Converting JSON data to Java object
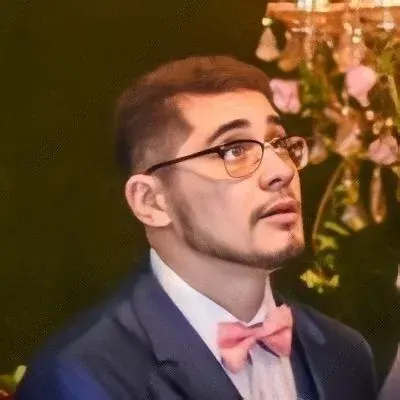
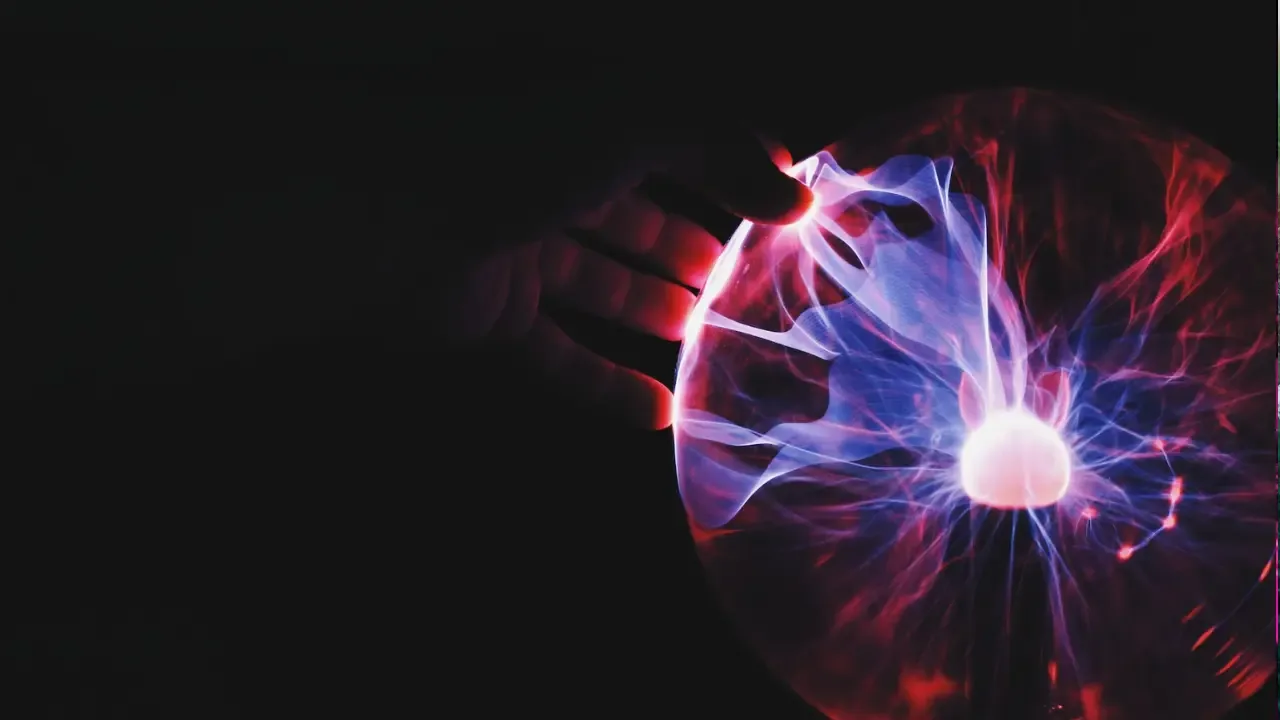
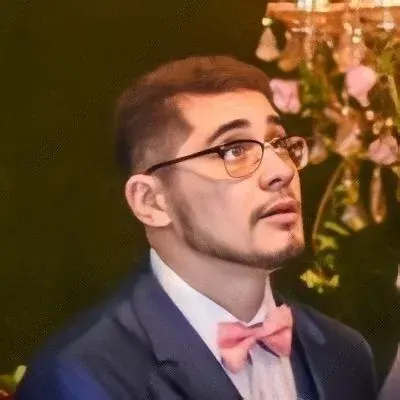
Converting JSON data to Java object: A Complete Guide
So you have a JSON string and you want to convert it into a Java object? 🤔 No worries, we've got you covered! In this guide, we'll walk you through the process of converting JSON data to Java objects, addressing common issues and providing easy solutions along the way. Let's dive in! 💪
Understanding the Problem
Before we start, let's take a closer look at the problem statement. You have a JSON string that represents a hierarchical structure, where each JSON object may contain an array of other JSON objects. Your goal is to extract a list of IDs from the JSON objects that have a "group" property containing other JSON objects.
Choosing a JSON Library
To convert JSON data to Java objects, we recommend using a JSON library. In this guide, we'll be using Google's Gson library, which provides a simple and efficient way to convert between JSON and Java objects.
Step 1: Add Gson Dependency
First things first, you need to add the Gson dependency to your project. If you're using Maven, you can include the following dependency in your pom.xml
file:
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.8</version>
</dependency>
If you're using Gradle, you can add the following line to your build.gradle
file:
implementation 'com.google.code.gson:gson:2.8.8'
Step 2: Create Java Classes
Next, you need to create Java classes that correspond to the structure of your JSON data. In the provided example, you can create classes like JsonData
, Group
, and Child
to represent the JSON structure.
public class JsonData {
private String title;
private int id;
private boolean children;
private List<Group> groups;
// getters and setters
}
public class Group {
private String title;
private int id;
private boolean children;
private List<Group> groups;
// getters and setters
}
public class Child {
private String title;
private int id;
private boolean children;
private List<Group> groups;
// getters and setters
}
Step 3: Convert JSON to Java Object
Now, it's time to convert your JSON string into a Java object using Gson. Here's how you can do it:
import com.google.gson.Gson;
public class Main {
public static void main(String[] args) {
String jsonString = "{ ... }"; // Replace with your actual JSON string
Gson gson = new Gson();
JsonData jsonData = gson.fromJson(jsonString, JsonData.class);
// Access properties of the Java object
System.out.println(jsonData.getTitle());
System.out.println(jsonData.getId());
// Perform further operations or access nested objects and arrays
}
}
That's it! 🎉 You have successfully converted your JSON string to a Java object using Gson. You can now access the properties of the Java object and perform any further operations you need.
Common Issues and Solutions
Issue 1: Malformed JSON
If your JSON string is malformed or contains syntax errors, Gson may throw a JsonSyntaxException
. Make sure your JSON string is valid and properly formatted.
Issue 2: Mismatched Field Names
If your Java class field names do not match the corresponding JSON keys, Gson will not be able to map the JSON data correctly. Use the @SerializedName
annotation to specify the JSON key for each field.
import com.google.gson.annotations.SerializedName;
public class JsonData {
@SerializedName("title")
private String dataTitle;
// ...
}
Issue 3: Missing Dependencies
If you encounter any issues related to missing dependencies or class not found errors, make sure you have added the Gson dependency correctly to your project.
Conclusion and Call-to-Action
Converting JSON data to Java objects doesn't have to be a daunting task. With the help of Gson, you can easily map your JSON string to Java classes and access the data efficiently.
Now that you know how to convert JSON data to Java objects, why not try it out on your own? Share your success stories, examples, or any questions you might have in the comments below. Happy coding! 😊🚀