Download a file with Android, and showing the progress in a ProgressDialog
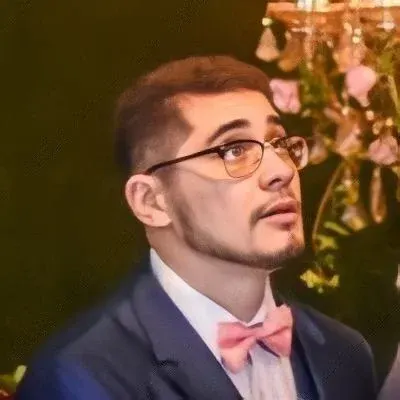
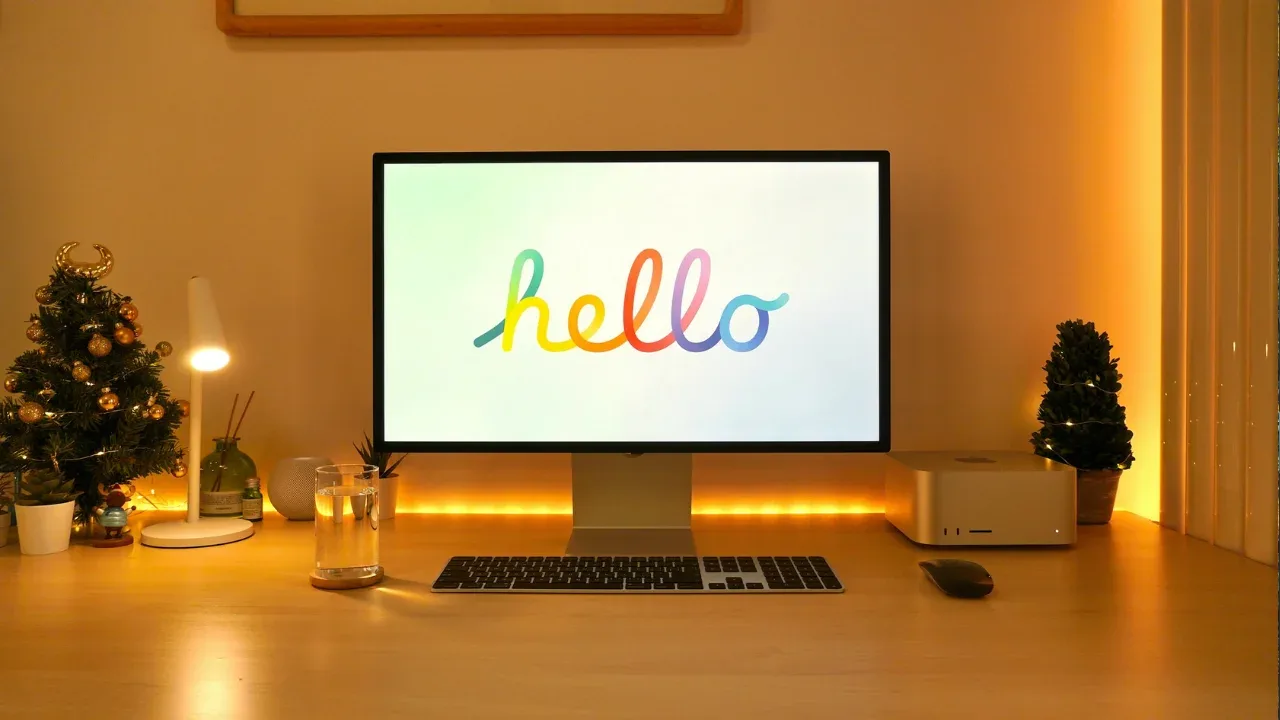
How to Download a File with Android and Show Progress in a ProgressDialog
š„šš±
Are you looking to create an Android application that allows users to download files while displaying the progress in a ProgressDialog? You're in the right place! In this guide, we'll cover everything you need to know to accomplish this task smoothly. š
The Problem: Downloading a File and Showing Progress
Many developers often struggle with two main challenges when it comes to downloading files and displaying the progress:
How to download a file in the first place.
How to show the current progress in a ProgressDialog.
Let's tackle these challenges step-by-step.
Solution #1: Downloading a File
To download a file in an Android application, we can make use of the built-in DownloadManager
class which simplifies the download process for us. Here's an example of how you can download a file with the DownloadManager
:
DownloadManager downloadManager = (DownloadManager) getSystemService(Context.DOWNLOAD_SERVICE);
DownloadManager.Request request = new DownloadManager.Request(Uri.parse("URL_OF_YOUR_FILE"));
downloadManager.enqueue(request);
In the above code snippet, replace "URL_OF_YOUR_FILE"
with the actual URL of the file you want to download. The DownloadManager
will handle the download process for you, making it simple and efficient.
Solution #2: Showing Progress in a ProgressDialog
To display the progress of the file download, we can use a ProgressDialog
to provide visual feedback to the user. Here's an example of how you can show the progress of the file download in a ProgressDialog
:
ProgressDialog progressDialog = new ProgressDialog(this);
progressDialog.setProgressStyle(ProgressDialog.STYLE_HORIZONTAL);
progressDialog.setMessage("Downloading file...");
progressDialog.show();
Make sure to customize the message and appearance of the ProgressDialog
to suit your application's needs. The key is to set the style to ProgressDialog.STYLE_HORIZONTAL
to enable horizontal progress display.
To update the progress of the download, you can use a BroadcastReceiver
to listen for the download progress updates and update the ProgressDialog
. Here's an example of how you can do this:
BroadcastReceiver receiver = new BroadcastReceiver() {
@Override
public void onReceive(Context context, Intent intent) {
int progress = intent.getIntExtra(DownloadManager.EXTRA_PROGRESS, 0);
progressDialog.setProgress(progress);
}
};
registerReceiver(receiver, new IntentFilter(DownloadManager.ACTION_DOWNLOAD_COMPLETE));
In the above code, we create a BroadcastReceiver
that listens for the progress updates sent by the DownloadManager
and updates the ProgressDialog
accordingly. Don't forget to unregister the receiver when it's no longer needed to avoid memory leaks.
Conclusion and Call-to-Action
Now that you know how to download a file and show the progress in a ProgressDialog, you can enhance your Android application by giving users the ability to download files seamlessly.
Try implementing these solutions in your own application and experiment with different customization options to create an engaging user experience. And don't forget to let us know how it went in the comments below! š¬
If you found this guide helpful, be sure to share it with your fellow Android developers who might also benefit from this knowledge. Happy coding! š
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
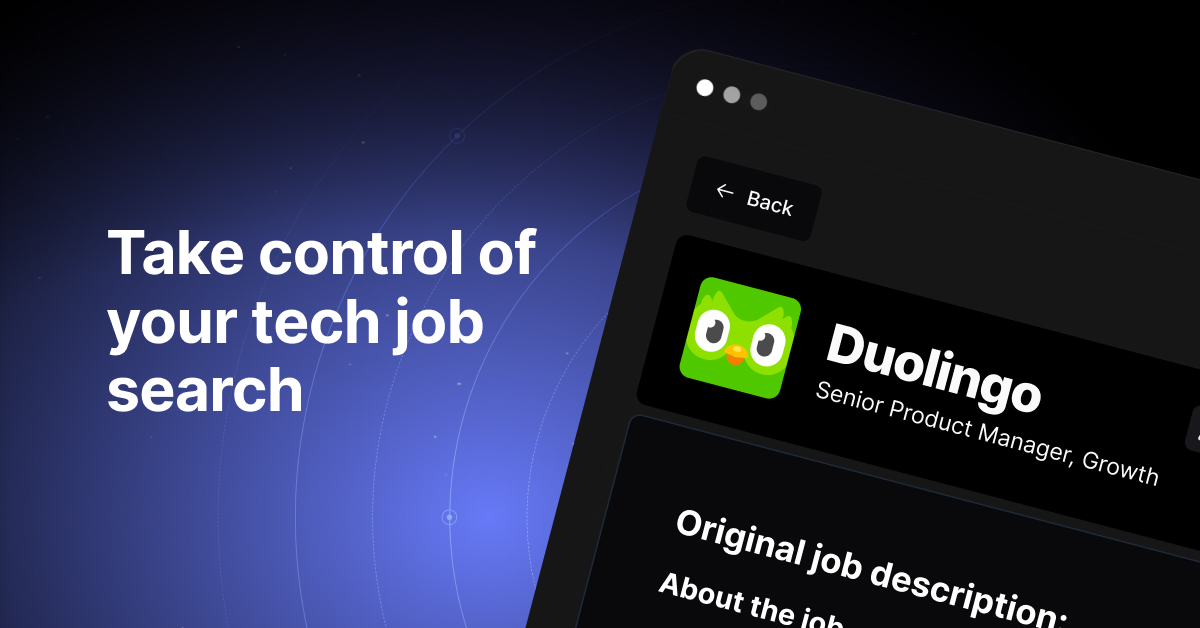