Difference between <? super T> and <? extends T> in Java
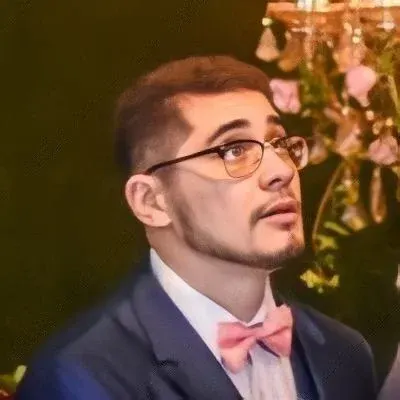

The Ultimate Guide to Understanding the Difference between List<? super T>
and List<? extends T>
in Java 🚀
So, you've come across the confusing issue of using List<? super T>
and List<? extends T>
in Java. Don't worry, you're not alone! Many developers struggle with this concept when dealing with generic types. But fear not, because we've got you covered with this comprehensive guide that will help you understand the difference and provide easy solutions to common problems. Let's dive in! 💪🏼
Understanding the Basics
Before we delve into the differences, it's important to grasp the fundamental concepts behind the wildcard types used in Java generics. The wildcard symbol (?
) represents an unknown type, which allows for more flexibility when dealing with generic types.
Now, let's look at the definitions of List<? super T>
and List<? extends T>
:
List<? super T>
: This represents a list that can contain elements of any type that is a supertype ofT
. In other words,T
can be assigned to any of its superclasses or theObject
class.List<? extends T>
: This represents a list that can contain elements of any subtype ofT
. Here,T
can be assigned to any of its subclasses or theT
class itself.
The Problem: Adding vs. Accessing Elements
One common confusion developers encounter is related to adding elements to and accessing elements from the list. Let's take a look at these two wildcard types in action:
List<? super T> superList = new ArrayList<>();
List<? extends T> extendsList = new ArrayList<>();
In the superList
, you can add elements that are of type T
or any of its subtypes. For example, assuming T
is a Fruit
class, you can add Apple
, Banana
, or any other subtype of Fruit
. This is because the unknown type (?
) can be a supertype of T
, so you have the flexibility to add elements of different subtypes to the list.
superList.add(new Apple());
superList.add(new Banana());
On the other hand, in the extendsList
, you can only access elements from the list, but you cannot add any new elements. This restriction is a trade-off for providing more specificity regarding the type of elements that can be accessed.
T element = extendsList.get(0); // Accessing elements is allowed
extendsList.add(new Apple()); // This will result in a compilation error!
Easy Solutions to Common Problems
If you need to add elements to the list 📝
If you find yourself needing to add elements to the list, you should use List<? super T>
. This wildcard type allows you to add any subtype of T
.
If you only need to access elements from the list 👀
If you only need to access elements from the list and don't require the ability to add new elements, you can use List<? extends T>
. This will provide type safety by restricting the list to contain only elements of a specific subtype of T
.
Let's Sum It Up 📚
In a nutshell, the key difference between List<? super T>
and List<? extends T>
boils down to their capabilities regarding adding and accessing elements.
Use
List<? super T>
if you want to add elements of any subtype ofT
.Use
List<? extends T>
if you only need to access elements, without the ability to add new ones.
Understanding this distinction will prevent those pesky compilation errors and help you code more effectively.
Engage and Share Your Thoughts! 💬
We hope this guide has shed some light on the difference between List<? super T>
and List<? extends T>
. Now, it's your turn to join the conversation! Have you ever encountered issues with wildcard types in Java generics? How did you solve them? Share your experience and tips in the comments section below. Let's learn and grow together! 🌱
Remember to share this post with your developer friends who might be struggling with this topic. Spread the knowledge and make their lives a little easier! 🚀
Happy coding! 👩💻👨💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
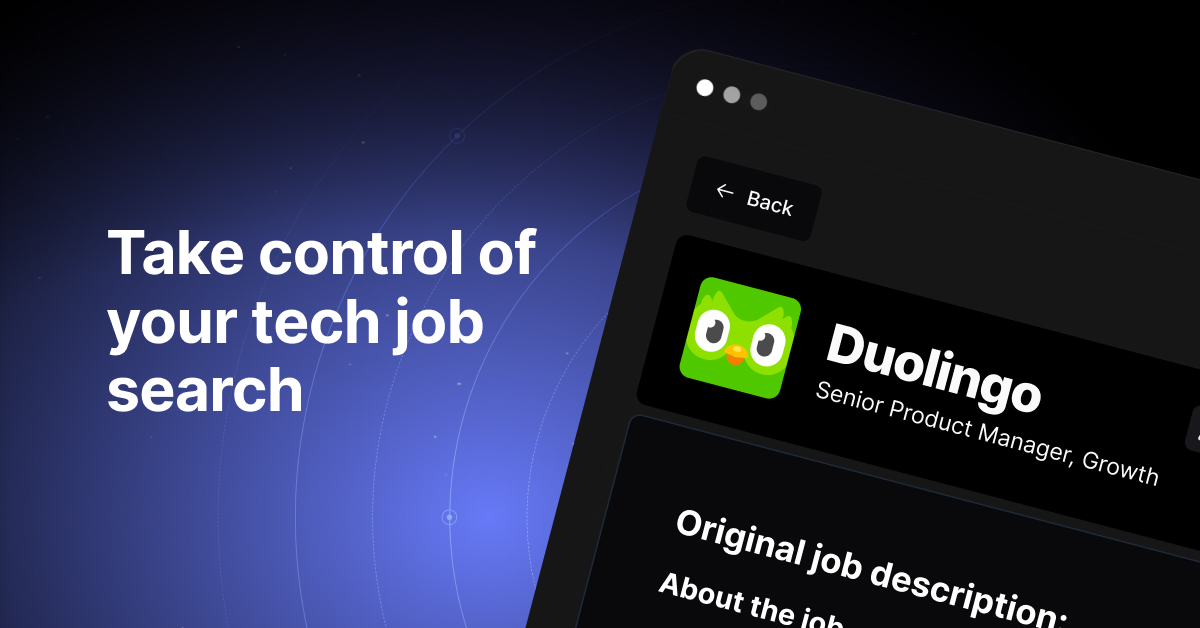