Difference between @Mock, @MockBean and Mockito.mock()
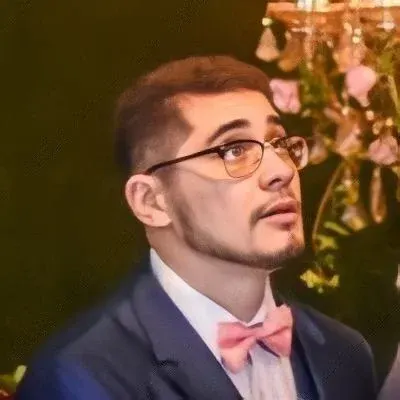
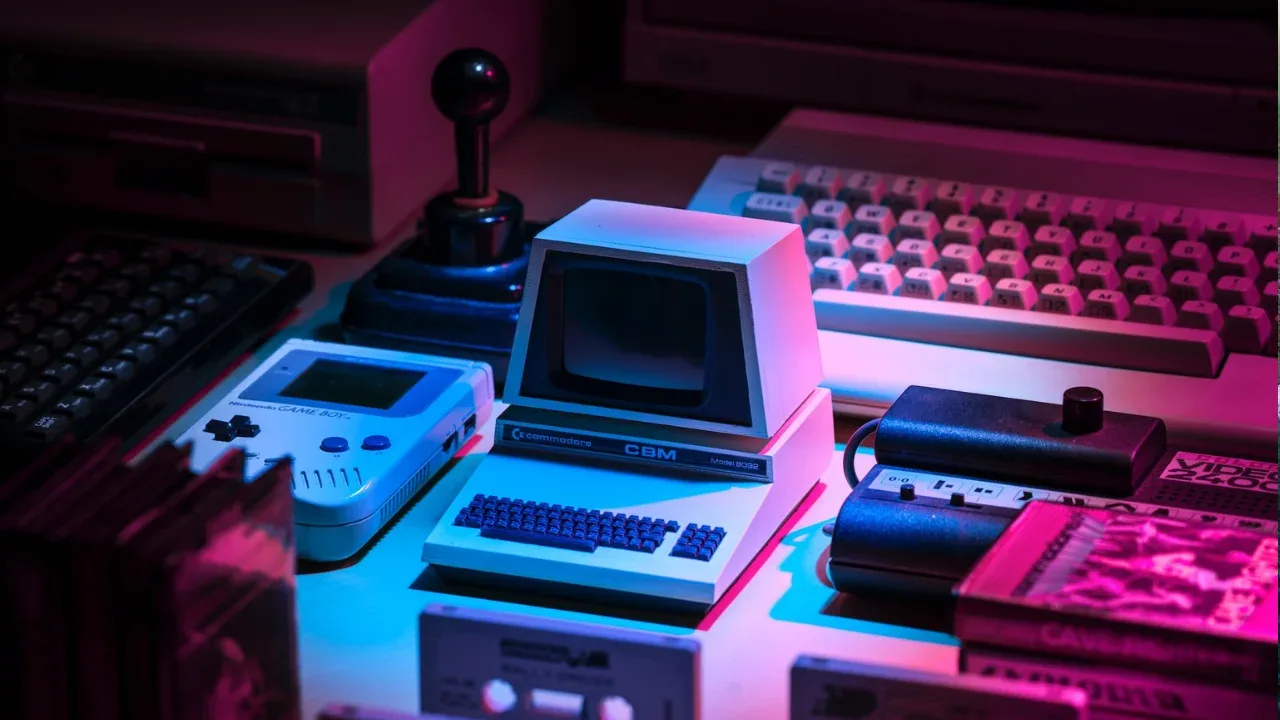
Three Ways to Mock Dependencies: @Mock, @MockBean, and Mockito.mock()
<p>When writing tests, mocking dependencies is a common practice. Mocking allows us to isolate components and test them independently, without relying on real dependencies. In this blog post, we'll explore the difference between three popular approaches for mocking: @Mock, @MockBean, and Mockito.mock(). So let's dive in! 💡</p>
1. @MockBean
<p>The <code>@MockBean</code> annotation is part of the Spring Boot framework and is used in integration tests. It creates a mock instance of the specified class and replaces any existing bean of the same type in the application context. Here's an example:</p>
@MockBean
MyService myService;
<p>By using <code>@MockBean</code>, we can easily mock a dependency and inject it into the test context. This approach is particularly useful when writing integration tests that involve Spring components, as it leverages the power of the Spring framework.</p>
2. @Mock
<p>The <code>@Mock</code> annotation is provided by the Mockito library, which is widely used for writing unit tests. It creates a mock instance of the specified class without replacing any beans in the Spring context. Here's how you can use it:</p>
@Mock
MyService myService;
<p>By using <code>@Mock</code>, we can create a mock object for the given class. It's important to note that unlike <code>@MockBean</code>, this annotation is not aware of the Spring context, so it's typically used in unit tests where mocking dependencies is the primary concern.</p>
3. Mockito.mock()
<p>The third approach is to use the <code>Mockito.mock()</code> method directly. This method is also part of the Mockito library and creates a mock instance of the specified class. Here's an example:</p>
MyService myService = Mockito.mock(MyService.class);
<p>Similar to <code>@Mock</code>, <code>Mockito.mock()</code> is commonly used in unit tests. It allows you to manually create a mock object without relying on annotations.</p>
Which Approach Should You Choose?
<p>Now that we've covered the three approaches, you might wonder which one to use. The choice depends on your testing scenario and the framework you are using. If you are writing integration tests with Spring Boot, <code>@MockBean</code> is the way to go. For unit tests using Mockito, <code>@Mock</code> and <code>Mockito.mock()</code> are both valid options.</p>
<p>Remember, the key difference between <code>@MockBean</code> and the other two approaches is that <code>@MockBean</code> replaces beans in the Spring context, while <code>@Mock</code> and <code>Mockito.mock()</code> do not.</p>
Wrapping Up
<p>In this blog post, we explored the difference between three mocking approaches: <code>@MockBean</code>, <code>@Mock</code>, and <code>Mockito.mock()</code>. Understanding these distinctions will help you choose the right mocking technique for your tests.</p>
<p>Whether you are working with Spring Boot or using Mockito directly, these mocking tools are essential for testing. So go ahead and give them a try in your next project! Remember, testing is crucial for writing robust and reliable code. Happy mocking! 🚀</p>
Your Turn!
<p>Now, it's your turn to share your thoughts! Which mocking technique do you prefer, and why? Have you encountered any challenges while using these approaches? Let's start a conversation in the comments below! 🗣️</p>
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
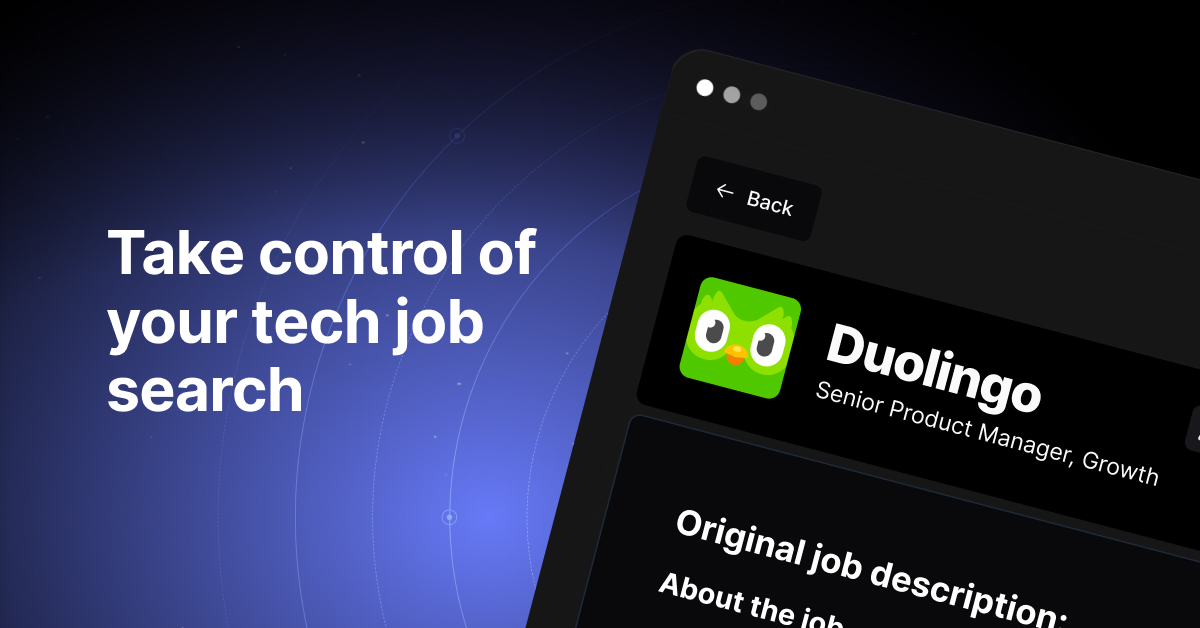