Downloading a file from spring controllers
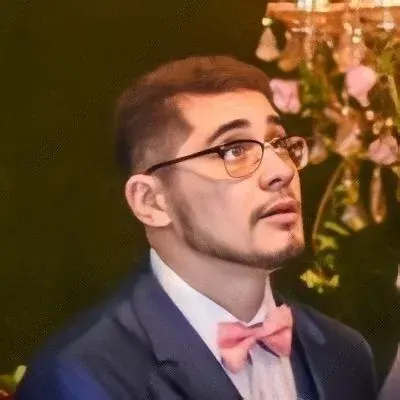
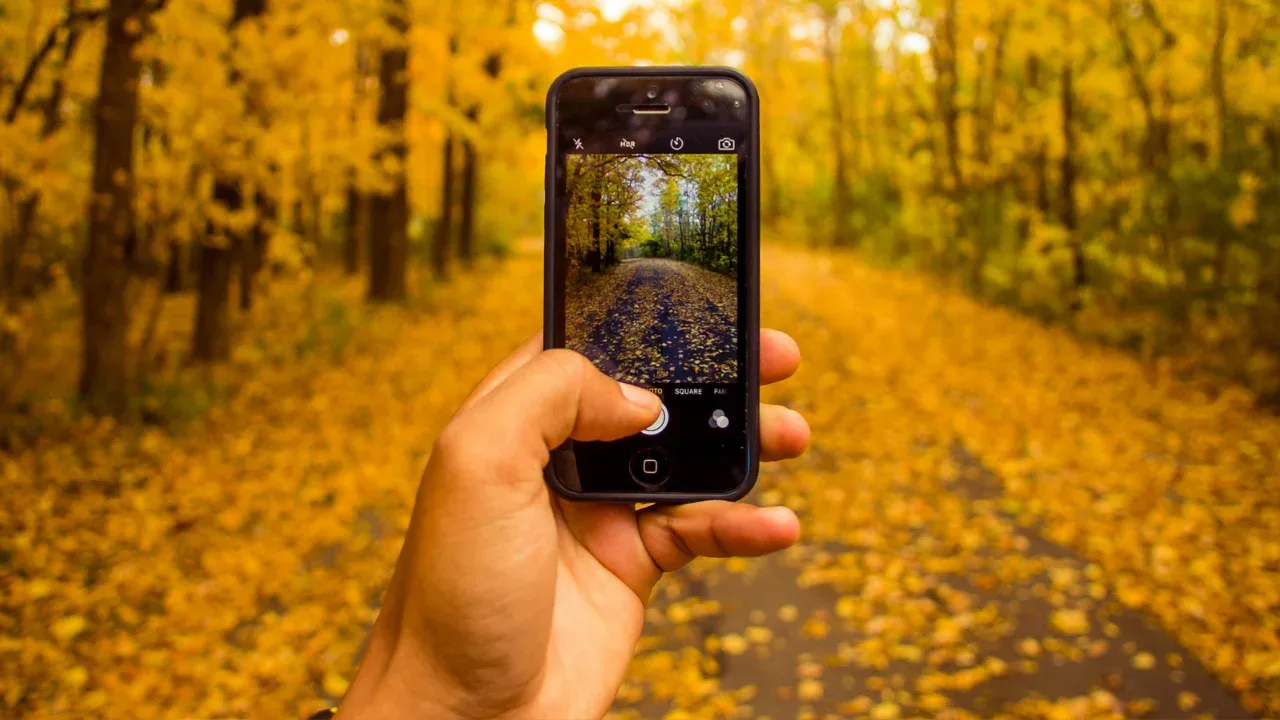
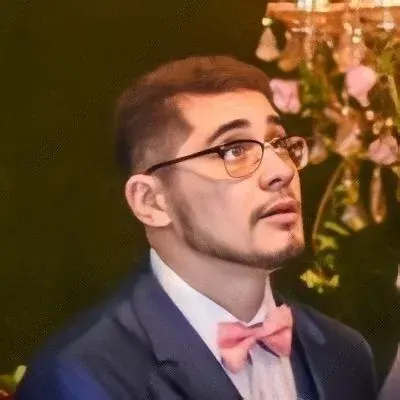
📥 A Guide to Downloading Files from Spring Controllers 🌸
Have you ever had the need to allow users to download files from your website? Perhaps a PDF generated dynamically within your code? 🧐 In this blog post, we'll explore the common issues related to downloading files from Spring Controllers and provide easy solutions to help you overcome them. 🚀
The Scenario: Generating a PDF with Freemarker and iText 📚
Our dear reader posed a question with a specific problem in mind. They were contemplating generating a PDF using Freemarker (a templating engine) combined with iText (a popular PDF generation framework). While this approach certainly works, our mission is to find an even better way! 💡
The Challenge: Enabling File Downloads via Spring Controllers 🤔
So, you've successfully generated your PDF. Congrats! But now comes the real challenge - how do you allow your users to download this file? Fear not, for Spring Controllers are here to save the day! 🎉
Spring Controllers provide a convenient way to handle HTTP requests and responses. To enable file downloads, we'll follow these simple steps:
First, you need to add the necessary dependencies to your project. For PDF generation, we recommend using libraries like iText, Apache PDFBox, or Flying Saucer. Add them to your project's pom.xml or build.gradle file.
Example (Maven):
<dependency> <groupId>com.itextpdf</groupId> <artifactId>itextpdf</artifactId> <version>latest-version</version> </dependency>
Next, you'll create a Spring Controller method that handles file downloads. Annotate this method with
@GetMapping
or@RequestMapping
(depending on your Spring version) and configure it to return aResponseEntity<Resource>
.Example:
@GetMapping("/download") public ResponseEntity<Resource> downloadFile() { // Logic to generate your PDF file goes here // Create a Resource object representing your PDF file Resource resource = new FileSystemResource("/path/to/your/file.pdf"); return ResponseEntity.ok() .header(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"file.pdf\"") .body(resource); }
In this example, we generate a PDF file and return it using a
FileSystemResource
. TheContent-Disposition
header is set to "attachment" to force the browser to download the file.Finally, you'll need to configure your web application to handle file downloads. This involves creating a
ResourceHttpRequestHandler
bean and registering it with Spring.Example (Java configuration):
@Configuration public class FileDownloadConfig implements WebMvcConfigurer { @Override public void addResourceHandlers(ResourceHandlerRegistry registry) { registry.addResourceHandler("/downloads/**") .addResourceLocations("file:/path/to/your/files/") .resourceChain(true) .addResolver(new PathResourceResolver()); } }
In this example, all files within the specified directory are made available for download under the
/downloads
URL path.
🚀 Supercharging Your File Download Capabilities!
Congratulations, you've successfully enabled file downloads from your Spring Controllers! 🎉 But why stop there? Here are some additional tips to supercharge your capabilities:
Dynamic File Generation: Instead of manually generating PDF files, consider using Thymeleaf or Mustache to dynamically generate them based on user input or data retrieved from a database.
Security Considerations: Ensure your application has appropriate access controls and validation mechanisms in place to prevent unauthorized file downloads.
Optimizing Downloads: Enhance user experience by providing progress indicators, handling large files efficiently, and compressing files to reduce download times.
📣 Engage with the Tech Community!
We hope this guide has shed some light on the process of downloading files from Spring Controllers. Share your success stories, tips, and tricks with our tech community! 🌐💬
Leave a comment below 👇 and let us know about your file download challenges or any other tech topics you'd like us to explore in future blog posts. Together, we can conquer the tech world! 🌍💪