Difference between HashMap, LinkedHashMap and TreeMap
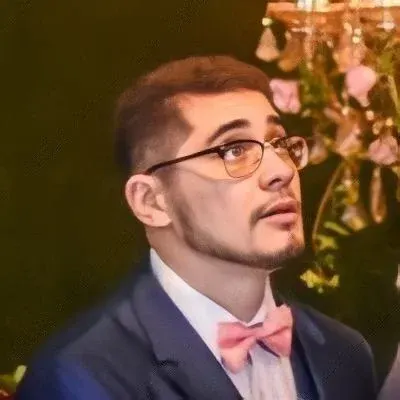

Demystifying HashMap, LinkedHashMap, and TreeMap in Java
Hey there, tech enthusiasts! 👋 Are you confused about the differences between HashMap
, LinkedHashMap
, and TreeMap
in Java? 🤔 Well, worry no more! In this blog post, we'll break down these three data structures and help you understand their unique features and use cases. By the end, you'll be equipped with the knowledge to choose the right one for your Java projects. Let's dive right in! 💡
Understanding the Basics
First things first, let's clarify what these data structures are:
HashMap
: It is an unordered collection that stores elements in key-value pairs. Efficiency is a key aspect here, with constant time complexity for basic operations likeput
,get
, andremove
. However, it does not guarantee any particular order of elements.LinkedHashMap
: Similar toHashMap
, it also stores elements in key-value pairs. However, unlikeHashMap
, it maintains the insertion order of the elements. This additional bookkeeping leads to slightly more overhead, but it provides predictable iteration order as a benefit.TreeMap
: As the name suggests, it is based on a Red-Black tree structure. This means that elements in aTreeMap
are stored in a sorted order defined by the natural ordering of keys or a customComparator
. It offers efficient searching, insertion, and deletion operations, but at a cost of increased time complexity compared to the previous two.
Common Confusion: Output Similarities
Now, let's address the common confusion raised in our question. It is true that when you print the keySet
and values
of these data structures, they may appear to have similar outputs. However, the differences lie beneath the surface. Let's see an example to better understand:
// Creating a HashMap
Map<String, String> hashMap = new HashMap<>();
hashMap.put("map", "HashMap");
hashMap.put("schildt", "java2");
hashMap.put("mathew", "Hyden");
hashMap.put("schildt", "java2s");
// Creating a TreeMap
SortedMap<String, String> treeMap = new TreeMap<>();
treeMap.put("map", "TreeMap");
treeMap.put("schildt", "java2");
treeMap.put("mathew", "Hyden");
treeMap.put("schildt", "java2s");
// Creating a LinkedHashMap
Map<String, String> linkedHashMap = new LinkedHashMap<>();
linkedHashMap.put("map", "LinkedHashMap");
linkedHashMap.put("schildt", "java2");
linkedHashMap.put("mathew", "Hyden");
linkedHashMap.put("schildt", "java2s");
System.out.println(hashMap.keySet());
System.out.println(treeMap.keySet());
System.out.println(linkedHashMap.keySet());
If you run this code, you'll notice that the outputs of hashMap.keySet()
, treeMap.keySet()
, and linkedHashMap.keySet()
might look the same. However, the underlying data structures dictate the iteration order.
Solution: Choosing the Right Data Structure
So, when should you use each of these data structures? Let's break it down for you:
Use
HashMap
when you need a fast and efficient key-value store, and order is not a concern.Use
LinkedHashMap
when you want to maintain the insertion order of elements while still having decent performance. It's especially useful in scenarios where you need a cache-like structure.Use
TreeMap
when you require a sorted order of elements based on keys or a custom sorting logic. It's perfect for situations where you need a range of keys or efficient searching operations.
Take Action: Engage and Share!
Congratulations, my friend! 🎉 You are now equipped with knowledge about HashMap
, LinkedHashMap
, and TreeMap
in Java. But don't let it stop here! Share this blog post with your fellow developers who might find it helpful. Let's spread the knowledge and make the tech community stronger. 💪
If you found this information useful or have any further questions, feel free to drop a comment below. Let's engage in a lively conversation and learn from each other!
Happy coding! 😄🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
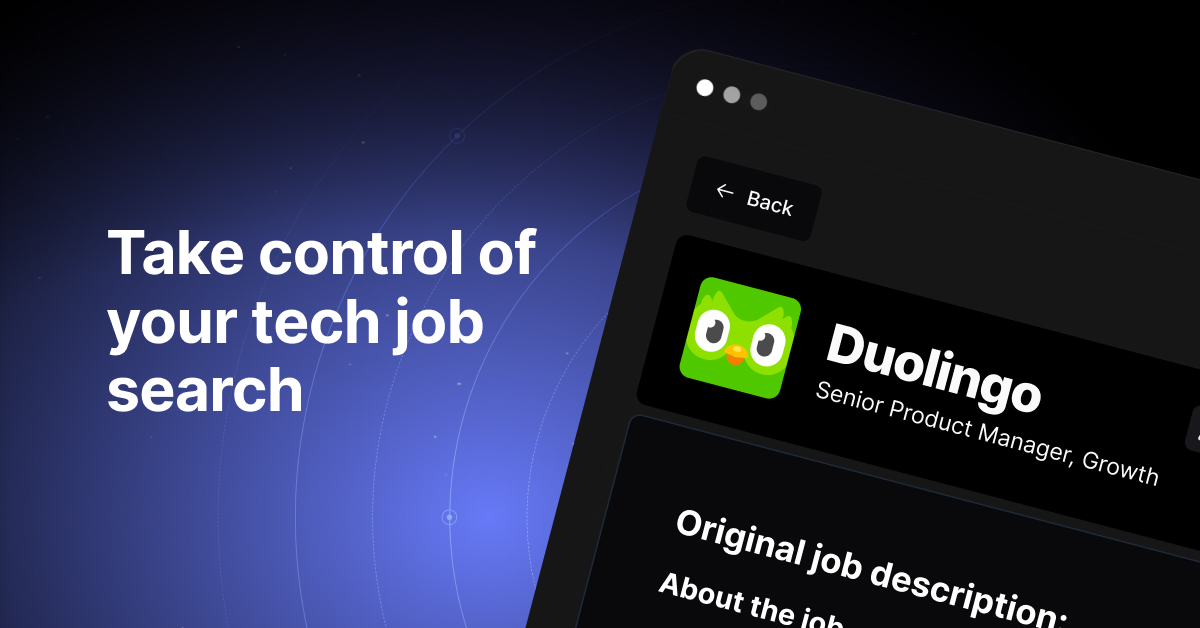