@Autowired and static method
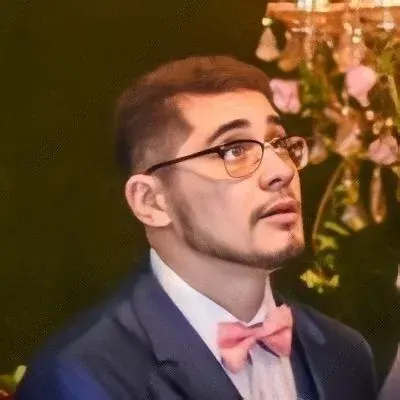
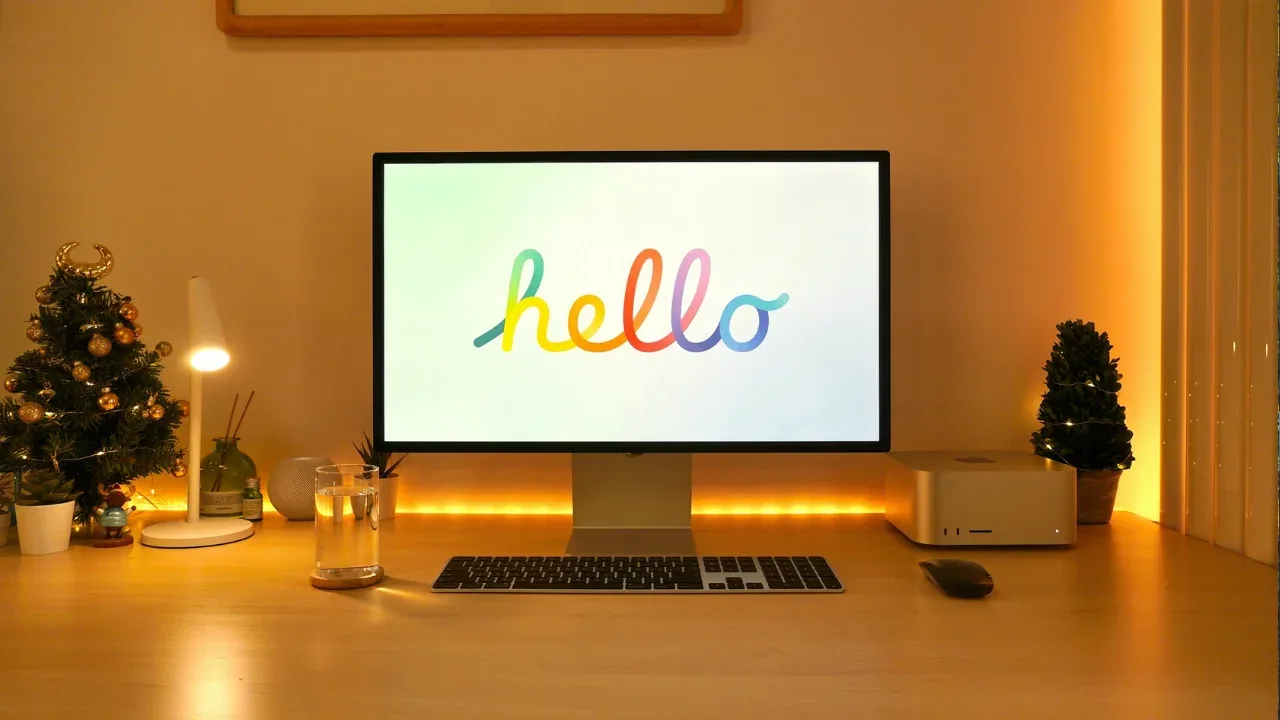
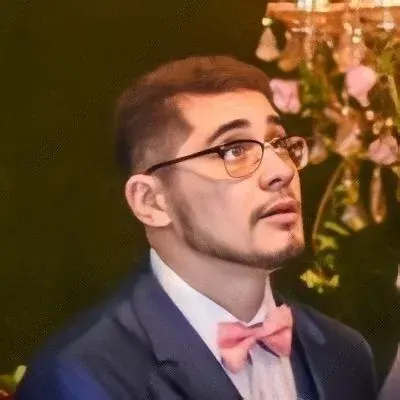
Blog Post: Using @Autowired in a Static Method: Finding a Simple Solution
š Hey there, tech enthusiasts! Are you facing the challenge of using the @Autowired
annotation within a static method? š¤ Don't worry, you're not alone! We've got your back with a simple hack to overcome this obstacle.
Imagine a scenario where you have a service, Foo
, that needs to be autowired within a static method of another class, Boo
. š” However, the current design doesn't allow you to change randomMethod()
to be non-static. So, how do you ensure that you can still use the autowired Foo
bean? Let's dive into the solution!
Understanding the Problem
Before we jump into the solution, let's quickly understand why using @Autowired
in a static method is a no-go š«.
Unlike non-static methods, static methods do not have access to instance variables, including autowired beans. This limitation arises because static methods belong to the class rather than an instance of the class.
The Simple Hack: ApplicationContext
To workaround this limitation, we can use the ApplicationContext
to manually fetch the autowired bean within the static method. š
First, we need to ensure that the
ApplicationContext
is accessible throughout our application. One approach is to make it a static field in a singleton class:public class ApplicationContextProvider implements ApplicationContextAware { private static ApplicationContext context; public static ApplicationContext getApplicationContext() { return context; } @Override public void setApplicationContext(ApplicationContext applicationContext) throws BeansException { context = applicationContext; } }
Next, we modify our
Foo
class to implement theInitializingBean
interface. This allows us to fetch the autowired bean and store it in a static variable using theApplicationContext
:@Service public class Foo implements InitializingBean { private static Foo fooInstance; @Override public void afterPropertiesSet() { fooInstance = ApplicationContextProvider.getApplicationContext().getBean(Foo.class); } public int doStuff() { return 1; } public static Foo getFooInstance() { return fooInstance; } }
Finally, in the
randomMethod()
of theBoo
class, we fetch the autowiredFoo
bean using the static method we added inFoo
:public class Boo { public static void randomMethod() { Foo.getFooInstance().doStuff(); } }
And there you have it! š By utilizing the ApplicationContext
and a simple hack, we were able to access the autowired Foo
bean within a static method.
When to Use this Approach
Although this hack allows you to circumvent the limitations of using @Autowired
in a static method, it is important to note that it should be used as a last resort.
When faced with scenarios where you cannot change the design to make the method non-static, or using a @Bean
method in a configuration class is not feasible, the ApplicationContext
hack is a viable solution. However, it's always recommended to refactor and design your codebase to follow best practices whenever possible. š
Engage with Us!
We hope this simple hack has helped you overcome the challenge of using @Autowired
in a static method. If you have any questions, suggestions, or alternative approaches, we would love to hear from you! Leave a comment below and join the discussion. Let's learn and grow together! š
Happy coding! š»āØ