Calling a @Bean annotated method in Spring java configuration
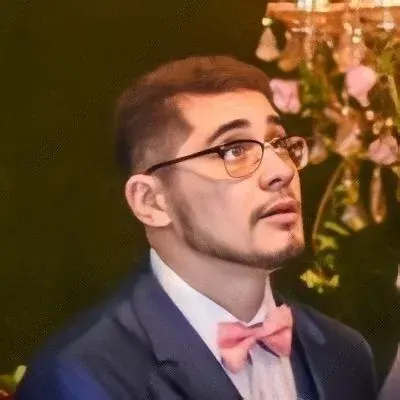
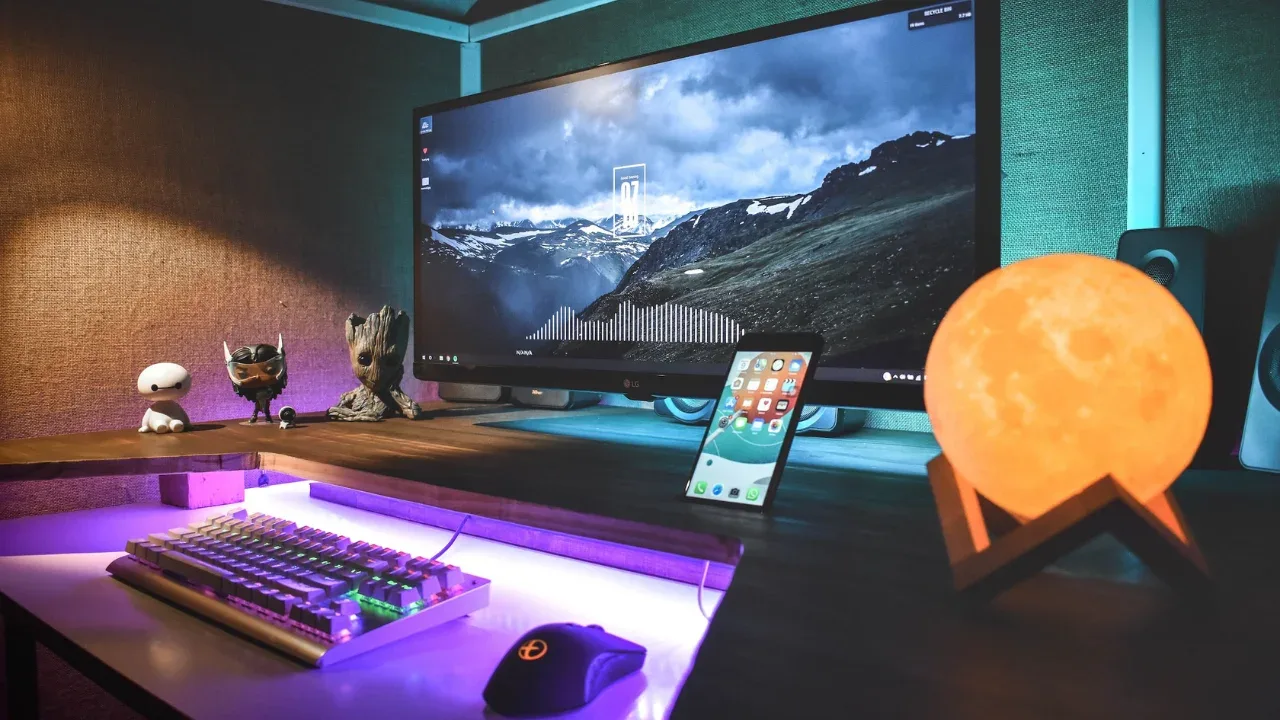
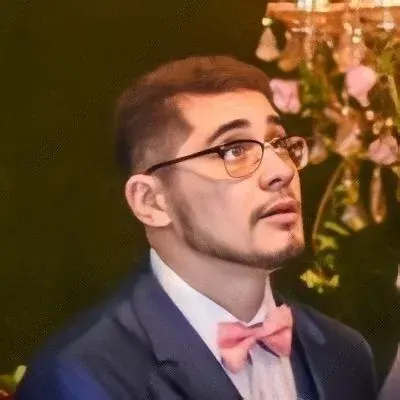
๐ Calling a @Bean annotated method in Spring Java Configuration: Demystified! ๐ฑ
Are you curious about how Spring injection handles calling methods with the @Bean annotation? ๐ค You might have wondered if putting the @Bean annotation on a method tells Spring to create a bean by calling that method and getting the returned instance. But what happens when that bean needs to be used to wire other beans or set up other code? And how does Spring prevent multiple instances of the bean from floating around? ๐คทโโ๏ธ
Let's dive into this intriguing topic and unravel the magic of @Bean annotated methods! ๐ฉโจ
๐ Understanding the @Bean Annotation
When you annotate a method with @Bean in your Spring Java configuration, you're essentially telling Spring that the method should be used to create and configure a bean. The method will be invoked by Spring, and its returned instance will be considered as the bean. ๐ฑ
๐ Wiring Beans and Setup
But what about wiring beans and setup processes that require the use of this @Bean annotated method? How do you ensure consistent access to the same instance of the bean? Let's look at an example to see it in action: ๐๏ธโโ๏ธ
@Bean
public BasicAuthenticationEntryPoint entryPoint() {
BasicAuthenticationEntryPoint basicAuthEntryPoint = new BasicAuthenticationEntryPoint();
basicAuthEntryPoint.setRealmName("My Realm");
return basicAuthEntryPoint;
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.exceptionHandling()
.authenticationEntryPoint(entryPoint())
.and()
.authorizeUrls()
.anyRequest().authenticated()
.and()
.httpBasic();
}
In this code snippet, the entryPoint()
method is annotated with @Bean, indicating that it should create a new instance of the BasicAuthenticationEntryPoint
and configure it. This method is then called in the configure()
method of the security configuration to set the authentication entry point. ๐
๐ Resolving Multiple Bean Instances
Now, you might be wondering why calling entryPoint()
multiple times in the configuration doesn't result in multiple instances of the bean. Excellent observation! ๐ง
When you call entryPoint()
in the configuration, Spring doesn't instantiate a new bean instance. Instead, it recognizes that you are referring to the bean defined by the entryPoint()
method and retrieves the existing instance. This approach ensures that you always get the same bean instance across your application. ๐
๐ Behind the Scenes: CGLIB Proxying
Have you ever wondered how Spring achieves this clever "magic" of retrieving a single instance of a bean method? The answer lies in behind-the-scenes Spring proxying using CGLIB (Code Generation Library). ๐งช
When you annotate a method with @Bean, Spring generates a dynamic proxy class based on the annotated method. This dynamic proxy intercepts calls to the method and tracks the bean instances created. Subsequent invocations of the method return the previously created instance, thanks to the proxy's bookkeeping. ๐
๐ก Pro Tips: Best Practices
To leverage the power of @Bean annotated methods effectively, consider these best practices:
Define @Bean annotated methods in @Configuration classes or @Component classes so that Spring can discover them automatically.
Make sure @Bean methods are non-private and non-static. Spring cannot access private methods, and static methods lose the benefits of dynamic proxying.
Prioritize constructor injection over method injection wherever possible. Constructor injection ensures that dependencies are explicit and can be better managed by Spring.
๐ข Call-to-Action: Engage with Us! ๐
Are you using @Bean annotated methods in your Spring Java configuration? Have you encountered any challenges or learned any cool tips? Share your experiences and insights with us in the comments section below! Let's help each other master the art of Spring development. ๐ธ
Keep coding, and remember, with @Bean annotated methods, Spring's magic is at your fingertips! โจ๐
Stay tuned for more exciting tech insights and don't forget to subscribe to our newsletter to stay updated with the latest trends and solve more tech mysteries together! ๐
Happy coding! ๐๐ฉโ๐ป๐จโ๐ป