Can you use @Autowired with static fields?
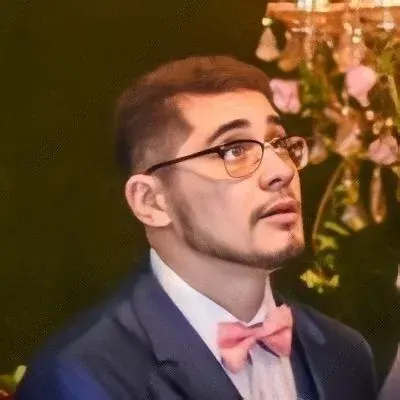
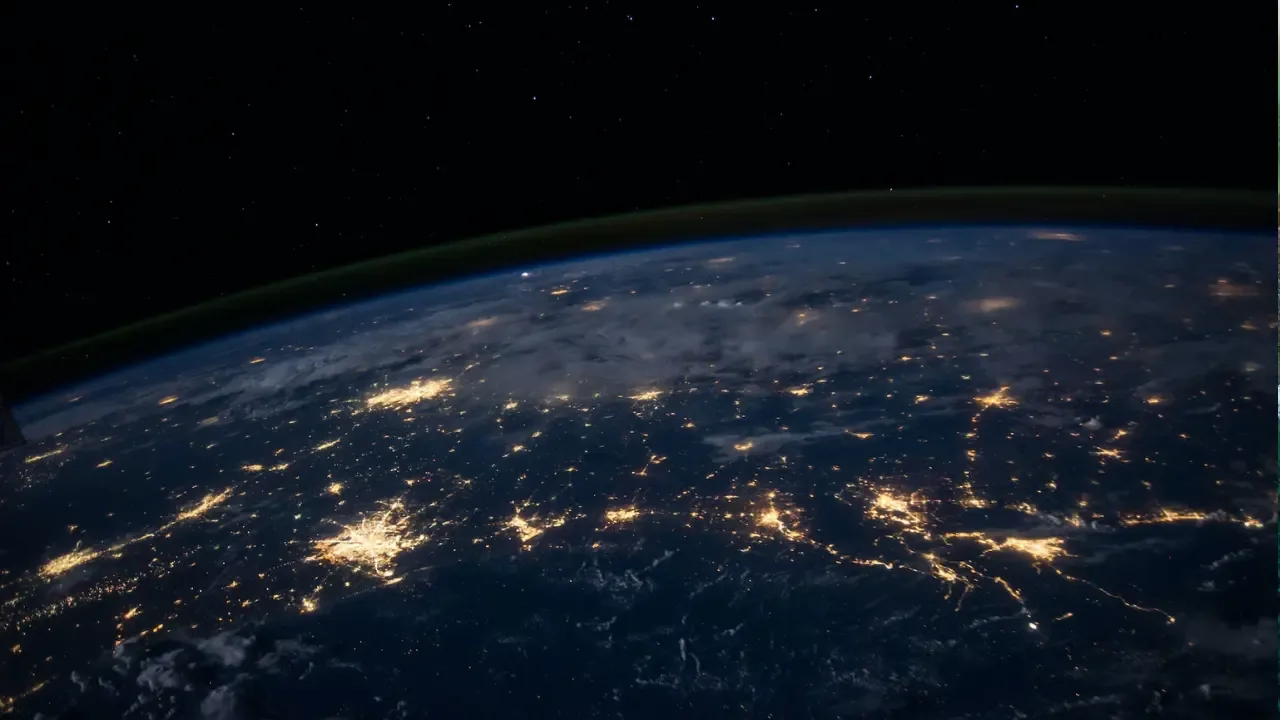
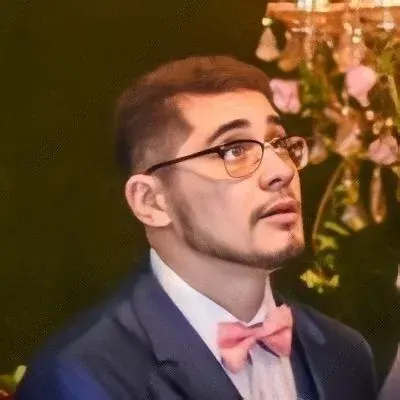
📝🔥 Blog Post: Can you use @Autowired with static fields?
Intro:
Hey tech enthusiasts! 👋 Have you ever wondered if you can use the mighty @Autowired
annotation with static fields? Well, keep reading because we're going to dive into this intriguing question and unravel the mystery behind it. 😉
Problem:
You might have stumbled upon a situation where you desperately needed to inject a dependency into a static field. You thought to yourself, "Can I sprinkle some @Autowired
magic here?" Unfortunately, the answer is no. The reason for this limitation lies in the way @Autowired
works.
Explanation:
The @Autowired
annotation is designed to be used with instance variables or non-static methods. It allows you to automatically wire dependencies into your Spring-managed beans. Since static fields don't belong to a specific instance, but rather to the class itself, it makes sense that @Autowired
cannot be utilized in this context.
Solutions:
While @Autowired
may not be suitable for static fields, fear not! There are alternative approaches you can use to achieve what you need:
Using a setter method: Instead of autowiring the static field directly, create a static setter method and annotate it with
@Autowired
. This way, you can still have your dependency injected, albeit indirectly. Here's an example:public class MyStaticClass { private static MyDependency myDependency; @Autowired public void setMyDependency(MyDependency myDependency) { MyStaticClass.myDependency = myDependency; } }
Initializing the static field manually: If you're unable to use setter methods, you can manually initialize the static field by directly accessing the Spring application context. Here's how:
public class MyStaticClass { private static MyDependency myDependency; @Autowired private ApplicationContext applicationContext; @PostConstruct public void init() { myDependency = applicationContext.getBean(MyDependency.class); } }
Call-to-Action:
And there you have it! While @Autowired
may not be applicable to static fields, you now have two flexible workarounds at your disposal. Experiment with them in your projects and see which one best fits your needs. If you found this information helpful, don't forget to share it with your fellow developers! 😊
Remember, if you have any more questions or need further assistance, drop a comment below and let's get the conversation going! Happy coding! 👨💻🚀