Can I add jars to Maven 2 build classpath without installing them?
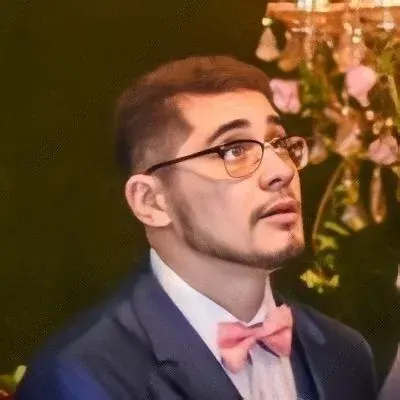
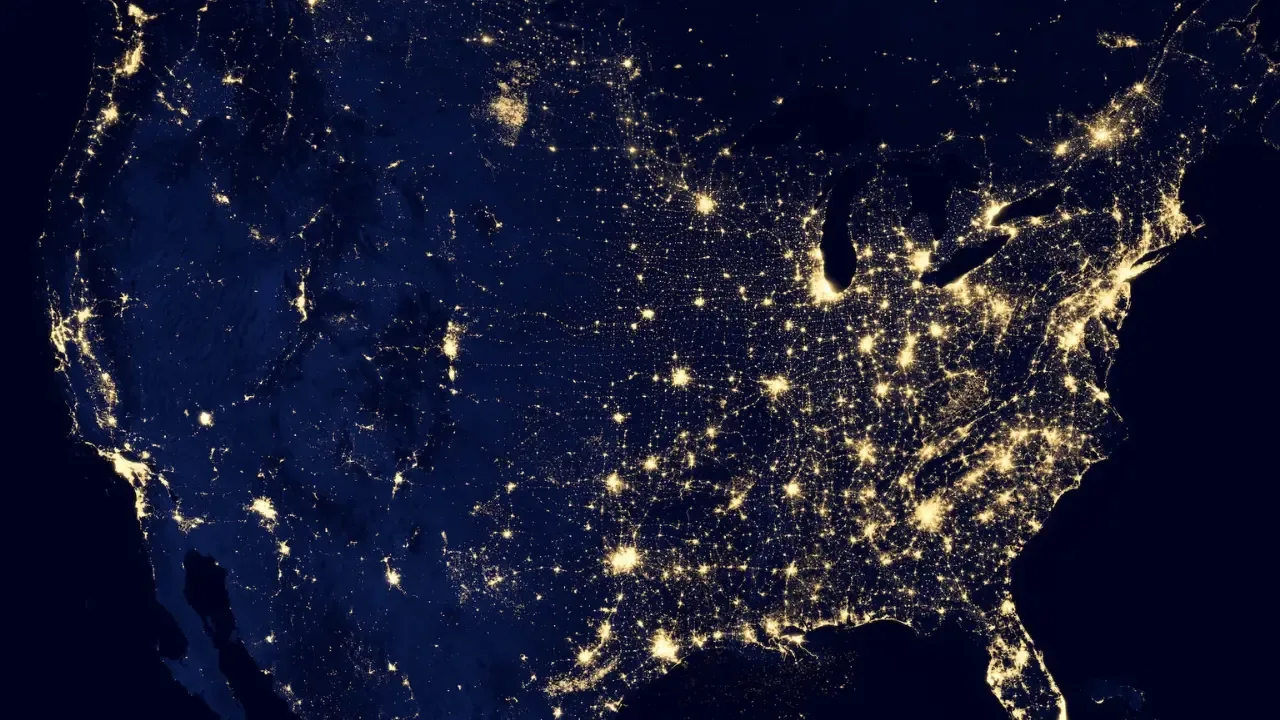
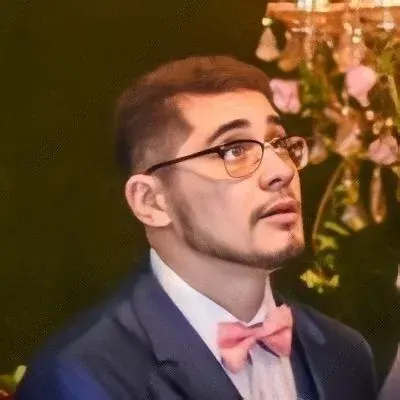
Can I add jars to Maven 2 build classpath without installing them?
š¤š¦š
Are you feeling frustrated with Maven 2 during the experimentation or quick and dirty mock-up phase of development? You have a pom.xml
file that defines the dependencies for the web-app framework you want to use, but sometimes you want to link to a third-party library that doesn't have a pom.xml
file defined. Creating the pom.xml
file for the third-party library by hand, installing it, and adding the dependency to your pom.xml
seems like a hassle. Is there an easier way to handle this?
The Problem
The problem is that you want to include additional JARs in your Maven 2 build classpath without having to install them manually or create a separate pom.xml
file.
The Solution
Fortunately, there are two simple solutions to address this issue:
Solution 1: Using the system scope
You can use the system scope in Maven to specify the JARs in your /lib
directory. Here's how:
Create a
lib
directory in the root of your Maven project (if you haven't already).Copy the JAR files you want to include into the
lib
directory.Add the following dependency to your
pom.xml
file:
<dependency>
<groupId>com.example</groupId>
<artifactId>example-libs</artifactId>
<version>1.0</version>
<scope>system</scope>
<systemPath>${basedir}/lib/example.jar</systemPath>
</dependency>
Make sure to replace com.example
, example-libs
, 1.0
, and example.jar
with the appropriate values for your project.
Solution 2: Using the install-file goal
Another option is to use the install-file
goal to install the JAR files into your local Maven repository. Here's how:
Open your command line or terminal.
Navigate to your project's root directory.
Run the following command for each JAR file you want to include:
mvn install:install-file -Dfile=path/to/your.jar -DgroupId=com.example -DartifactId=example-libs -Dversion=1.0 -Dpackaging=jar
Make sure to replace path/to/your.jar
, com.example
, example-libs
, 1.0
, and jar
with the appropriate values for your project.
Conclusion
By using either the system scope or the install-file goal, you can easily add JAR files to your Maven 2 build classpath without the need for manual installation or creating separate pom.xml
files. Experimentation and quick mock-up phases of development will become less frustrating as you can quickly include third-party libraries without any hassle.
So, the next time you need to include additional JARs, remember to try these simple solutions and save time during your development process. Happy coding! šš©āš»šØāš»
Now it's your turn: Have you ever encountered this issue during your development? How did you solve it? Share your experiences in the comments below! š