Converting "ArrayList<String> to "String[]" in Java
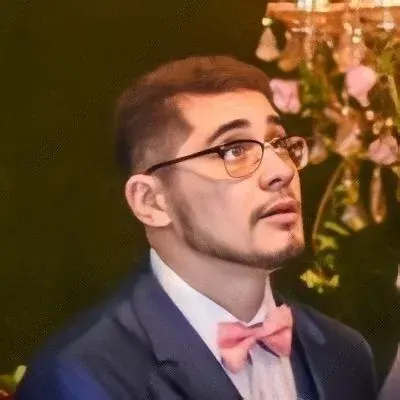
![Cover Image for Converting "ArrayList<String> to "String[]" in Java](https://images.ctfassets.net/4jrcdh2kutbq/69jEroxggJHYqowis93Xim/658e398d5146e541419a17307ade256a/Untitled_design.webp?w=3840&q=75)
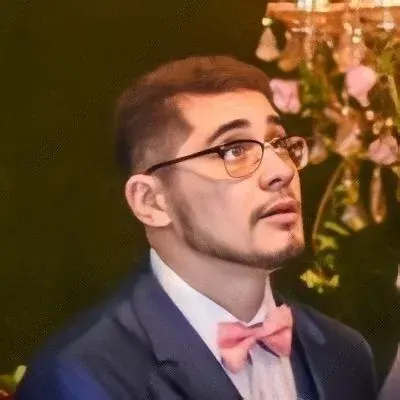
Converting ArrayList<String>
to String[]
in Java: A Super Easy Guide! 🚀
So, you have an ArrayList<String>
object and you want to convert it to a String[]
array in Java. We've got you covered! In this guide, we'll walk you through the process step by step, addressing common issues and providing easy solutions. 🙌
The problem: ArrayList<String>
to String[]
Let's first understand the problem at hand. You have an ArrayList<String>
object, which is a dynamic array in Java that can grow or shrink in size. On the other hand, you need to convert it into a String[]
array, which is a fixed-size array.
The easy solution: Using the toArray()
method 💡
Java makes our lives easier with the toArray()
method, which is available for ArrayList
objects. This method allows us to convert an ArrayList
into an array of the desired type. Fantastic, right?
Here's a code snippet to help you out:
ArrayList<String> myList = new ArrayList<>();
myList.add("Hello");
myList.add("World");
myList.add("!");
String[] myArray = myList.toArray(new String[myList.size()]);
In the above code, we create an ArrayList<String>
called myList
and add some elements to it. Then, we use the toArray()
method to convert it to a String[]
array. The toArray()
method expects an array of the desired type as an argument, so we pass new String[myList.size()]
to indicate the size of the resulting array.
Common issues and additional tips 🔍
1. Handling empty arrays
If your ArrayList<String>
is empty, you might encounter a slight hiccup. The resulting String[]
array would be of size 0, but fear not! By passing an array of the desired type and size (even if empty) to toArray()
, you can ensure that you get the correct type of array.
ArrayList<String> emptyList = new ArrayList<>();
String[] emptyArray = emptyList.toArray(new String[0]);
2. Performance considerations
Keep in mind that calling toArray()
with an argument array of appropriate size results in a performance boost. Java will fill in the array internally instead of creating a new one and copying elements. So, if you know the size of your ArrayList
beforehand, it's beneficial to pass an appropriately sized array to the toArray()
method.
Your turn to shine! ✨
You've made it this far! Now, it's your turn to give it a try! Convert your ArrayList<String>
to a String[]
array using the awesome toArray()
method. Experiment with different scenarios and let us know how it went!
Join the conversation!
We love hearing from our readers! If you have any questions, suggestions, or additional tips about converting ArrayList<String>
to String[]
arrays in Java, feel free to leave a comment below. Let's chat and learn together! 🎉