Binding a list in @RequestParam
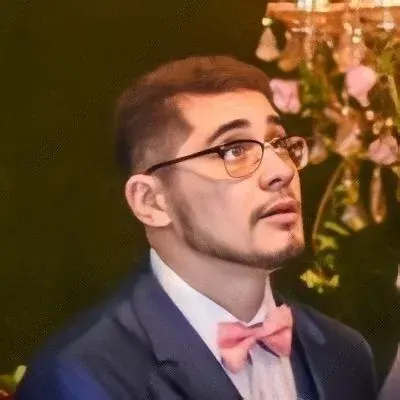
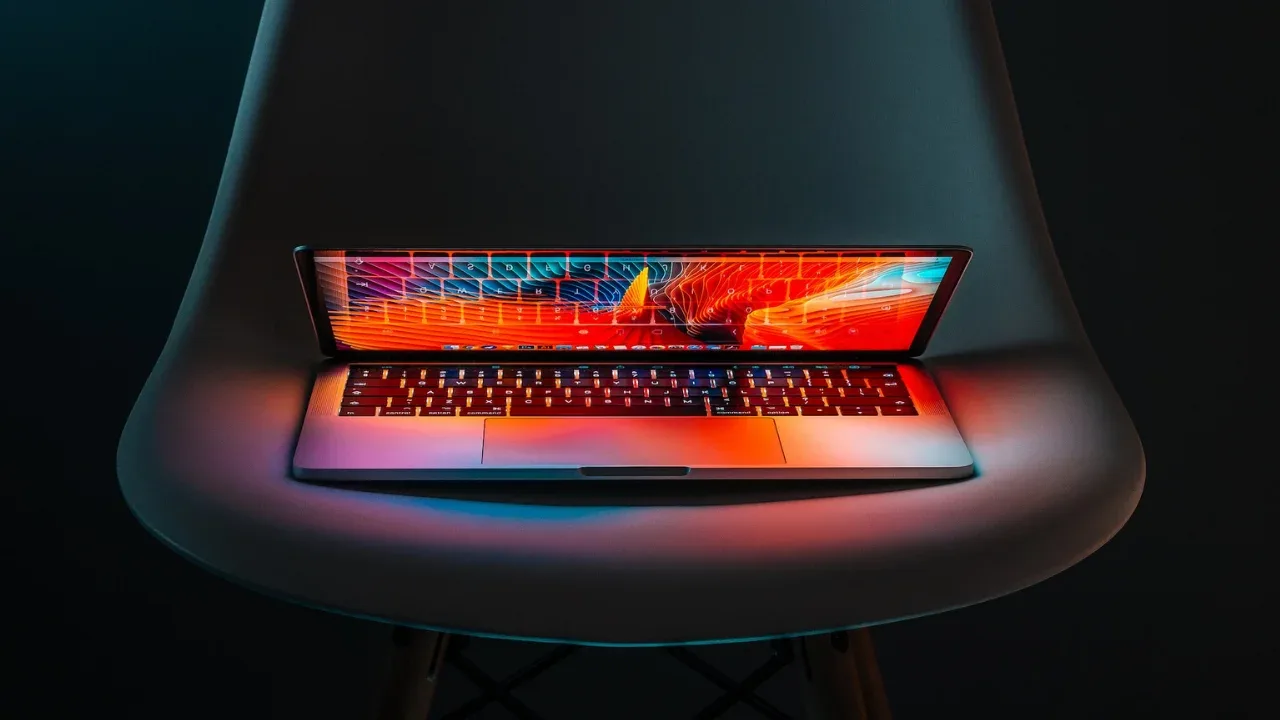
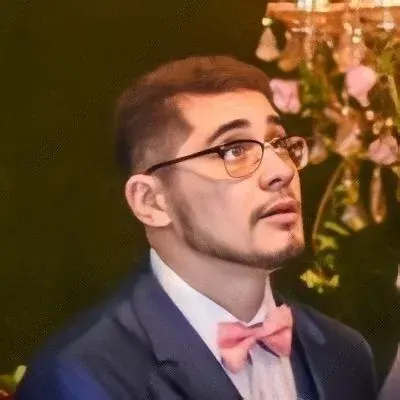
Binding a List in @RequestParam: A One-Stop Guide 📋🔀💡
Are you struggling with binding a list of parameters in your controller method using @RequestParam? We've got you covered! In this blog post, we'll address the common issues and provide you with easy solutions to bind those elusive parameters. 💪🤓
The Challenge 🤔
Let's start with the challenge at hand. You have a form that sends parameters in a specific format, such as:
myparam[0] : 'myValue1'
myparam[1] : 'myValue2'
myparam[2] : 'myValue3'
otherParam : 'otherValue'
anotherParam : 'anotherValue'
You want to bind the myParam[]
parameters to a list or array in your controller method, while ignoring the other parameters.
Attempting the Usual Solutions ⚙️❌
Naturally, you might think you can achieve this by using the following syntax:
public String controllerMethod(@RequestParam(value="myParam") List<String> myParams){
// ...
}
Or perhaps:
public String controllerMethod(@RequestParam(value="myParam") String[] myParams){
// ...
}
But, unfortunately, none of them seem to do the trick. Even when you add a value to the map, it fails to bind the params:
public String controllerMethod(@RequestParam(value="myParam") Map<String, String> params){
// ...
}
A Simple Solution ✅
Don't worry! We have a simple solution for you. Instead of relying solely on @RequestParam
, you can combine it with another annotation to achieve the desired binding. 🎉
Here's what you can do:
public String controllerMethod(@RequestParam Map<String, String> params, @RequestParam(value="myParam") List<String> myParams){
// ...
}
By including both @RequestParam Map<String, String> params
and @RequestParam(value="myParam") List<String> myParams
in your controller method, you can now bind the myParam[]
parameters to a list successfully.
A Better Alternative 🌟
If you find this solution a bit cumbersome and want a more elegant approach, you can create a simple class with a list attribute and annotate it with @ModelAttribute
. Let's take a look:
public class MyParams {
private List<String> myParams;
// getters and setters...
}
public String controllerMethod(@ModelAttribute MyParams myParams){
// ...
}
By introducing the MyParams
class and using @ModelAttribute
, you can bind the myParam[]
parameters to a list without cluttering your method signature.
Your Turn to Take Action! 💪📝
Now that you've discovered the solutions to your binding woes, it's time to apply them in your own code. Give our suggestions a try and let us know how they worked for you. If you have any further questions or need additional assistance, don't hesitate to reach out. We're here to help!
Remember, sharing is caring! If you found this blog post helpful, spread the word by sharing it with your fellow developers and tech enthusiasts. Let's empower each other to conquer those coding challenges together! 👥🚀
Stay tuned for more tech tips and tricks! Happy coding! 💻😄