Android getting value from selected radiobutton
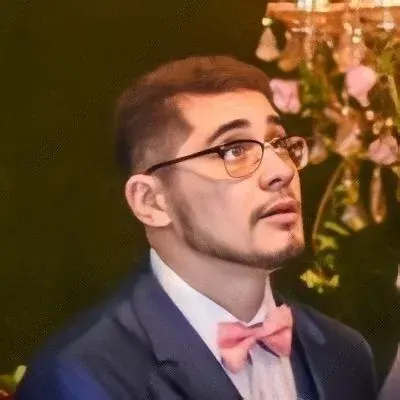
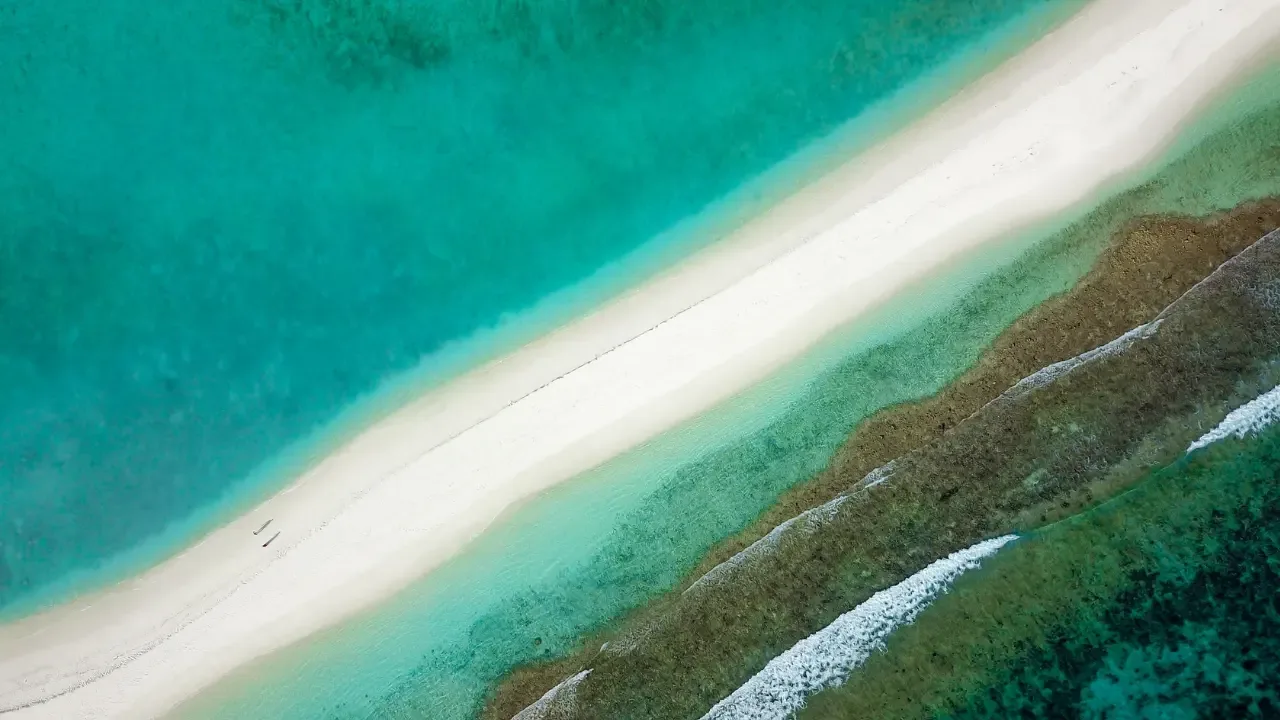
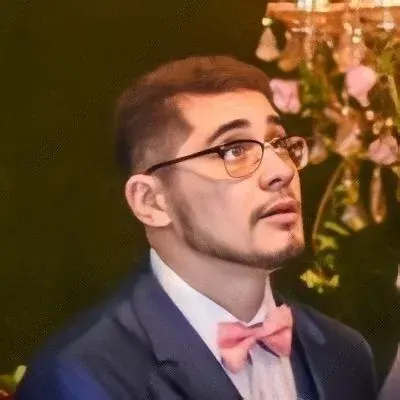
📱 Getting Value from Selected RadioButton in Android
Are you trying to get the value of a selected RadioButton in your Android app but struggling with implementing the correct code? Don't worry, we've got you covered! In this guide, we will walk you through the common issues and provide easy solutions to help you display the selected RadioButton value in a Toast message. 🥳
The Problem
The code snippet you provided shows that you have a RadioGroup with three RadioButtons, and you want to show the value of the selected RadioButton in a Toast message when the selection changes. However, the value displayed in the Toast message doesn't update correctly.
The Solution
To get the correct value of the selected RadioButton, you need to update the value variable inside the onCheckedChanged listener. Here's the updated code with the necessary changes:
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.widget.RadioButton;
import android.widget.RadioGroup;
import android.widget.Toast;
public class MainActivity extends Activity {
RadioGroup rg;
RadioButton selectedRadioButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
rg = findViewById(R.id.radioGroup1);
rg.setOnCheckedChangeListener(new RadioGroup.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(RadioGroup group, int checkedId) {
selectedRadioButton = findViewById(checkedId);
if (selectedRadioButton != null) {
String value = selectedRadioButton.getText().toString();
Toast.makeText(MainActivity.this, value, Toast.LENGTH_SHORT).show();
}
}
});
}
}
Here's what changed:
We removed the duplicate declaration of the
rg
variable inside theonCreate
method.We added a
RadioButton
variable,selectedRadioButton
, to store the currently selected RadioButton.Inside the
onCheckedChanged
listener, we updated theselectedRadioButton
variable with the currently selected RadioButton.We checked if
selectedRadioButton
is not null before retrieving its text value.
Now, when you change the selection in the RadioGroup, the Toast message will display the correct value of the selected RadioButton.
How to Implement
To implement this solution in your own Android app, follow these steps:
Open your project in Android Studio.
Open the
MainActivity.java
file where you want to implement the code.Replace the existing code with the updated code provided above.
Update the layout XML files if necessary to match the IDs used in the Java code.
Build and run your app to test the changes.
Now you have a working solution to get the value from the selected RadioButton in your Android app!
Let's Connect!
If you found this guide helpful, leave a comment below and let us know your thoughts. We would love to hear about your experiences and any other Android-related topics you would like us to cover. Happy coding! 🚀