn! 🎉
Now that you have a deeper understanding of determining if an integer's square root is an integer, it's time to put that knowledge into practice. Try implementing the initial straightforward solution or any of the alternatives we discussed. Experiment and see which one works best for your specific use case.
Don't forget to share your experience and results with us! We'd love to hear your feedback and learn about any unique approaches you discover.
Happy coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
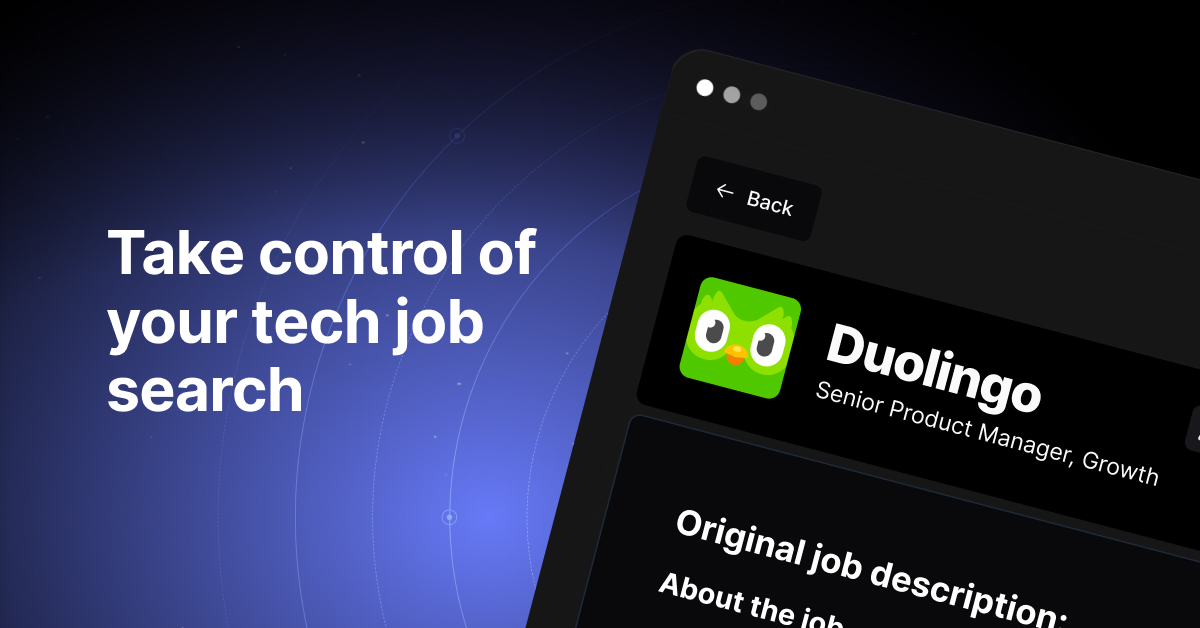