Connection Java - MySQL : Public Key Retrieval is not allowed
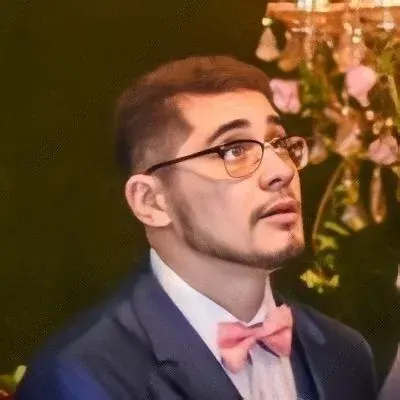
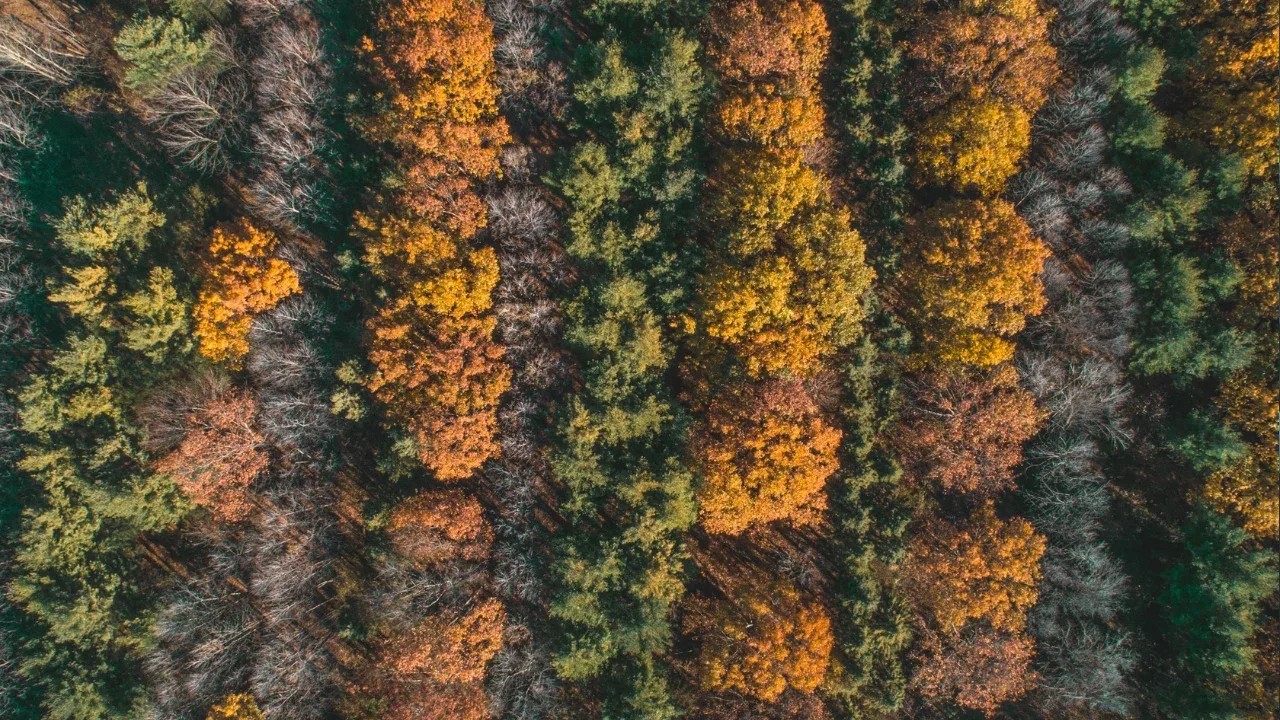
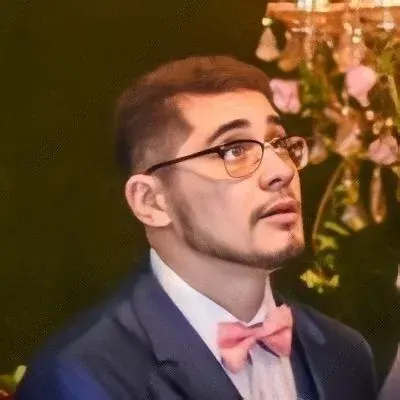
📝 Blog Post: Connection Java - MySQL : Public Key Retrieval is not allowed
Connecting Java to a MySQL database should be a breeze, but sometimes you run into those pesky exceptions that can make your head spin. One common issue that might arise is the "Public Key Retrieval is not allowed" error. Don't worry, though! I'm here to break it down for you and provide easy solutions so you can get your Java and MySQL connection up and running smoothly!
🕵️♂️ Understanding the Issue
So, you're trying to connect to your MySQL database using the Connector 8.0.11, and everything seems fine until you hit an exception. The exception message looks something like this:
Exception in thread "main" java.sql.SQLNonTransientConnectionException: Public Key Retrieval is not allowed at com.mysql.cj.jdbc.exceptions.SQLError.createSQLException(SQLError.java:108) at ...
This error occurs when the MySQL server doesn't allow fetching the public key, which is required for secure SSL/TLS connections. By default, Connector/J 8.0+ enforces stricter security measures, leading to this exception.
💡 Easy Solutions
No need to panic! Here are a couple of easy solutions to fix the "Public Key Retrieval is not allowed" error:
1. Disabling SSL/TLS
One straightforward solution is to disable SSL/TLS encryption for the connection. To do this, you can modify your ConnectionManager
class as follows:
public static Connection getConnection() throws SQLException {
MysqlDataSource dataSource = new MysqlDataSource();
dataSource.setUseSSL(false);
// ...
return dataSource.getConnection();
}
By setting setUseSSL(false)
, you disable SSL/TLS for the MySQL connection, and the exception should disappear. However, make sure to only use this solution if your connection doesn't contain sensitive data, as it could potentially compromise security.
2. Allowing Public Key Retrieval
If maintaining SSL/TLS encryption is crucial for your connection, you can modify the JDBC URL to allow public key retrieval. Here's an example of how it could look:
public static final String jdbcURL = "jdbc:mysql://localhost/database?allowPublicKeyRetrieval=true";
By appending allowPublicKeyRetrieval=true
to the JDBC URL, you instruct Connector/J to allow fetching the public key, resolving the issue.
🚀 Time to Engage!
I hope these solutions helped you overcome the "Public Key Retrieval is not allowed" error when connecting Java to a MySQL database. Now it's your turn to take action!
👉 Try implementing one of the solutions provided in your code and see if it resolves the issue.
👉 Have any other exceptional connection problems? Comment below, and let's solve them together!
Remember, troubleshooting database connections can be daunting, but armed with the right knowledge, you'll conquer any challenge that comes your way! Happy coding! 😄