Does Java have support for multiline strings?
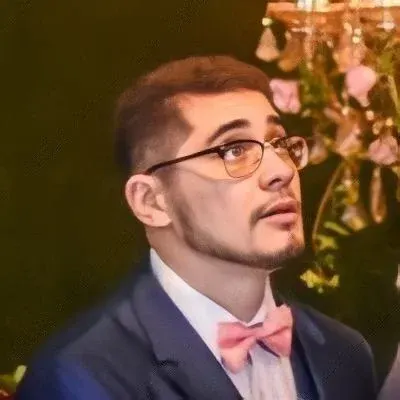

📝 Does Java have support for multiline strings?
Are you tired of having to concatenate strings line by line in Java just to create a multiline string? Coming from Perl, I sure do miss the convenience of the "here-document" feature. But fear not! In this blog post, I will address this common issue and provide you with easy solutions to create multiline strings in Java. 🎉
➕ String concatenation
One way to create a multiline string in Java is by using string concatenation. As the original post highlighted, this can be quite cumbersome and can clutter your code with quotes and plus signs on every line. But let's take a look at an example to see how it's done:
String multilineString = "Line 1" +
"Line 2" +
"Line 3";
In this example, we are concatenating three lines of text to create a multiline string. Although this works, it can quickly become difficult to read and maintain as the number of lines increases.
🧰 StringBuilder
An alternative to string concatenation is using the StringBuilder
class. Instead of manually concatenating strings, StringBuilder
provides a more efficient way to build strings dynamically. Check out this example:
StringBuilder multilineString = new StringBuilder();
multilineString.append("Line 1")
.append("Line 2")
.append("Line 3");
Now you might be wondering why using StringBuilder
is considered preferable to string concatenation. The main advantage is that StringBuilder
is more efficient when dealing with large multiline strings. It avoids unnecessary string object creations and provides better performance. Additionally, using StringBuilder
improves code readability and makes it easier to add or remove lines in the future.
🎯 Keep it maintainable and designed-focused
In the original post, the author expressed concerns about maintainability and design issues. It's important to address these concerns when working with multiline strings. Here are a few tips to keep your code maintainable and well-designed:
Consider using constants or resource files: If your multiline string is a static piece of text that won't change often, you can define it as a constant or store it in a resource file. This approach separates your string from your code, making it easier to update and localize your application.
Example using a constant:
private static final String MULTILINE_STRING = "Line 1\nLine 2\nLine 3";
Use proper indentation: To improve readability, make sure to indent each line of your multiline string consistently. This helps other developers understand the structure of your text and avoids confusion.
Example with proper indentation:
String multilineString = "Line 1\n" +
" Line 2\n" +
" Line 3";
Utilize formatting libraries: If your project involves significant amounts of formatted text, you can consider using libraries like Apache Commons Text's
WordUtils
or Google's GuavaStrings
to format your multiline strings.
✍️ Your turn!
Now that you have learned how to create multiline strings in Java and how to address maintainability and design issues, it's time to put your knowledge into practice. Experiment with the different techniques shared in this post and find the one that works best for your specific situation.
Have you encountered any other challenges or have additional insights? I'd love to hear your thoughts and experiences in the comments below. Let's continue the conversation and help each other out!
Happy coding! 👩💻👨💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
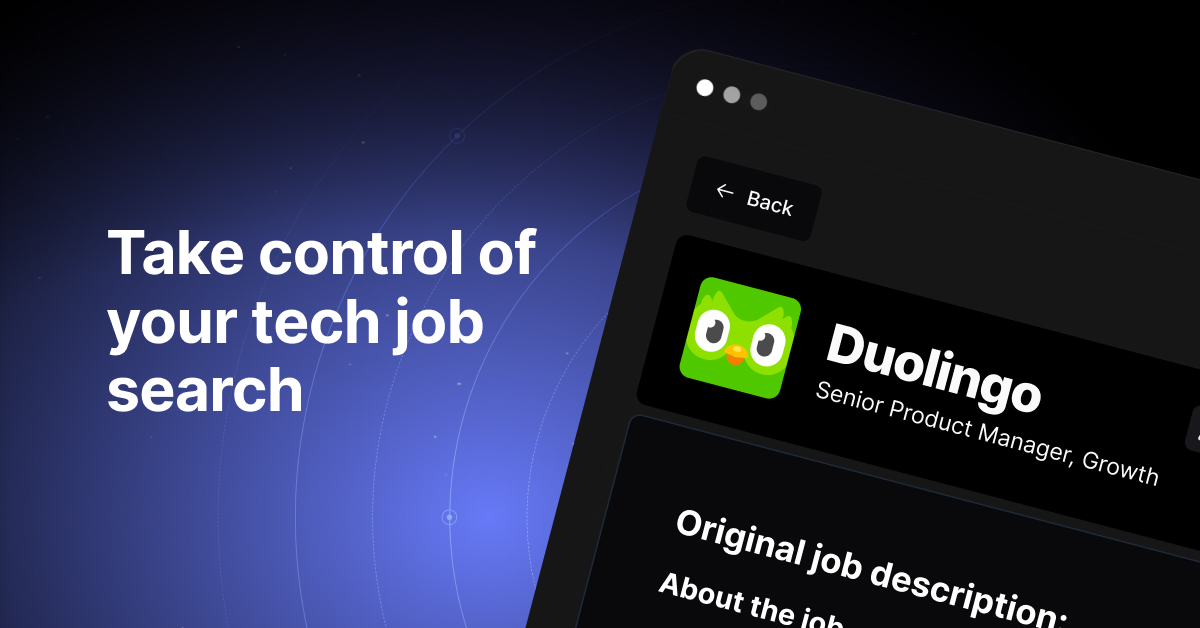