Could not autowire field:RestTemplate in Spring boot application
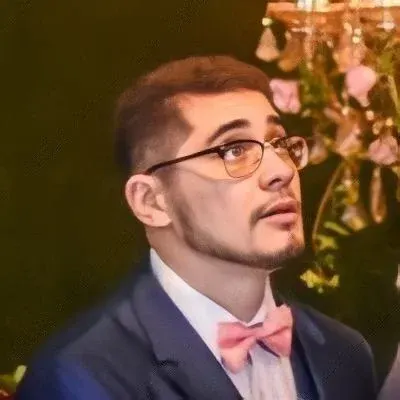
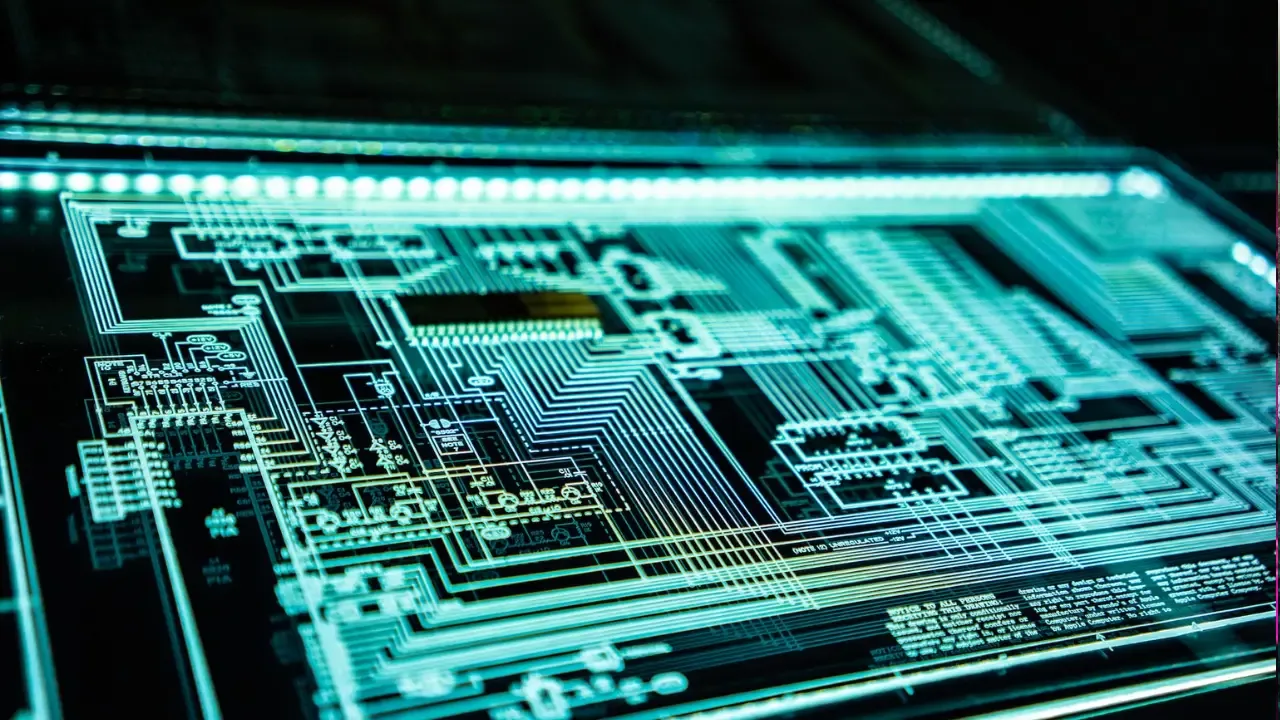
How to Fix "Could not autowire field: RestTemplate" Error in Spring Boot Application 😮🛠️
If you are encountering the "Could not autowire field: RestTemplate" error while running your Spring Boot application, don't worry! This blog post will guide you through common issues and provide easy solutions. 🚀
Understanding the Error 🤔❗
The error message you see indicates that Spring is unable to autowire the RestTemplate
field in your TestController
class. Let's take a closer look at the error message:
org.springframework.beans.factory.BeanCreationException:
Error creating bean with name 'testController':
Injection of autowired dependencies failed;
nested exception is
org.springframework.beans.factory.BeanCreationException:
Could not autowire field:
private org.springframework.web.client.RestTemplate
com.micro.test.controller.TestController.restTemplate;
nested exception is
org.springframework.beans.factory.NoSuchBeanDefinitionException:
No qualifying bean of type
[org.springframework.web.client.RestTemplate]
found for dependency: expected at least 1 bean which qualifies
as autowire candidate for this dependency.
Dependency annotations: {@org.springframework.beans.factory.annotation.Autowired(required=true)}
From this error message, we can deduce that Spring is unable to find a qualifying RestTemplate
bean for autowiring, even though you have declared it in your TestController
class. This error typically occurs when there is an issue with dependency management.
Solution 🛠️
To resolve this error, follow these steps:
Step 1: Check Maven Dependency
Make sure you have the necessary Maven dependency for RestTemplate
in your pom.xml
file. Here is an example of the required dependency:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Add RestTemplate dependency -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
Step 2: Restart Your Application
After adding the RestTemplate
dependency, restart your Spring Boot application. This will allow Spring to scan and load the necessary beans.
Step 3: Verify Spring Boot Version
Ensure that you are using a compatible Spring Boot version. The RestTemplate
class was deprecated as of Spring Boot 2.0, and it is recommended to use WebClient
instead. If you are using an older version of Spring Boot, you should be able to use RestTemplate
without any issues.
Step 4: Check Component Scanning
Verify that the package containing your RestTemplate
bean is being scanned by Spring. By default, Spring Boot only scans the package where your main application class is located. If your RestTemplate
bean is defined in a different package, make sure to add the @ComponentScan
annotation to your main application class, specifying the package to be scanned.
@SpringBootApplication
@ComponentScan("com.micro.test.controller")
public class TestMicroServiceApplication {
// ...
}
Step 5: Use Constructor Injection
Instead of using field injection (annotating the field with @Autowired
), try using constructor injection. Create a constructor in your TestController
class that takes a RestTemplate
parameter and annotate it with @Autowired
:
@RestController
public class TestController {
private RestTemplate restTemplate;
@Autowired
public TestController(RestTemplate restTemplate) {
this.restTemplate = restTemplate;
}
// ...
}
Constructor injection is a recommended best practice, as it promotes better testability and reduces coupling between components. It can also help in resolving issues related to autowiring.
Conclusion and Call-to-Action ✅📣
By following the steps outlined in this guide, you should be able to fix the "Could not autowire field: RestTemplate" error in your Spring Boot application. Make sure to check your Maven dependencies, restart your application, and verify your component scanning. If all else fails, consider using constructor injection as a more robust approach.
If you found this post helpful, share it with your friends and colleagues who might be facing a similar issue. Let's spread the knowledge! 🔗💡
Have you encountered any other Spring Boot errors or challenges? Let me know in the comments below, and I'll be happy to help. Happy coding! 👩💻💥
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
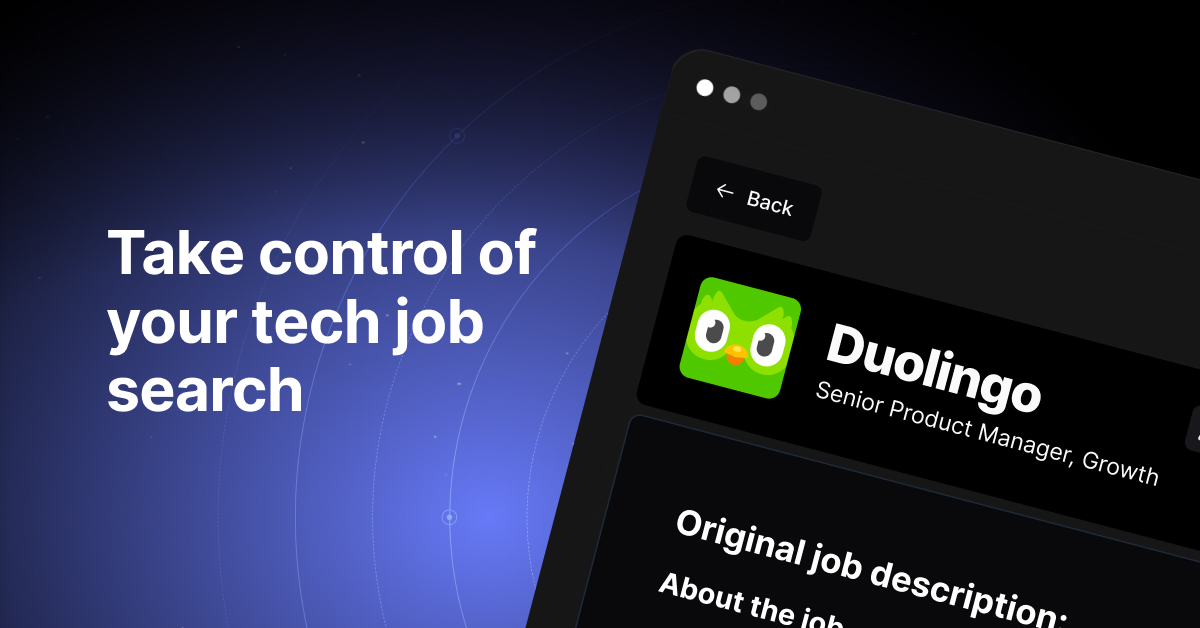