Best way to compare 2 XML documents in Java
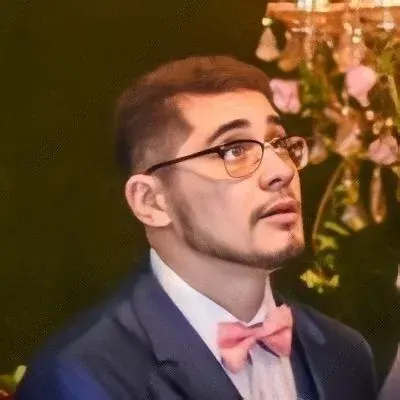
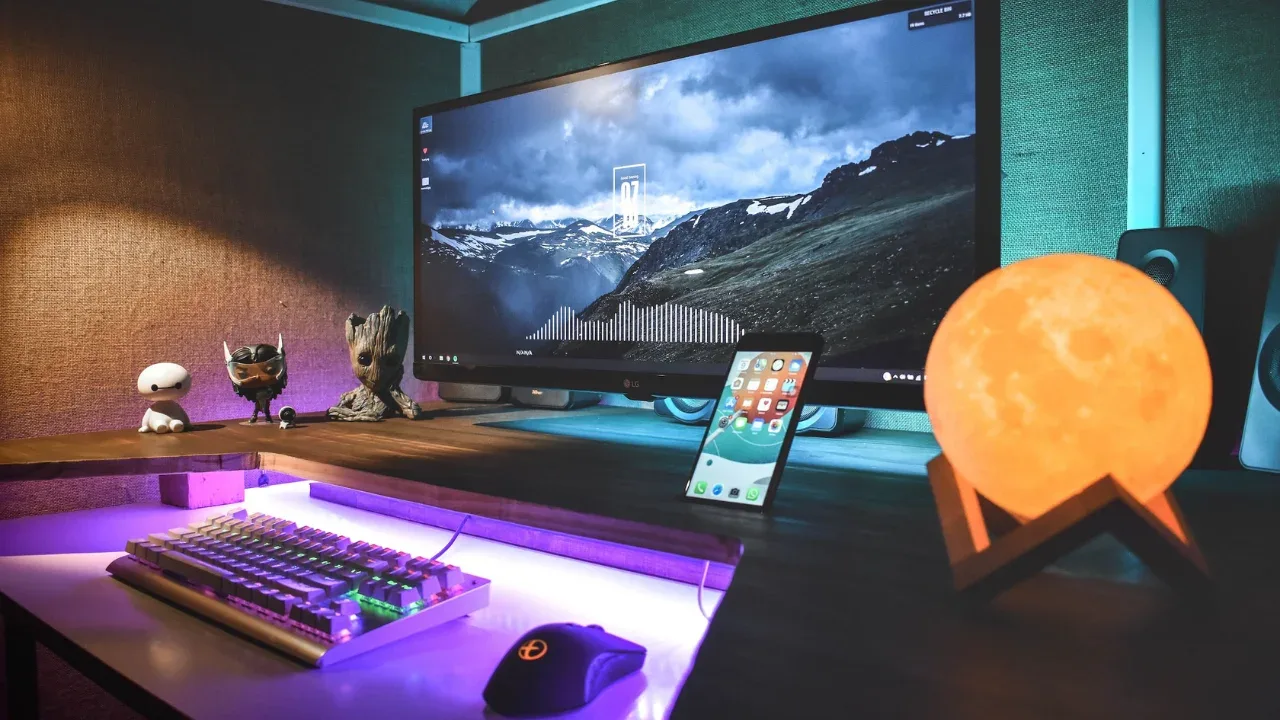
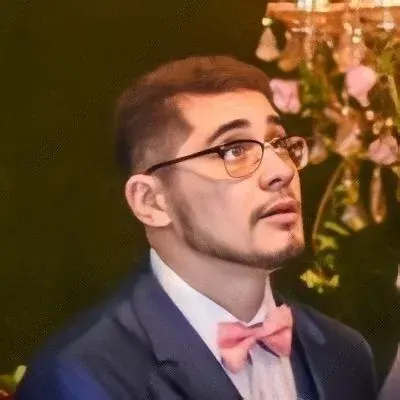
🖥️✨ Comparing XML Documents in Java Made Easy ✨🖥️
Are you struggling to compare two XML documents in Java? 😕 Don't worry, we've got you covered! In this blog post, we'll address the common issues you might encounter and provide you with easy and efficient solutions. 💪
🔎 The Problem
So, you have two Java Strings that contain valid XML, and you want to determine if they are semantically equivalent. Sounds simple, right? 🤔 Well, not quite. The challenge arises when the XML documents have inconsistent formatting or different XML namespaces. String comparisons won't cut it because they lack the flexibility to handle these variations.
🚀 The Solution
Fortunately, Java offers several libraries that can simplify the XML comparison process. One of the most popular and powerful libraries is XMLUnit. 🌟
1. Adding XMLUnit to Your Project
Before we dive into the code, let's make sure you've added XMLUnit to your project's dependencies. Add the following Maven dependency to your pom.xml
file:
<dependency>
<groupId>org.xmlunit</groupId>
<artifactId>xmlunit-core</artifactId>
<version>2.8.2</version>
</dependency>
2. Comparing XML Documents
To compare two XML documents using XMLUnit, follow these steps:
import org.xmlunit.builder.DiffBuilder;
import org.xmlunit.builder.Input;
import org.xmlunit.diff.Diff;
public class XMLComparator {
public static boolean compareXML(String xml1, String xml2) {
Diff diff = DiffBuilder.compare(Input.fromString(xml1))
.withTest(Input.fromString(xml2))
.build();
return !diff.hasDifferences();
}
}
In the example above, compareXML
method takes two XML Strings as input and returns true
if they are semantically equivalent, false
otherwise.
3. Determining Differences
If you want to go the extra mile and determine the specific differences between the XML documents, XMLUnit provides a convenient way to achieve that:
import org.xmlunit.diff.Difference;
import org.xmlunit.diff.DifferenceEvaluators;
public class XMLComparator {
public static List<Difference> getDifferences(String xml1, String xml2) {
Diff diff = DiffBuilder.compare(Input.fromString(xml1))
.withTest(Input.fromString(xml2))
.withDifferenceEvaluator(DifferenceEvaluators.Default)
.build();
return diff.getDifferences();
}
}
The getDifferences
method will return a list of Difference
objects, each representing a specific difference between the XML documents.