Does Spring @Transactional attribute work on a private method?
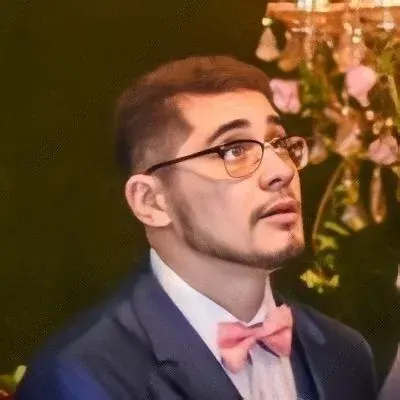
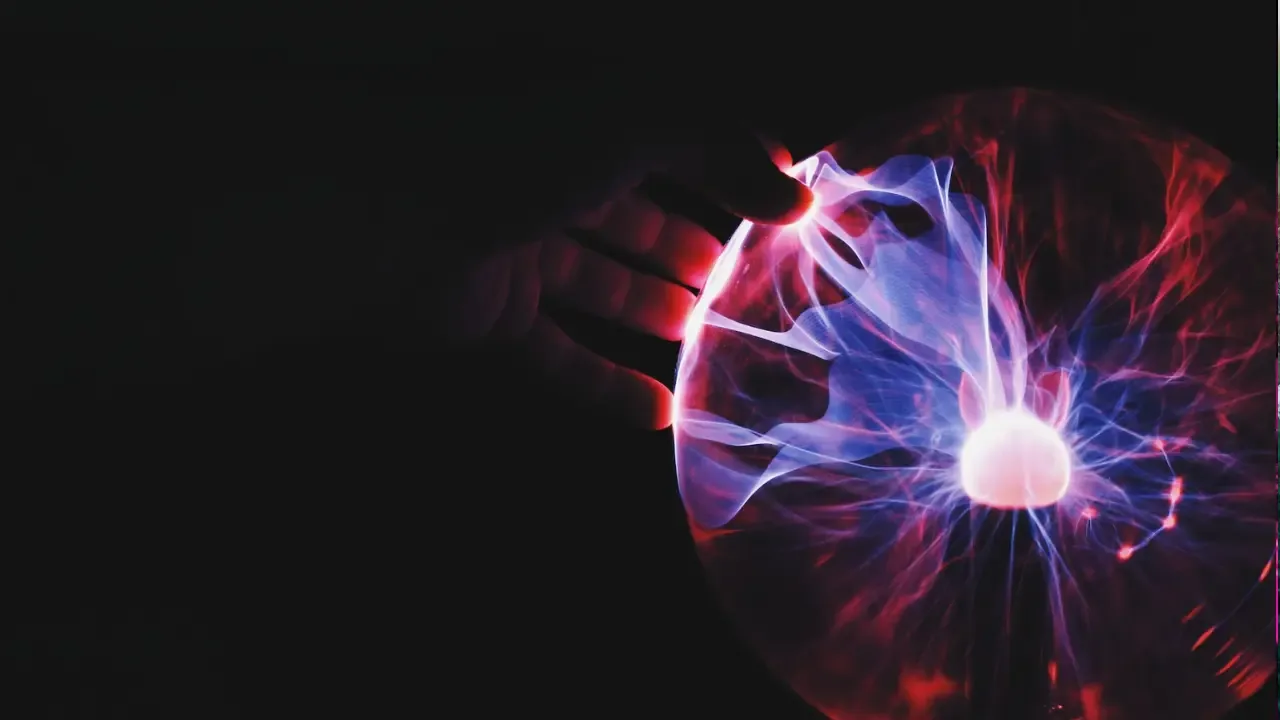
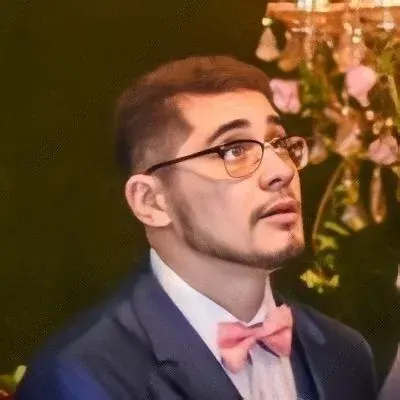
š» Tech Blog: Does Spring @Transactional attribute work on a private method?
š¢ Hey tech enthusiasts! Welcome back to our blog! Today, we're diving into a question that often confuses developers when using the Spring framework: Does the Spring @Transactional
attribute work on a private method?
š± For those who are new to Spring, the @Transactional
annotation allows us to define methods or classes as transactional, meaning they can participate in a database transaction. It ensures that the database operates in a consistent state, and it rolls back the transaction if any unexpected errors occur.
š¤ So, the big question is, can we use this powerful annotation on private methods? Let's find out!
š The answer is straightforward: Yes, the @Transactional
annotation works on private methods! However, it's essential to understand that the application context must be aware of the @Transactional
annotation.
š” In the provided code snippet, we have a class named Bean
, which has a public method doStuff()
and a private method doPrivateStuff()
. Inside doStuff()
, the private method doPrivateStuff()
is invoked.
š¼ This is how it looks:
public class Bean {
public void doStuff() {
doPrivateStuff();
}
@Transactional
private void doPrivateStuff() {
// Transactional logic here
}
}
...
Bean bean = (Bean) appContext.getBean("bean");
bean.doStuff();
š Notice that the @Transactional
annotation is present on the private method doPrivateStuff()
. Despite being private, the annotation will still work, opening a transaction when doPrivateStuff()
is invoked through the public method doStuff()
.
š® Surprised? Don't worry; many developers are! It's a common misconception that @Transactional
only works on public methods. Spring's transaction management mechanism ensures that private methods with the @Transactional
annotation are transactional, too.
š So, what's the catch? Why don't we always mark methods as private when using @Transactional
?
š The primary reason is readability. By convention, transactional methods should be public so that code maintainers and other developers can easily identify which methods are transactional in a class.
šļøāāļø Additionally, private transactional methods bypass the proxy mechanism Spring uses for managing transactions, which means we might not get the desired transactional behavior in certain scenarios. Therefore, it's best to stick to public methods for transactional purposes.
š That's it, folks! You now know that the @Transactional
annotation does work on private methods, but it's recommended to use it on public methods for better readability and consistent transaction behavior.
š We hope this blog post clarified any confusion you had regarding this topic. If you found this helpful, don't forget to share it with your fellow developers. Stay tuned for more exciting content!
š¢ Let us know in the comments below if you have any questions or share your thoughts on using transactional annotations with private methods. We'd love to hear from you!