Any way to declare an array in-line?
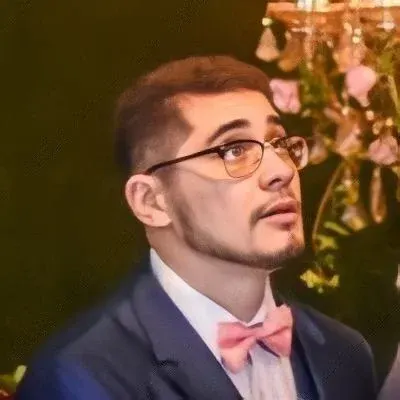

πDeclare an Array In-Line: The Ultimate Guide!π
Are you tired of declaring a separate variable just to pass an array to a method? π Do you wish there was a more concise way to declare an array in-line? π€ You're in luck! In this guide, we'll explore the different ways to declare an array in-line, saving you time and lines of code! πͺ
The Problem: Declaration Clutter π
Let's start by illustrating the problem you're facing. Imagine you have a method m()
that takes an array of Strings as an argument. You currently have to declare a separate array variable and then pass it to the method like this:
String[] strs = {"blah", "hey", "yo"};
m(strs);
But what if you don't want to clutter your code with unnecessary variables and want a one-liner solution? π±
The Solution: Inline Array Declaration π
Good news! Java provides a compact way to declare an array in-line while making the method call. Here's how you can achieve it:
m(new String[]{"blah", "hey", "yo"});
By using the new
keyword and specifying the array type within square brackets []
, you can directly create and pass the array to the method in one line! π
Example Usage π±
To help solidify your understanding, let's walk through an example:
class ArrayExample {
public static void main(String[] args) {
printArray(new int[]{1, 2, 3, 4, 5});
printArray(new String[]{"apple", "banana", "orange"});
}
static void printArray(int[] arr) {
for (int num : arr) {
System.out.print(num + " ");
}
System.out.println();
}
static void printArray(String[] arr) {
for (String str : arr) {
System.out.print(str + " ");
}
System.out.println();
}
}
In this example, we have two methods: printArray
for printing integer and string arrays. Instead of declaring separate variables for each array, we directly pass them in-line when making the method calls! π
When to Use Inline Array Declaration ποΈ
While the ability to declare an array in-line can be handy, it's important to use it wisely. Here are a few scenarios where it comes in handy:
When passing small, simple arrays to a method.
When you don't need to reuse the array later in your code.
When you want to remove clutter and keep your code concise.
However, if you have complex array initialization logic or need to manipulate the array further, declaring it separately might be a better approach. π§
Get Ready to Simplify Your Code! π₯
Now that you know how to declare an array in-line, you can kiss goodbye to clutter and unnecessary variable declarations! π Spice up your code with this one-liner and make it more concise, readable, and enjoyable! π
Give it a try in your next project and share your experience with us! We love hearing from fellow developers! ππ¬
Do you have any more questions about array declaration or any other Java topic? Let us know in the comments below, and we'll be happy to help! π
Happy coding! π»β¨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
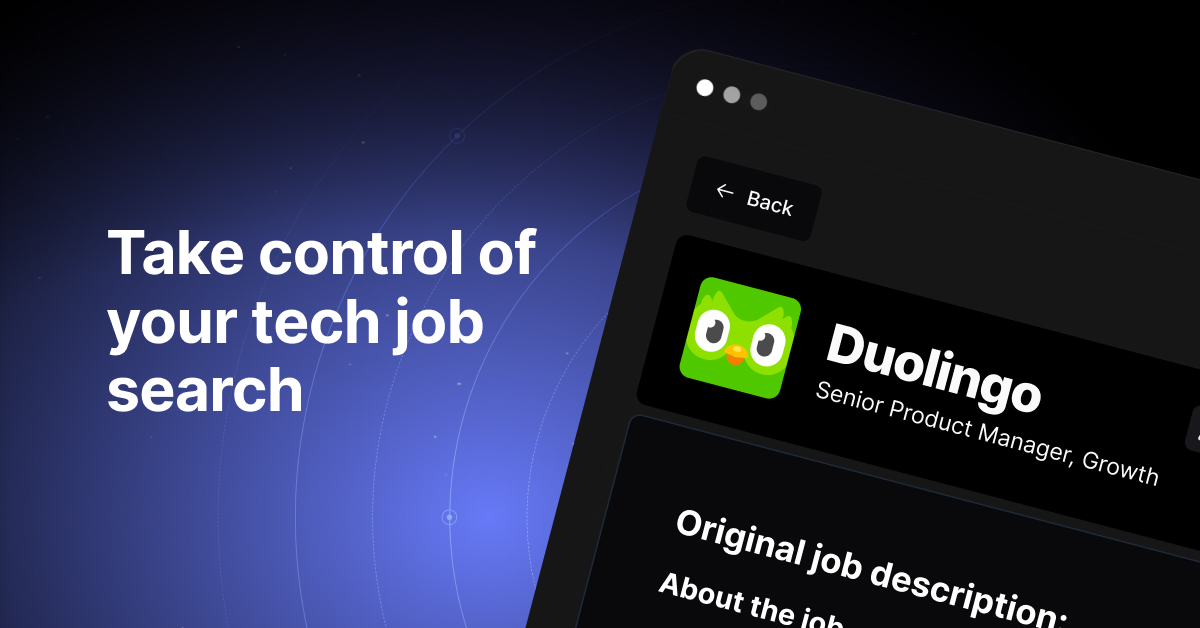