Converting array to list in Java
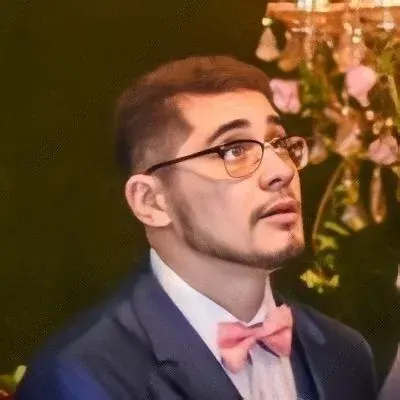
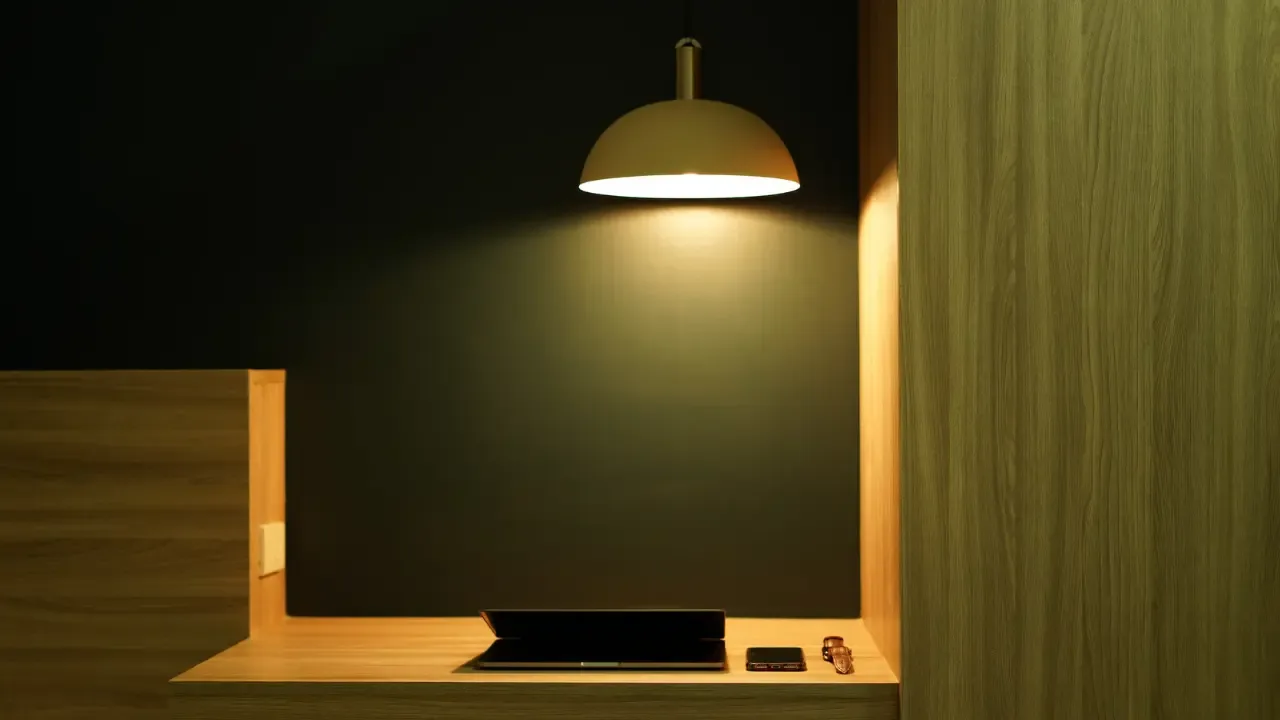
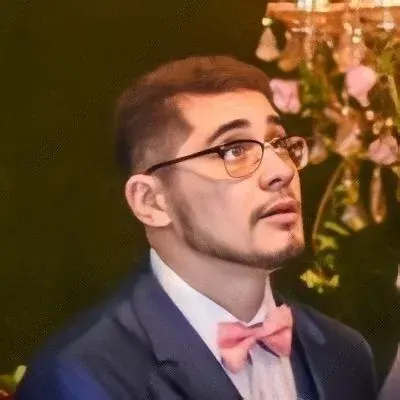
Converting Array to List in Java: The Dilemma 😕
So you want to convert an array to a list in Java, but you've stumbled upon some unexpected behavior? Don't worry, you're not alone! Many developers have encountered this confusion when using the Arrays.asList()
method. Let's dig deeper into this array-to-list conversion conundrum and explore the solutions together! 💪
Understanding the Issue 👀
The problem lies in the change of behavior and signature of the Arrays.asList()
method between Java SE 1.4.2 and Java 8. In the old days, using Arrays.asList()
would yield a list containing the elements of the array. However, from Java 1.5.0 onwards, the method wraps the entire array as a single element in the resulting list. Ouch! That's a sneaky change! 😱
Unveiling the Unexpected Result 🕵️♀️
To grasp the issue more clearly, let's take a look at an example:
int[] numbers = new int[] { 1, 2, 3 };
List<int[]> convertedList = Arrays.asList(numbers);
On Java 1.4.2, convertedList
would contain the elements [1, 2, 3]
just as you'd expect. However, starting from Java 1.5.0, the resulting list would actually be [[1, 2, 3]]
, wrapping the entire numbers
array as a single element. This can lead to inadvertent mistakes and confusion if not handled carefully. 😅
Easy Solutions to the Rescue! 🚀
Fear not! We have some simple and effective solutions for you to convert the array to a list, regardless of the Java version you're using.
Solution 1: Manual Conversion using a Loop
The most straightforward approach is to manually convert each element of the array to a list element using a loop. Here's an example:
int[] numbers = new int[] { 1, 2, 3 };
List<Integer> convertedList = new ArrayList<>();
for (int number : numbers) {
convertedList.add(number);
}
By iterating over the array's elements and adding them to the list manually, you ensure consistent behavior across Java versions. It may require a few extra lines of code, but it's a reliable solution that gives you the desired outcome. 🙌
Solution 2: Utilize Java 8 Streams
If you're fortunate enough to be using Java 8 or above, you can leverage the power of Java streams to streamline the conversion process. Here's how:
int[] numbers = new int[] { 1, 2, 3 };
List<Integer> convertedList = Arrays.stream(numbers)
.boxed()
.collect(Collectors.toList());
Using the Arrays.stream()
method, you convert the array to an IntStream
, followed by the boxed()
operation to obtain a Stream<Integer>
. Finally, with the collect()
method, you transform the stream into a list. Voilà! 🎉
Ensuring Safety and Avoiding Pitfalls 🚧
When using the Arrays.asList()
method, it's crucial to be aware of the described behavior difference and choose the appropriate solution accordingly. Applying the wrong method could lead to subtle bugs and unexpected results, so pay attention! 😮
To make your code more resilient, consider writing unit tests that cover the array-to-list conversion scenarios, ensuring the expected behavior across different Java versions. Safety first! 🔒
Share Your Wisdom! 📢
Now that you're armed with multiple solutions to tackle the array-to-list conversion challenge, it's your turn to shine! Share your experiences, learnings, and any additional tips you might have encountered along the way. Let's help the developer community grow together! 🌱
Leave a comment below and let us know which solution worked best for you. Have you experienced any unexpected behavior related to this issue? We'd love to hear your stories! 😄