android.content.res.Resources$NotFoundException: String resource ID #0x0
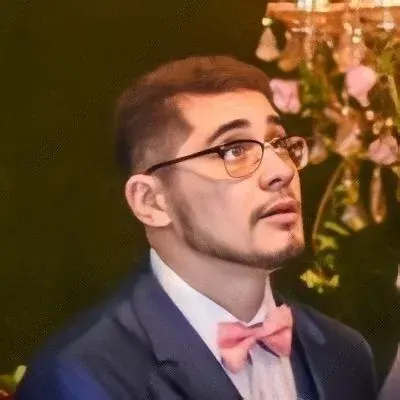
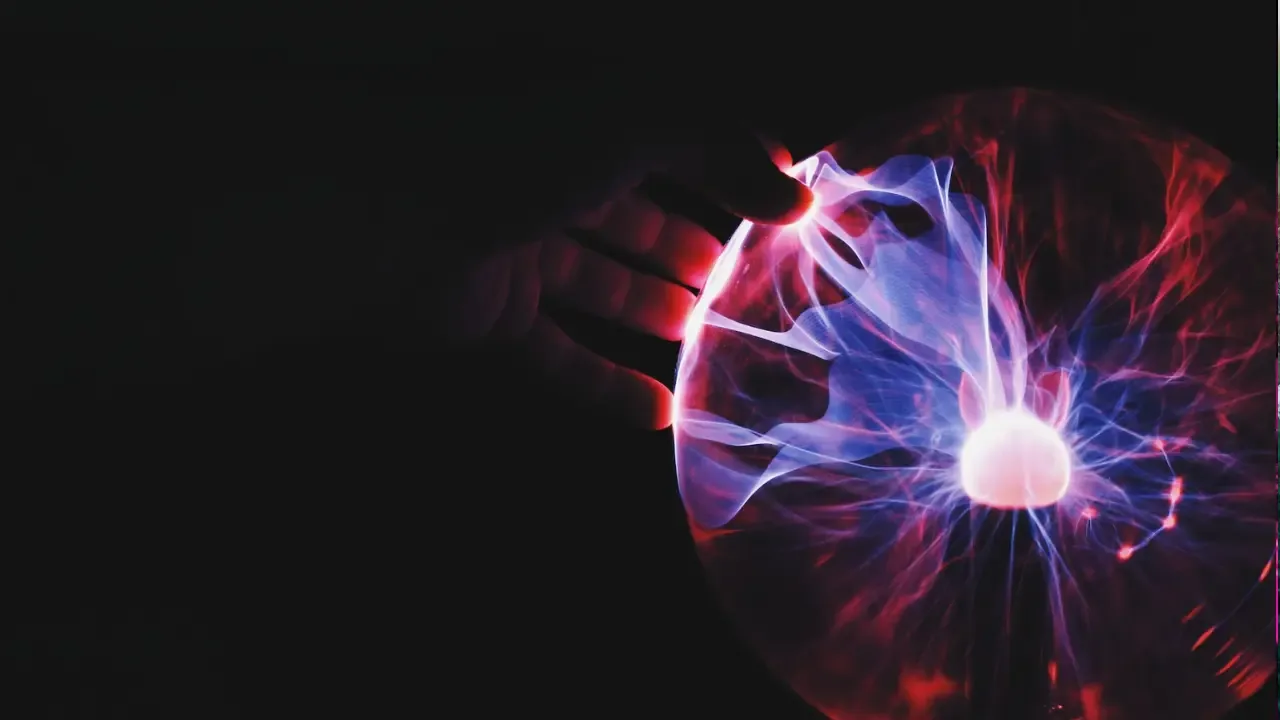
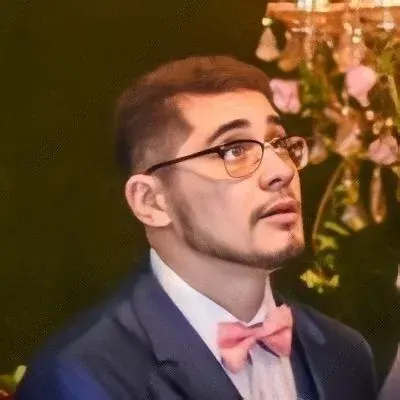
How to Fix the android.content.res.Resources$NotFoundException Error
If you are developing an Android app and you encounter the dreaded android.content.res.Resources$NotFoundException
error, don't panic! This error commonly occurs when you're trying to access a resource (such as a string or a layout) that cannot be found. Fortunately, there are easy solutions to this problem. In this blog post, we will guide you through the steps to fix this error and get your app up and running smoothly. 💪
Understanding the Problem
Before we jump into the solutions, let's understand why this error occurs. The error message String resource ID #0x0
suggests that the resource ID being referenced is not valid. In the provided code snippet, the error occurs in the ApplicationAdapter.getView()
method when trying to set the text of a TextView
using textView.setText(app.getTitle())
.
Solution 1: Check Resource IDs
The most common cause of this error is referencing a resource that doesn't exist. To fix this, double-check the resource IDs in your XML layout file and make sure they match the IDs in your Java code. In the provided code snippet, ensure that the resource IDs wardNumber
, name
, and dateTime
in the XML layout file (app_custom_list.xml
) match the IDs used in the getView()
method.
Here's an example of a correct XML layout:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/wardNumber"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="3dp"
android:text="Ward Number"
android:textSize="22dp" />
<!-- Add the rest of your layout components here -->
</RelativeLayout>
Solution 2: Check Context and Resources
Another possible cause of this error is an issue with the Context
or Resources
object being used. Make sure you have passed the correct Context
object to your ApplicationAdapter
. In the provided code snippet, ensure that the Context
object passed in the constructor is valid and not null.
Here's an example of the correct usage of the ApplicationAdapter
:
public ApplicationAdapter(Context context, List<Application> items) {
super(context, R.layout.app_custom_list, items);
this.items = items;
}
Solution 3: Check Resource Availability
If the above solutions didn't solve the issue, it's possible that the resource you are trying to access is not available in the current context. Check if the resource (e.g., drawable, string) is part of the app's resources and properly defined. Ensure that you have added the necessary resources in your project's resource folders (e.g., res/drawable
, res/values/strings.xml
).
For example, if you are trying to set an image drawable dynamically, make sure the drawable resource exists with the correct name and in the appropriate folder. You can verify this by checking the R.drawable
class to see if the resource matching your name is present.
Conclusion
The android.content.res.Resources$NotFoundException
error can be frustrating, but by following the solutions mentioned above, you should be able to resolve the issue. Remember to double-check your resource IDs, ensure the Context
and Resources
objects are valid, and verify the availability of the resources you are trying to access.
If you continue to experience the error or have any other questions or suggestions, feel free to leave a comment below. Happy coding! 💻🚀