Difference between the annotations @GetMapping and @RequestMapping(method = RequestMethod.GET)
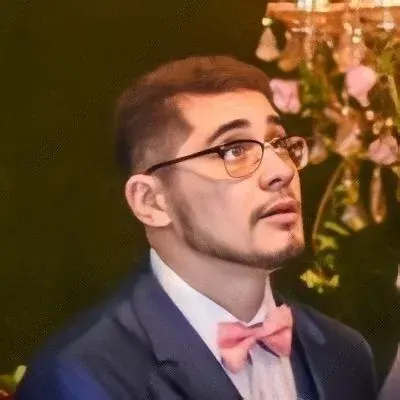
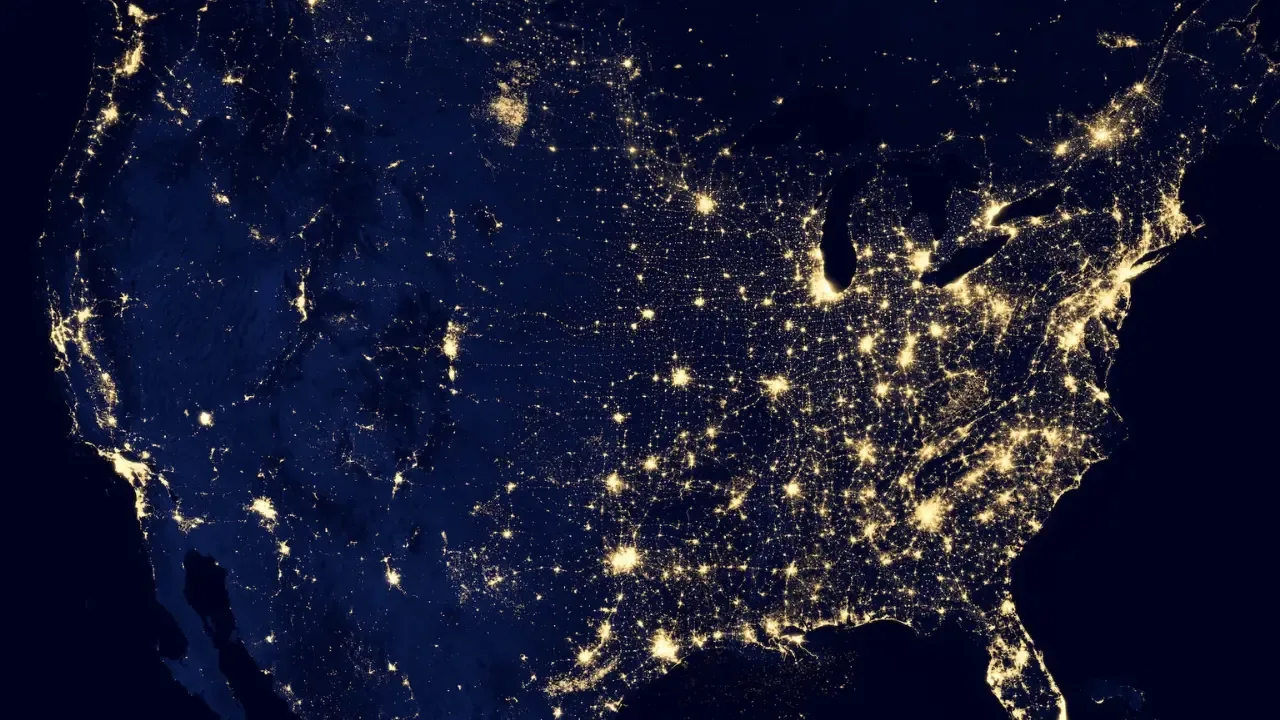
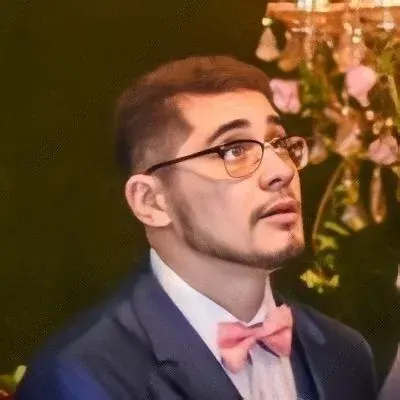
Understanding the Difference: @GetMapping vs @RequestMapping(method = RequestMethod.GET) ๐ก
If you're new to Spring Reactive or even a seasoned developer, you might have come across the annotations @GetMapping
and @RequestMapping(method = RequestMethod.GET)
and wondered what sets them apart. While both annotations are used for handling HTTP GET requests, they do have distinct differences. In this blog post, we'll explore those differences, address common issues, and provide easy solutions. Let's dive in! ๐
@GetMapping: The Shortcut ๐ฃ๏ธ
First up, let's talk about @GetMapping
. This annotation is a shortcut version of @RequestMapping
that specifically handles HTTP GET requests. The @GetMapping
annotation is available starting from Spring 4.3, and it simplifies the process of mapping a method to a GET request endpoint. Instead of explicitly specifying the HTTP method using @RequestMapping
, you can use @GetMapping
to achieve the same result more succinctly. It's all about reducing boilerplate code! ๐
Here's an example using @GetMapping
:
@GetMapping("/api/books")
public ResponseEntity<List<Book>> getAllBooks() {
// Logic for retrieving all books
}
In this example, the getAllBooks()
method will be invoked when a GET request is made to the /api/books
endpoint. The @GetMapping
annotation not only simplifies the code but also improves readability by explicitly indicating that the method is specifically designed for GET requests only. ๐
@RequestMapping(method = RequestMethod.GET): The Generic Approach ๐
On the other hand, @RequestMapping(method = RequestMethod.GET)
is the more generic approach for mapping a method to a GET request endpoint. This annotation allows you to handle various HTTP methods, not just GET. By explicitly specifying the HTTP method, you can accommodate different types of requests within the same method.
Here's an example using @RequestMapping(method = RequestMethod.GET)
:
@RequestMapping(value = "/api/books", method = RequestMethod.GET)
public ResponseEntity<List<Book>> getAllBooks() {
// Logic for retrieving all books
}
In this case, the getAllBooks()
method is again mapped to the /api/books
endpoint, but it can handle other HTTP methods if necessary.
Common Issues and Solutions โ๏ธ
Now that we've covered the basics of @GetMapping
and @RequestMapping(method = RequestMethod.GET)
, let's address a common issue faced by developers: migrating from @RequestMapping
to @GetMapping
.
If you're working on an older project that uses @RequestMapping
extensively, you might consider migrating to @GetMapping
to improve readability and make your code more concise. To do this, follow these simple steps:
Replace all instances of
@RequestMapping(method = RequestMethod.GET)
with@GetMapping
.Update any method signatures accordingly.
Remember, code refactoring should always be done with caution. Make sure to thoroughly test your application after making changes to avoid any unintended consequences. ๐งช
Your Turn, Engaging Readers! ๐ฃ
Now that you understand the difference between @GetMapping
and @RequestMapping(method = RequestMethod.GET)
, it's time to put your newfound knowledge to the test! Reflect on your own Spring projects or share your experiences with these annotations in the comments below. We'd love to hear your thoughts! ๐
And don't forget, if you enjoyed this blog post, share it with your fellow developers who might find it useful. Happy coding! ๐ฉโ๐ป๐จโ๐ป
Note: This guide assumes you're using Spring MVC or Spring WebFlux.