Bold words in a string of strings.xml in Android
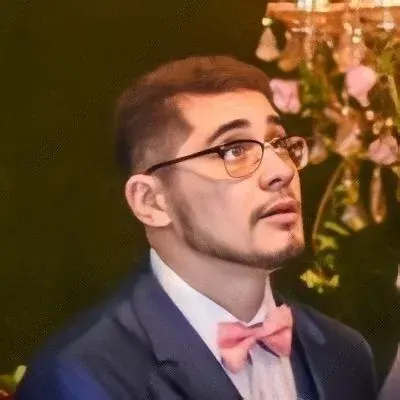
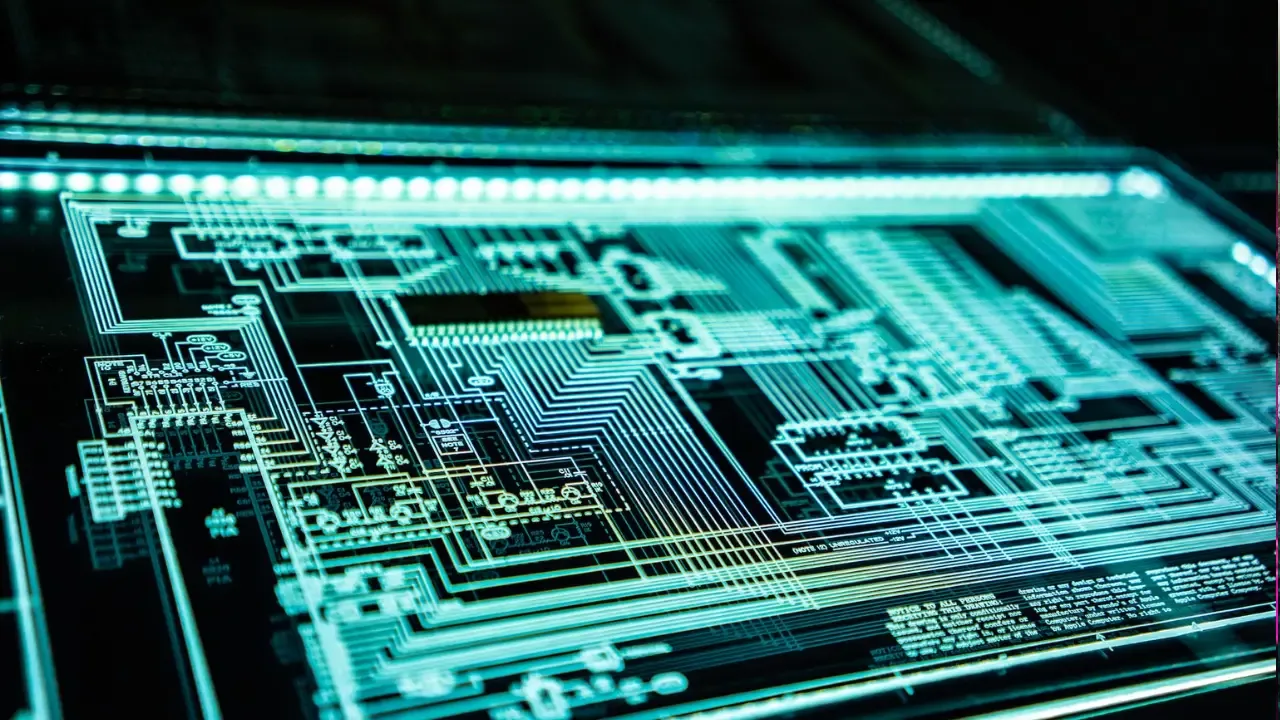
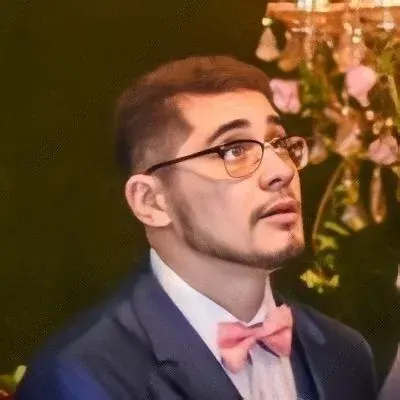
Making Bold Words in strings.xml in Android 📲
Having a long text in one of the strings of your Android project's strings.xml
file can be quite common. But what if you want to emphasize certain words in that text by making them bold and changing their color? In this blog post, we'll explore a simple and effective solution to achieve this desired effect.
The Problem 🤔
Let's say you have the following content in your strings.xml
file:
<string name="long_text">This is a <b>long</b> text where <b>some</b> words need to be <b>bold</b> and have a different color.</string>
As you can see, we have enclosed the words "long," "some," and "bold" with the <b>
tag. However, when you use this string in your app, you might not see the expected result. 🔍
The Solution ✅
To make the bold and color changes take effect in your app, you need to use the appropriate Html.fromHtml()
method when retrieving the string from strings.xml
. Here's an example:
TextView textView = findViewById(R.id.your_text_view);
String text = getResources().getString(R.string.long_text);
CharSequence formattedText = Html.fromHtml(text);
textView.setText(formattedText);
By using Html.fromHtml()
, the HTML tags within the string will be interpreted correctly, allowing you to display the bold and colored words as intended. 🎉
Adding Colors 🌈
If you also want to change the color of the bold words, you can incorporate CSS in HTML tags. Here's an example:
<string name="long_text">This is a <b><font color="#FF0000">long</font></b> text where <b><font color="#00FF00">some</font></b> words need to be <b><font color="#0000FF">bold</font></b> and have a different color.</string>
In this modified strings.xml
snippet, the <font>
tag with the color
attribute is used to specify the desired color using hexadecimal values. Feel free to customize the colors as per your preference. 💅
Engage with Us! 🌟
We hope this guide helped you tackle the challenge of bolding words and changing their color in strings.xml files in Android with ease. If you have any questions or face any other Android development-related problems, don't hesitate to reach out to us in the comments section below. Let's solve those coding puzzles together! 💪
Also, be sure to share this post with your fellow developers who might be struggling with the same issue. Spread the knowledge and help build a stronger and more colorful Android development community! 🌍📲💻
Now go forth and make your strings.xml bold and beautiful! Happy coding! 😄✨