Convert InputStream to byte array in Java
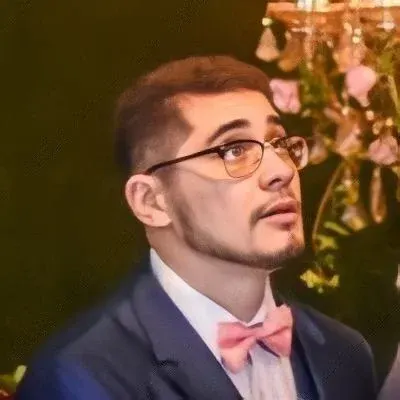
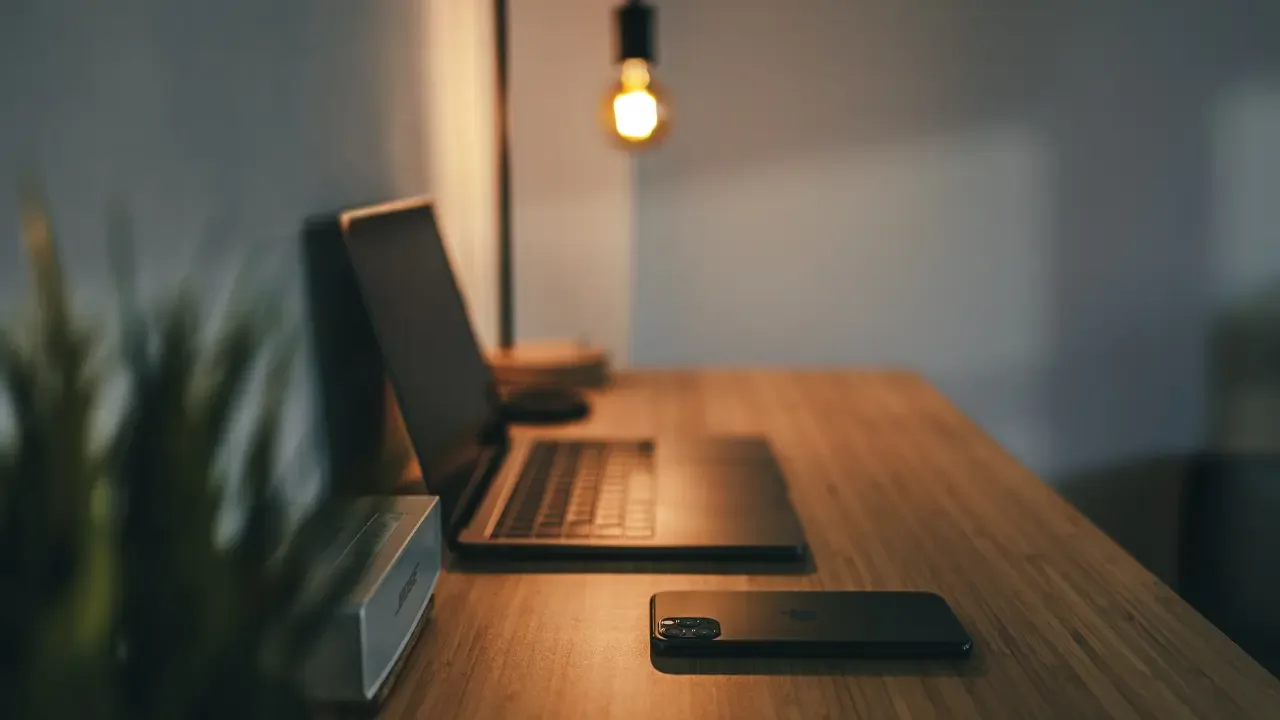
📝💻 Tech Blog Post: Converting InputStream to Byte Array in Java
Hey tech wizards! Do you find yourself scratching your head when it comes to converting an InputStream into a byte array in Java? Fret not! I've got you covered with some super-easy solutions that will save you time and frustration. Let's dive right into it! 🏊♂️
The Common Challenge
One of the most common challenges we face as Java developers is reading an entire InputStream into a byte array. We all know that an InputStream provides a way to read data from a source, but sometimes we need to convert that data into a byte array for further processing.
The Solution: Let's unveil the magic trick! 🎩
Luckily, Java provides a built-in class called ByteArrayOutputStream
that can help us achieve this effortlessly. Here's a step-by-step guide to solving this conundrum:
First, create an instance of
ByteArrayOutputStream
:
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
Next, create a byte array, commonly referred to as a buffer, to store the read data from the InputStream:
byte[] buffer = new byte[4096]; // You can choose the buffer size according to your needs
Now, we're ready to read the data from the InputStream and write it to the ByteArrayOutputStream. We'll need to iterate until we reach the end of the InputStream:
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
Finally, we can retrieve the byte array representing the data:
byte[] byteArray = outputStream.toByteArray();
That's it! 🎉 You successfully converted the InputStream into a byte array without breaking a sweat!
Boost your skills: Advanced Tips
Handling Exceptions: Remember to handle exceptions like
IOException
. Wrap your code with atry-catch
block or propagate the exception using thethrows
keyword.
try {
// The code for reading the InputStream and converting it to a byte array
} catch (IOException e) {
// Handle the exception or propagate it using throws
}
Closing Streams: It's important to close the InputStream and the ByteArrayOutputStream after you're done using them. To ensure proper resource management, enclose the code within a
try-with-resources
statement:
try (InputStream inputStream = ...; ByteArrayOutputStream outputStream = ...) {
// The code for reading the InputStream and converting it to a byte array
} catch (IOException e) {
// Handle the exception or propagate it using throws
}
Don't Stop, Engage! 📣
I hope this guide helped you effortlessly convert an InputStream to a byte array in Java. Now it's your turn to put this knowledge into practice! Share in the comments below if you have any questions or have encountered any interesting use cases for this conversion.
Spread the tech love! Share this post with your fellow Java developers who might find it helpful. Happy coding, my friends! 💻❤️
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
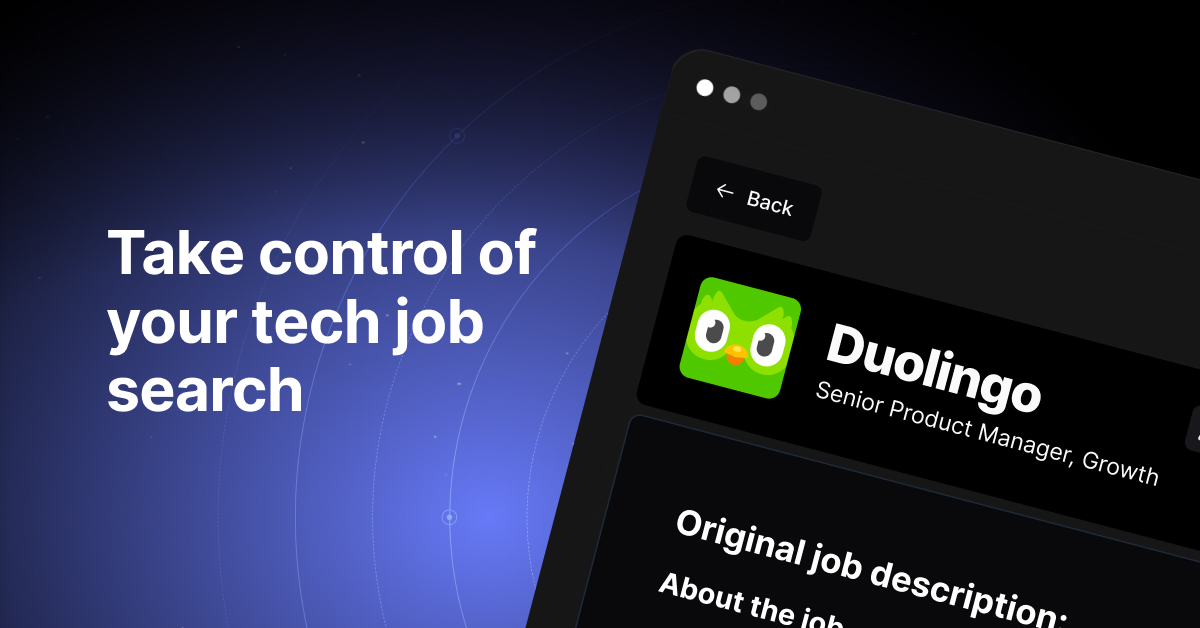