Comparing Java enum members: == or equals()?
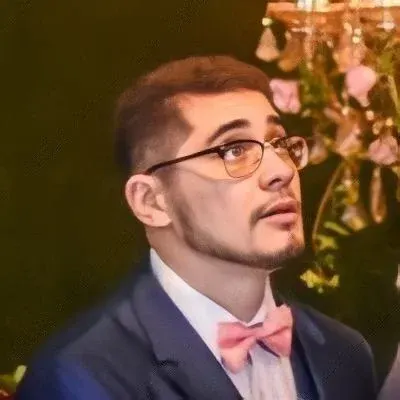
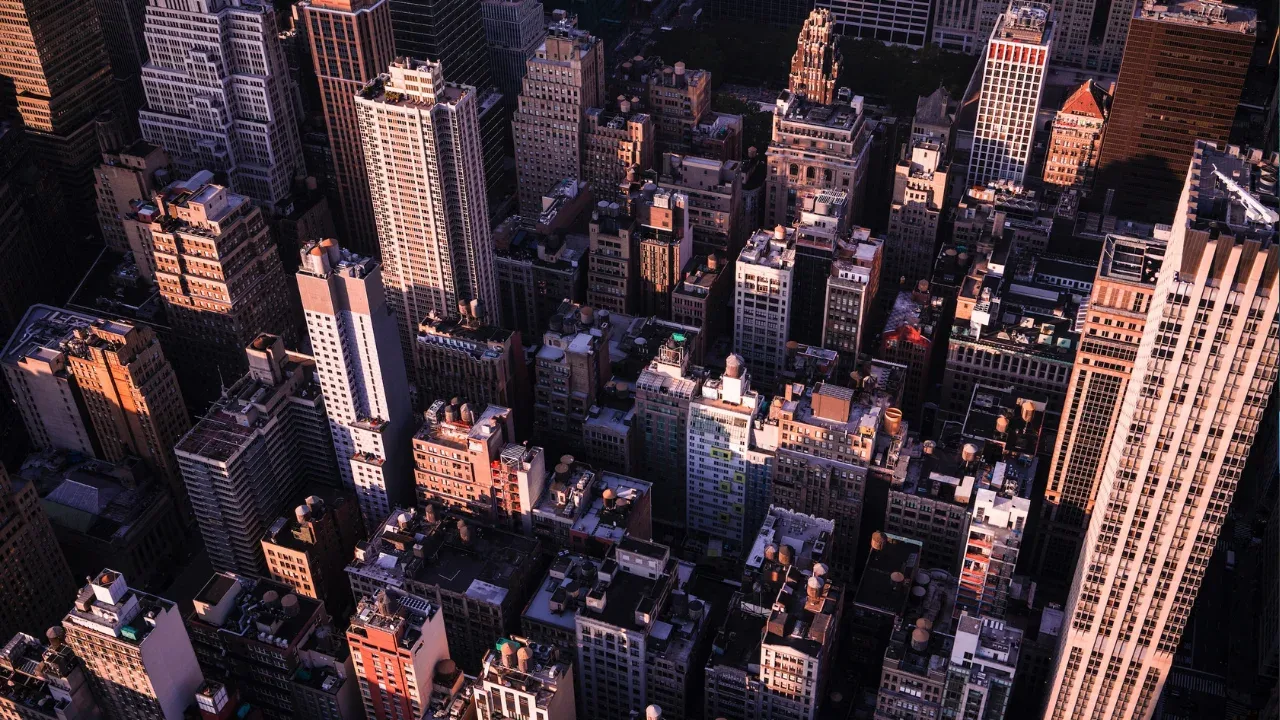
Comparing Java enum members: == or equals()? š¤
š Hey there, fellow Java developers! Today's topic is all about comparing Java enum members. We're going to answer the burning question: should we use the ==
operator or the .equals()
method? Let's clear the air and put an end to this confusion! š
Understanding the Context š
Java enums, as we know, are compiled to classes with private constructors and a bunch of public static members. When comparing two members of a given enum, we commonly use the .equals()
method. But wait, there's a twist! Recently, we stumbled upon some code that used the equals operator ==
instead. Gather around, and let's dig deeper into this dilemma! š”
.equals() or ==: The Big Debate āļø
First things first, the ==
operator checks for reference equality, while the .equals()
method checks for content equality. So, which one should we use when comparing Java enum members? š¤
The Power of the .equals() Method š
The .equals()
method inherited from the Object
class has been Java's go-to way of comparing objects for a long time. It allows us to compare the content of the objects, which is perfect for most use cases. It goes something like this:
public useEnums(SomeEnum a) {
if (a.equals(SomeEnum.SOME_ENUM_VALUE)) {
...
}
...
}
This approach compares the content of a
with the content of SomeEnum.SOME_ENUM_VALUE
. If they match, we execute the corresponding logic. Easy peasy! š
The Might of the == Operator šŖ
But what about the ==
operator? Should we neglect it altogether? Not so fast! The ==
operator is designed to check the reference equality of objects. It compares the memory addresses to determine if the objects are actually the same object instance, regardless of their content.
public useEnums2(SomeEnum a) {
if (a == SomeEnum.SOME_ENUM_VALUE) {
...
}
...
}
Using ==
in this scenario compares the memory addresses of a
and SomeEnum.SOME_ENUM_VALUE
. If they point to the same location, you hit the jackpot! š
Knowing When to Use Each šÆ
We've explored the nature of both .equals()
and ==
. Now, it's time to discover which one is the right fit for different situations!
ā
Use .equals()
:
When comparing enum members for content equality.
When you want to match enum members with different references but identical content.
š« Avoid using .equals()
:
When you specifically want to check if two enum members are the same object instance, not just if their content matches.
ā
Use ==
:
When comparing enum members for reference equality.
When you want to check if two enum members are the exact same object instance.
š« Avoid using ==
:
When you need to compare the content of enum members, rather than their references.
Call to Action! š
With this newfound knowledge, you can now confidently navigate the world of Java enum comparisons! Embrace .equals()
for content comparison and use ==
to unleash the power of reference equality. Remember, each has its moment to shine! āØ
If you found this guide helpful or have any other burning Java questions, feel free to leave us a comment below. Let's keep the conversation going! Happy coding! š
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
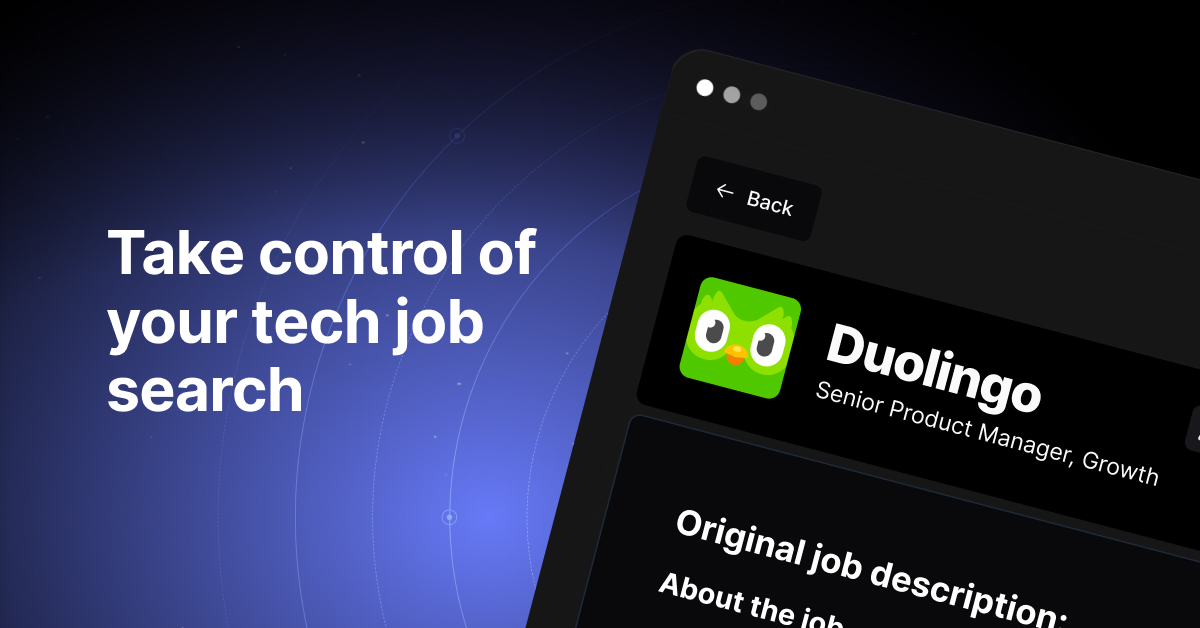