Converting ISO 8601-compliant String to java.util.Date
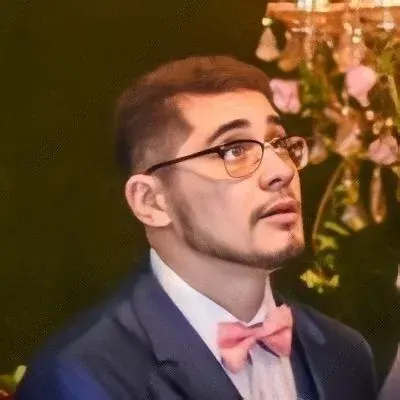
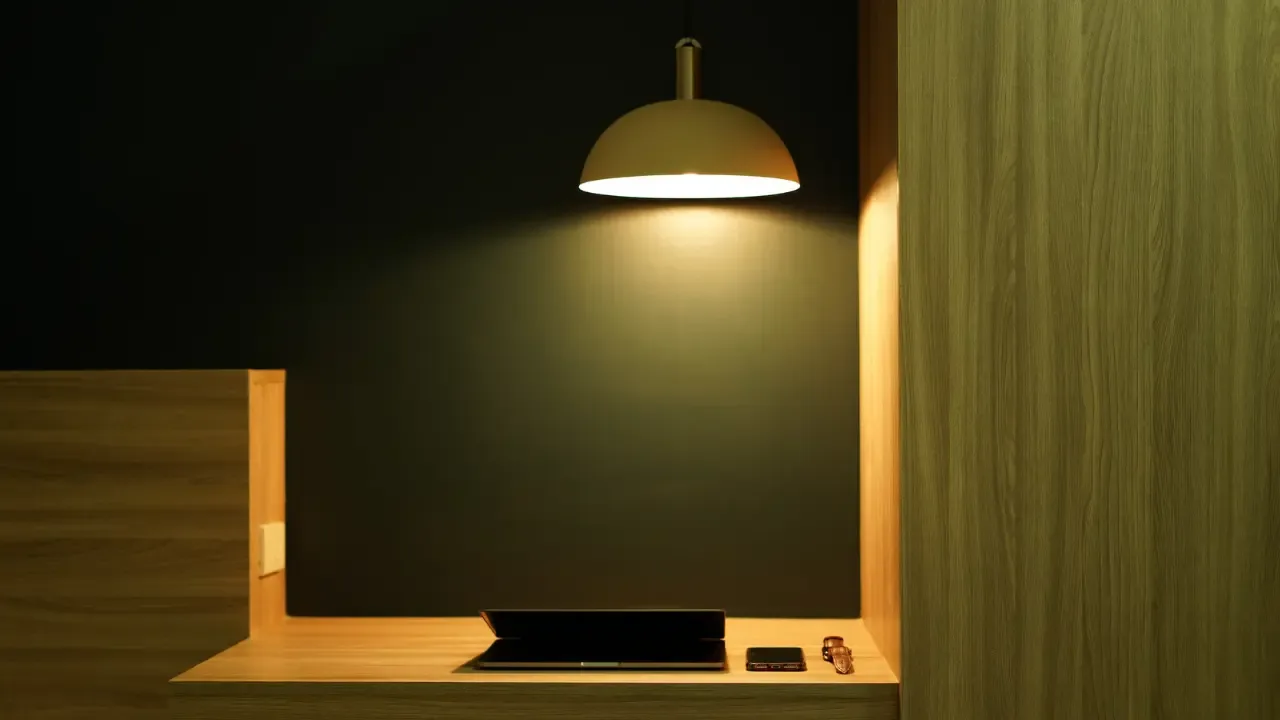
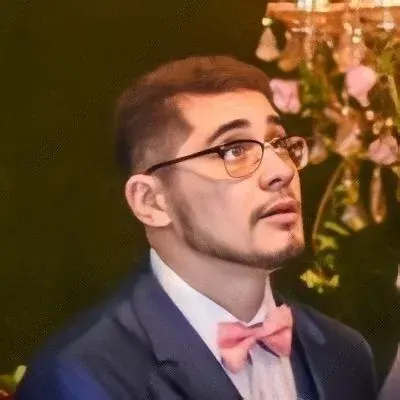
Converting ISO 8601-compliant String to java.util.Date: A Simple Solution ✨
Are you struggling to convert an ISO 8601 formatted string to a java.util.Date
in your Java application? Don't worry, you're not alone! Many developers face this challenge, but fear not - we've got you covered with a simple solution.
Understanding the Problem 🤔
The ISO 8601 format is widely used for representing dates and times in a standardized way. It includes various elements such as the year, month, day, hour, minute, second, and even timezone information. However, when it comes to converting such strings to a java.util.Date
, we can encounter some common issues.
The original pattern to convert an ISO 8601 string to a java.util.Date
using SimpleDateFormat
is yyyy-MM-dd'T'HH:mm:ssZ
, which works fine with a specific locale. However, for some reason, you may encounter problems when dealing with strings like 2010-01-01T12:00:00+01:00
, where the colon in the timezone offset is causing issues.
The Current Solution 😕
One workaround, as suggested in the question, is to temporarily remove the colon from the timezone offset using the replaceAll()
method. Here's an example:
SimpleDateFormat ISO8601DATEFORMAT = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ssZ", Locale.GERMANY);
String date = "2010-01-01T12:00:00+01:00".replaceAll("\\+0([0-9]){1}\\:00", "+0$100");
System.out.println(ISO8601DATEFORMAT.parse(date));
Although this solution works, it's not the most elegant or intuitive. Don't worry; there's a better way!
A Better Solution with Joda-Time 🌟
Thanks to the wonders of the Joda-Time library, there's an easier and more straightforward solution for parsing ISO 8601 strings and converting them to a date object.
First, you'll need to include the Joda-Time library in your project. You can find it here.
Once you have the library set up, you can use the DateTimeFormatter
class from Joda-Time to parse the ISO 8601 string. Here's how you can do it:
DateTimeFormatter parser = ISODateTimeFormat.dateTimeNoMillis();
String jtdate = "2010-01-01T12:00:00+01:00";
System.out.println(parser.parseDateTime(jtdate));
Alternatively, if you prefer a more concise approach, you can directly create a DateTime
object using the ISO 8601 string. Check out this example:
DateTime dt = new DateTime("2010-01-01T12:00:00+01:00");
And that's it! With Joda-Time, you can effortlessly parse and convert an ISO 8601-compliant string to a date object without any fuss.
Embrace the Simplicity ✨
Converting ISO 8601 strings to java.util.Date
objects doesn't have to be a complex task. By leveraging the power of Joda-Time, you can simplify your code and achieve more elegant solutions.
So, go ahead and try out this approach in your next project. Don't let the complexities of date and time conversions hold you back - embrace the simplicity, save time, and keep coding cool! 😎
Have you tried any other methods or libraries for handling ISO 8601 conversions in Java? Share your thoughts and experiences in the comments below. Let's learn and grow together!
✍️ Written by [Your Name] | 🗓️ [Date]
📢 Call to Action:
If you found this guide helpful and want to learn more time-saving tips and tricks, make sure to subscribe to our newsletter. Stay up-to-date with the latest tech trends and join a community of passionate developers.
Click here to sign up now and level up your coding game! 🚀