Create ArrayList from array
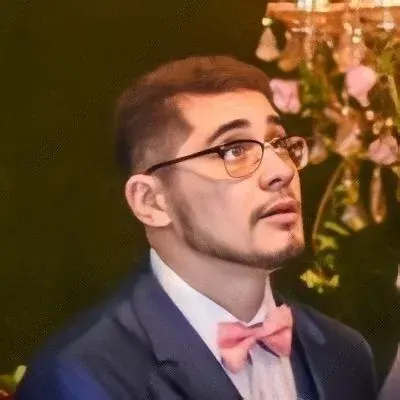
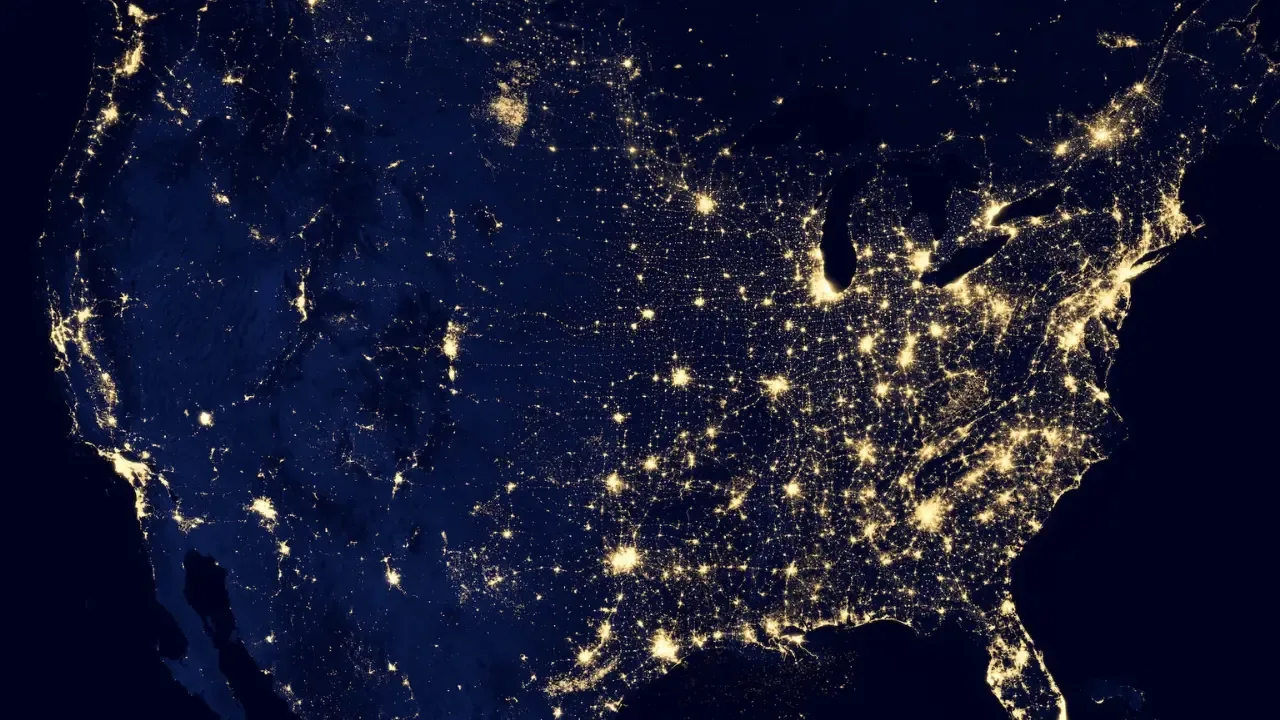
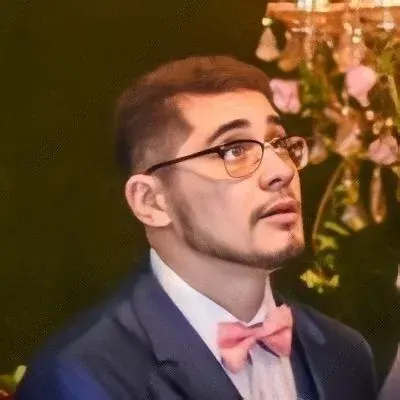
📝 Converting an Array into an ArrayList in Java
So you have an array of type Element[]
and you want to convert it into an ArrayList<Element>
. No worries, mate! I got your back! 💪
The Problem
Let's take a look at the example array we have:
Element[] array = {new Element(1), new Element(2), new Element(3)};
And here's what we want to achieve:
ArrayList<Element> arrayList = ???;
Basically, we want to populate the ArrayList
with the elements from our existing array. But how do we do that? 🤔
The Solution
Fear not, my friend! Java has got a handy-dandy Arrays
class with a method called asList()
that can help us out here. Let's use it:
ArrayList<Element> arrayList = new ArrayList<>(Arrays.asList(array));
Voilà! 😎 By passing the array
into Arrays.asList()
, we create a List<Element>
object, and then by passing that into the ArrayList
constructor, we convert it into an ArrayList<Element>
.
A Closer Look
Now, let's break down the solution and understand it better.
We start by calling
Arrays.asList(array)
. This method takes an array as a parameter and returns a fixed-size list that wraps the original array. However, keep in mind that this list is not a regularArrayList
yet; it just implements theList
interface.By passing the
list
returned byArrays.asList()
into theArrayList
constructor, we create a properArrayList
object that we can manipulate as we wish.
The Complication
Wait! Just a small caveat I have to mention: when using this method, the resulting ArrayList
is still backed by the original array. That means any changes made to the ArrayList
will also affect the original array and vice versa. So, if you modify any element in the ArrayList
, the corresponding element in the original array will also be updated. Keep this in mind when dealing with mutable objects! 👀
The Call to Action
I hope this mini-guide has helped you understand how to convert an array into an ArrayList
with ease. Now, it's time for you to put it to practice! ✨
Why not try converting an array of your own and explore the possibilities that come with using an ArrayList
? Share your experience in the comments below, and let's keep the conversation going! 💬🚀