download a file from Spring boot rest service
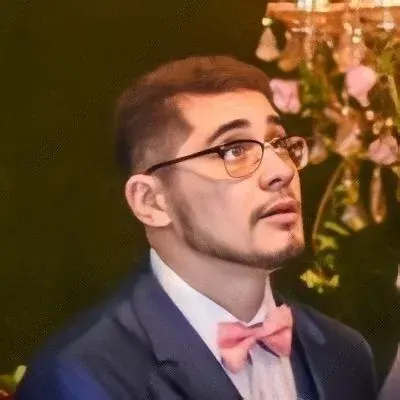
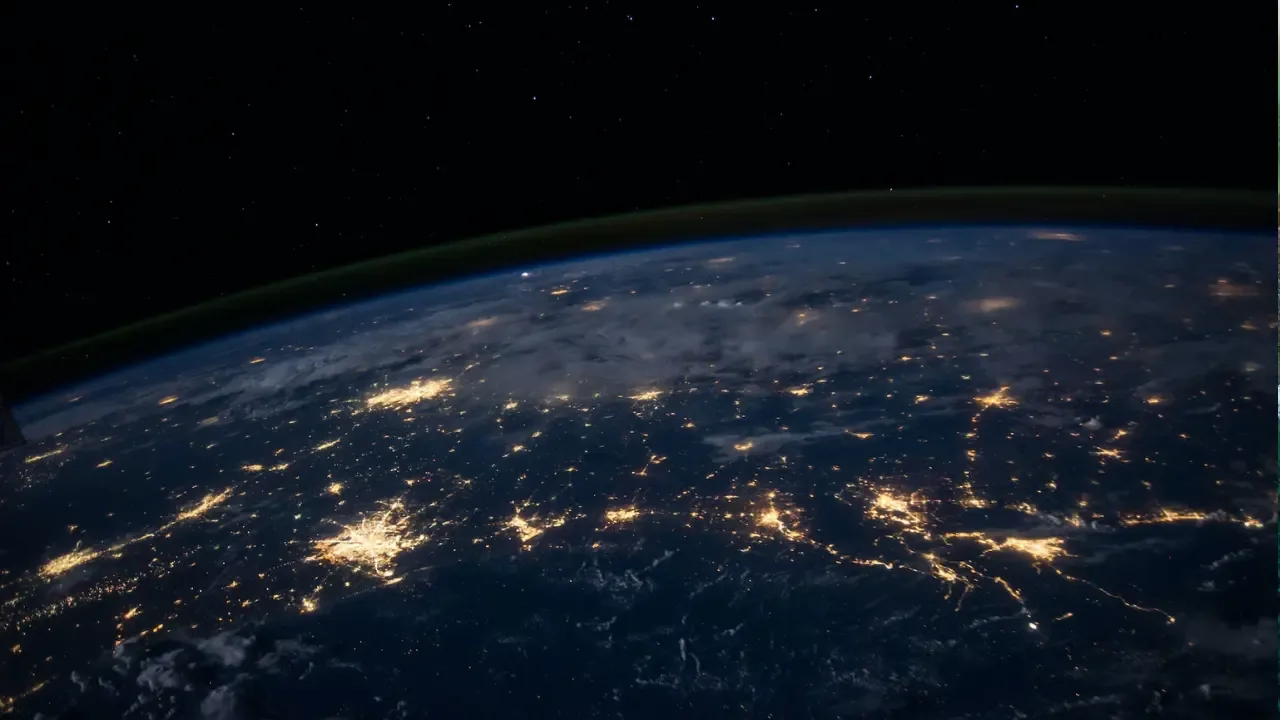
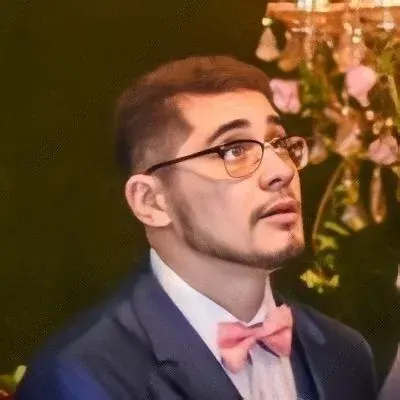
Downloading a File from a Spring Boot Rest Service: Common Issues and Solutions 📥
Are you trying to download a file from a Spring Boot REST service but facing issues? Don't worry, we've got you covered! In this blog post, we'll explore common problems that can occur during file download and provide easy solutions to ensure a successful download.
The Problem: Download Failed ❌
Let's take a look at the code snippet provided:
@RequestMapping(path="/downloadFile", method=RequestMethod.GET)
@Consumes(MediaType.APPLICATION_JSON_VALUE)
public ResponseEntity<InputStreamReader> downloadDocument(String acquisitionId, String fileType, Integer expressVfId) throws IOException {
File file2Upload = new File("C:\\Users\\admin\\Desktop\\bkp\\1.rtf");
HttpHeaders headers = new HttpHeaders();
headers.add("Cache-Control", "no-cache, no-store, must-revalidate");
headers.add("Pragma", "no-cache");
headers.add("Expires", "0");
InputStreamReader i = new InputStreamReader(new FileInputStream(file2Upload));
System.out.println("The length of the file is: " + file2Upload.length());
return ResponseEntity.ok()
.headers(headers)
.contentLength(file2Upload.length())
.contentType(MediaType.parseMediaType("application/octet-stream"))
.body(i);
}
The above code fetches the file from the specified path and returns it as a response to the REST service call. However, when trying to download the file from the browser, the download always fails. But why?
The Solution: Adding Content-Disposition Header 🚀
One common issue that can cause a download to fail is missing or incorrect headers in the HTTP response. In our case, the Content-Disposition
header, which specifies the filename to be used when saving the file, is missing. Without this header, the browser doesn't know how to handle the downloaded content.
To fix this issue, we need to add the Content-Disposition
header to the response. Modify your code as follows:
@RequestMapping(path="/downloadFile", method=RequestMethod.GET)
@Consumes(MediaType.APPLICATION_JSON_VALUE)
public ResponseEntity<InputStreamResource> downloadDocument(String acquisitionId, String fileType, Integer expressVfId) throws IOException {
File file2Upload = new File("C:\\Users\\admin\\Desktop\\bkp\\1.rtf");
HttpHeaders headers = new HttpHeaders();
headers.add("Cache-Control", "no-cache, no-store, must-revalidate");
headers.add("Pragma", "no-cache");
headers.add("Expires", "0");
// Add the Content-Disposition header
headers.add("Content-Disposition", "attachment; filename=\"1.rtf\"");
InputStreamResource inputStreamResource = new InputStreamResource(new FileInputStream(file2Upload));
System.out.println("The length of the file is: " + file2Upload.length());
return ResponseEntity.ok()
.headers(headers)
.contentLength(file2Upload.length())
.contentType(MediaType.parseMediaType("application/octet-stream"))
.body(inputStreamResource);
}
By adding the Content-Disposition
header with the value attachment; filename="1.rtf"
, we tell the browser to treat the response as an attachment and provide the filename as "1.rtf".
Test Your Download! ✅
Now that you've made the necessary changes to your code, it's time to test the file download. Try accessing the REST endpoint from your browser, and voilà! Your file should now download successfully.
If you're still facing any issues or have any other questions, feel free to leave a comment below or reach out to us. Happy downloading! 🎉
CALL TO ACTION:
Did this blog post help you solve your file download issue? Share your experience in the comments below and let us know if you have any other questions or topics you'd like us to cover in future blog posts. Don't forget to hit the share button and spread the knowledge!