Difference between Role and GrantedAuthority in Spring Security
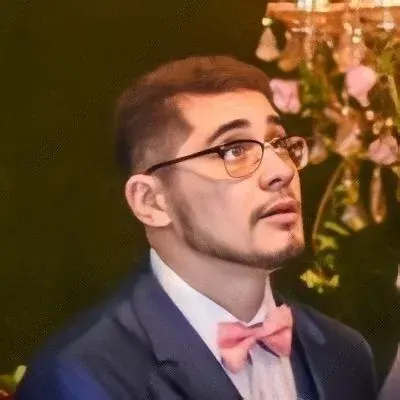
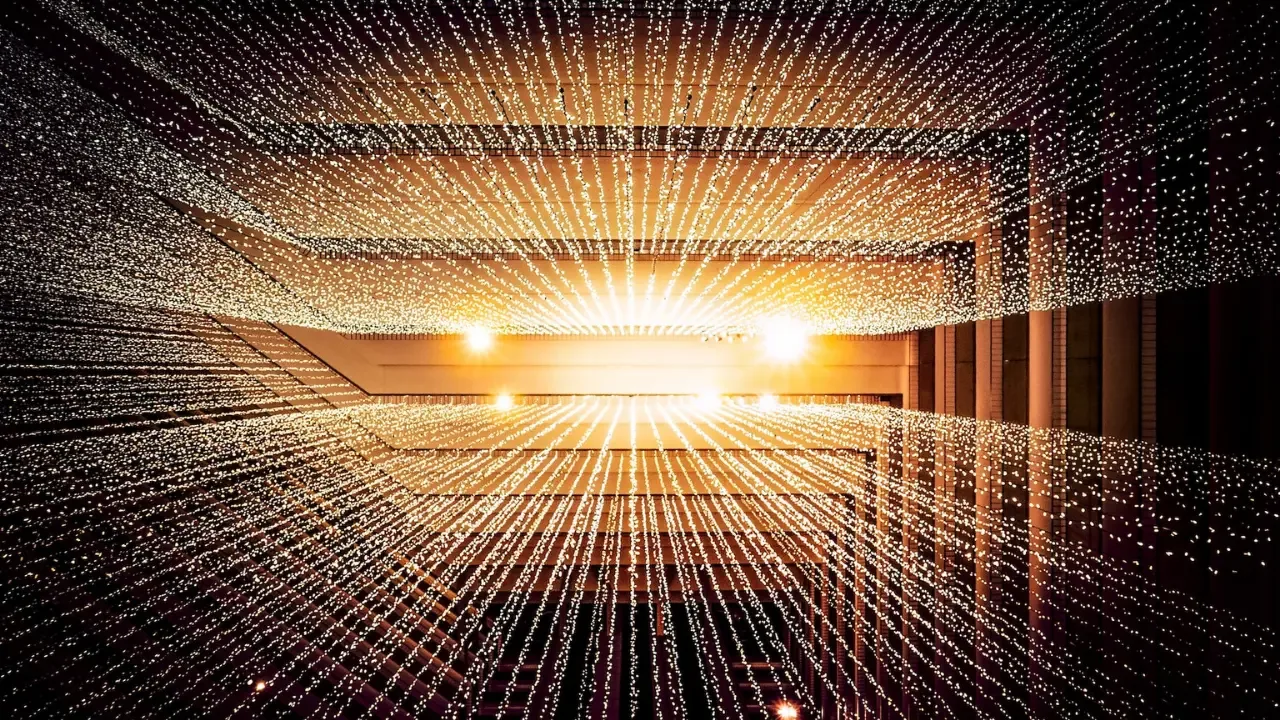
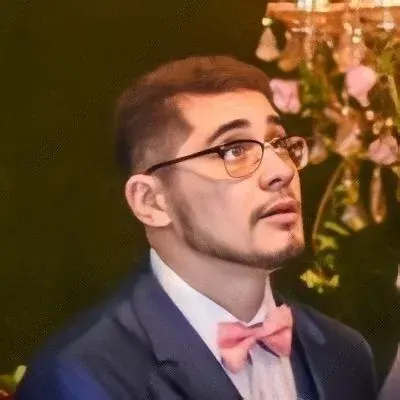
🌟 Understanding the Difference between Role and GrantedAuthority in Spring Security 🌟
Are you feeling puzzled by the concepts of "Role" and "GrantedAuthority" in Spring Security? Don't worry, you're not alone! Many developers find it confusing and treat these two terms interchangeably. But fear not, I'm here to shed some light on this topic and provide you with easy solutions!
🔑 Role and GrantedAuthority: Conceptual Explanation
In Spring Security, a "Role" represents a higher-level authorization or access level assigned to a user. It defines a set of permissions or authorities associated with it. For example, you might have an "admin" role with the permissions to create sub-users and delete accounts.
On the other hand, a "GrantedAuthority" is an interface used to define individual authorities or permissions granted to a user. These authorities are linked to a specific role, representing a fine-grained level of access control. For example, a "createSubUsers" authority or a "deleteAccounts" authority can be associated with the "admin" role.
💡 Storing Role and Authorities Separately
Now, you might wonder how to store the role of a user separately from the authorities for that role. Fortunately, Spring Security provides flexibility in this regard. You can create your own implementation of the User
class, implementing the UserDetails
interface. This allows you to store the role and authorities in separate attributes.
For example, let's say we have a custom User
class with attributes like username
, password
, enabled
, accountNonExpired
, credentialsNonExpired
, accountNonLocked
, and authorities
. The authorities
attribute can be a collection of GrantedAuthority
objects associated with the user.
Here's an example of how you can create a custom User
class:
public class User implements UserDetails {
private String username;
private String password;
private boolean enabled;
private boolean accountNonExpired;
private boolean credentialsNonExpired;
private boolean accountNonLocked;
private Collection<? extends GrantedAuthority> authorities;
// Constructor and other methods here...
}
🔄 Differentiating Role and Authorities
To differentiate between roles and authorities in your code, you need to understand how they are used. Roles are typically used for higher-level access control, such as in @PreAuthorize("hasRole('ROLE_ADMIN')")
annotations or configuration. On the other hand, authorities are used for more granular access control, like @PreAuthorize("hasAuthority('createSubUsers')")
or @PreAuthorize("hasAuthority('deleteAccounts')")
.
❗️ Compelling Call-to-Action
Now that you have a clearer understanding of the difference between Role and GrantedAuthority in Spring Security, it's time to put your knowledge into practice! Try implementing role-based and authority-based access control in your application. Experiment with different scenarios to see how they work in real-world situations.
Remember, understanding these concepts is crucial for building robust and secure applications. So dive into the Spring Security documentation, explore more examples, and don't hesitate to reach out to the friendly developer community for any assistance.
Happy coding! 🚀