Can not deserialize instance of java.util.ArrayList out of START_OBJECT token
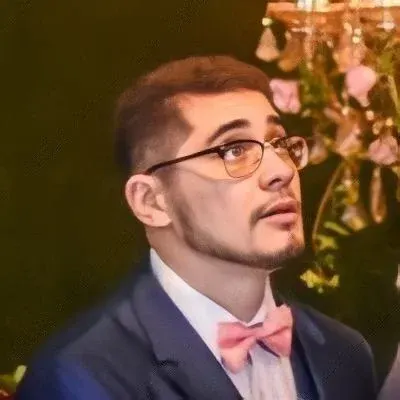
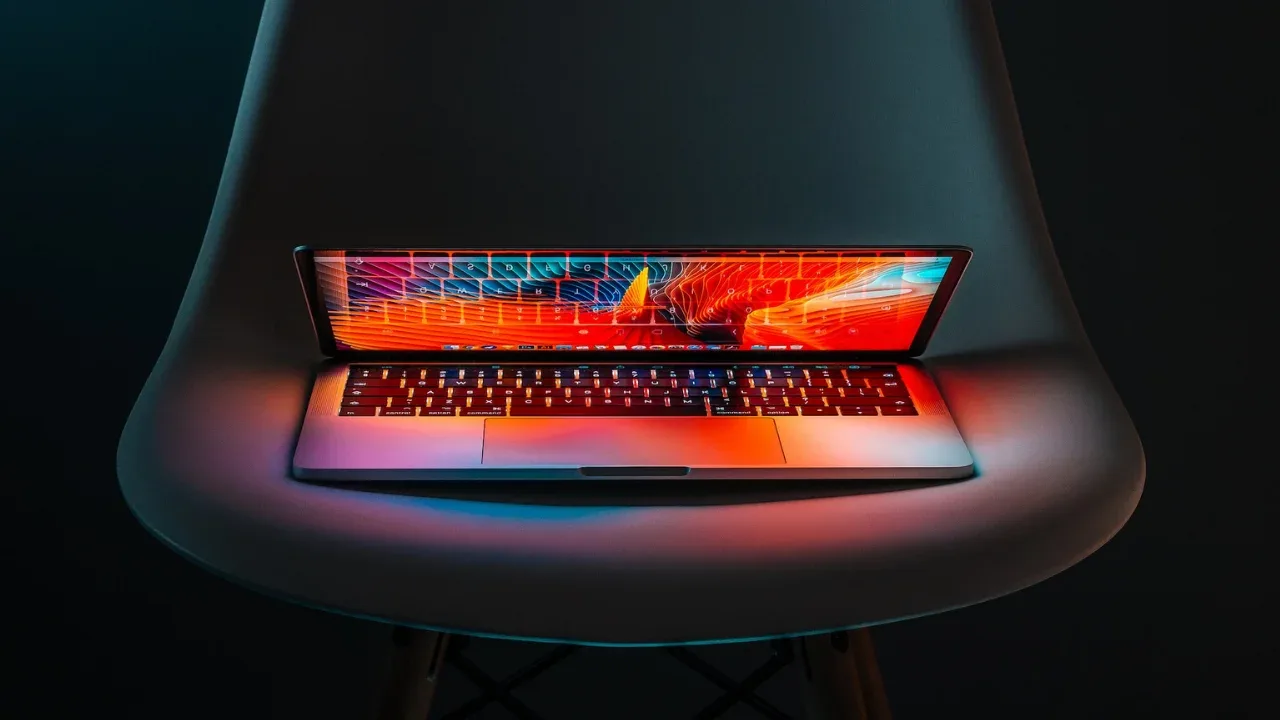
How to Fix the "Can not deserialize instance of java.util.ArrayList out of START_OBJECT token" Error
So, you encountered the dreaded "Can not deserialize instance of java.util.ArrayList out of START_OBJECT token" error. Don't worry, you're not alone! This error is a common issue faced by developers when trying to deserialize JSON into a Java object.
Let's break down the problem and find a solution.
Understanding the Error
The error message indicates that there was an issue deserializing the JSON request body into an ArrayList
object. It specifically mentions the START_OBJECT
token, which suggests that the JSON parser encountered an object instead of an array.
Analyzing the Code
To further understand the issue, let's examine the code you provided.
Server-side code
In the server-side code snippet, you have a JAX-RS resource class called COrderRestService
. It defines a POST
method that accepts a Collection<COrder>
object.
@POST
@Produces(MediaType.APPLICATION_JSON)
@Consumes(MediaType.APPLICATION_JSON)
public Response postOrder(Collection<COrder> orders) {
// ...
}
Entity Class
There's also an entity class called COrder
which you want to deserialize from the JSON request body.
@XmlRootElement
public class COrder {
String name;
String detail;
// ...
}
Finding the Issue
From the code, it seems that you're expecting a JSON array in the request body, but the error suggests that it received a JSON object instead.
To visualize the issue, let's look at the JSON you provided:
{
"collection": [
{
"name": "Test order1",
"detail": "ahk ks"
},
{
"name": "Test order2",
"detail": "Fisteku"
}
]
}
The JSON contains a collection
property, which is an array of objects. However, the server-side code expects the JSON to be an array directly, without any property.
Resolving the Issue
To fix the issue, you need to adjust the JSON structure to match the server-side expectations. Instead of sending an object with a collection
property, send the array directly.
For example, modify the JSON request body as follows:
[
{
"name": "Test order1",
"detail": "ahk ks"
},
{
"name": "Test order2",
"detail": "Fisteku"
}
]
By doing this, your code will be able to deserialize the JSON array correctly.
Your Turn
Now that you understand the problem and have a solution, it's time to try it out. Update your request with the modified JSON structure and see if the issue is resolved.
If you encounter any further issues or have any questions, feel free to reach out in the comments below. Happy coding! 💻😊
Is this blog post helpful? Share it with your fellow developers to save them from the "Can not deserialize instance of java.util.ArrayList out of START_OBJECT token" error! 🚀🔗
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
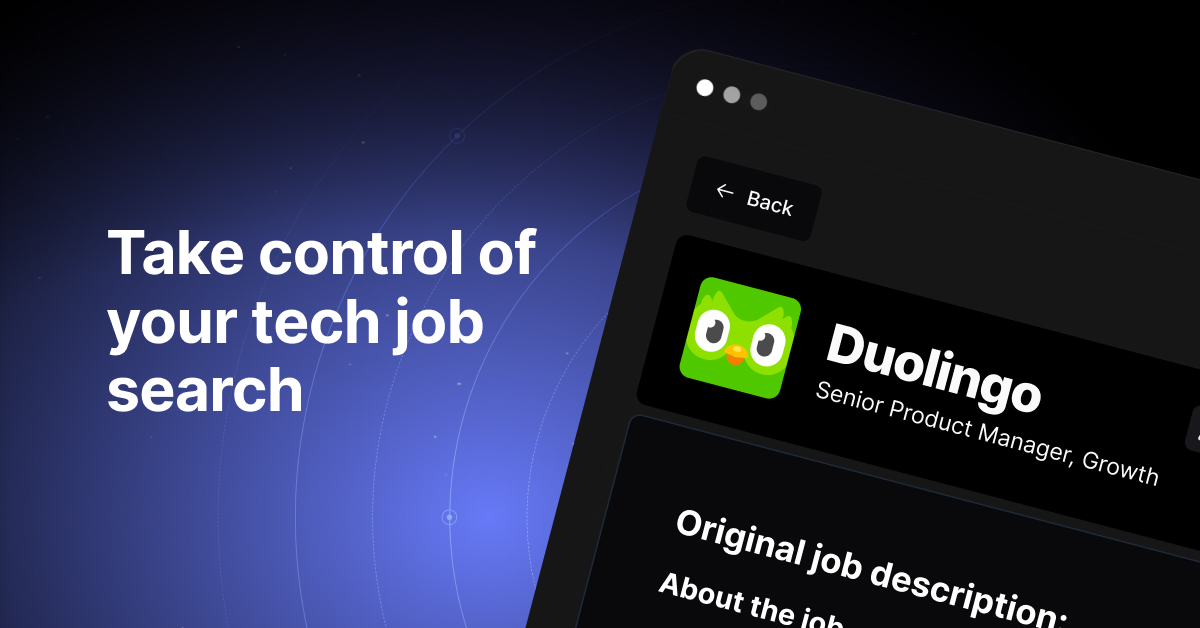